Question
My code will only use multiplication even if the user prompts the program to use a different arithmetic. How can i fix that? Also, my
My code will only use multiplication even if the user prompts the program to use a different arithmetic. How can i fix that?
Also, my percentage variable in the generateProblem function is not working, if i get a single problem wrong, it resets the difficulty. it should let me continue if i score a 75% or higher.
//Gage Pelon //Project 1
#include
//Prototypes bool multiplication(int num1, int num2); bool addition(int num1, int num2); bool subtraction(int num1, int num2); bool division(int num1, int num2); int menu(); int reset(int currentLevel); void right(); void wrong(); bool generateProblem(int level);
//Function to compute multiplication bool multiplication(int num1, int num2) { int useranswer; cout << num1 << " * " << num2 << " = "; cin >> useranswer; if (useranswer == (num1*num2)) return true; else return false; } //Function to compute addition bool addition(int num1, int num2) { int useranswer; cout << num1 << " + " << num2 << " = "; cin >> useranswer; if (useranswer == (num1+num2)) return true; else return false; } //Function to compute subtraction bool subtraction(int num1, int num2) { int useranswer; cout << num1 << " - " << num2 << " = "; cin >> useranswer; if (useranswer == (num1-num2)) return true; else return false; } //Function to compute division bool division(int num1, int num2) { int useranswer; cout << num1 << " / " << num2 << " = "; cin >> useranswer; if (useranswer == (num1/num2)) return true; else return false; } //Function to choose difficulty and arithmetic type int menu() { int arithmetic, i, lvl; cout << "Welcome to CAI" << endl << endl; cout << "Please choose one of the following: " << endl; cout << " 1. Addition 2. Subtraction 3. Multiplication 4. Division 5. Random "; cin >> arithmetic;
cout << "Enter the level of difficulty that you would like to start at: "; cin >> lvl; generateProblem(lvl); cout << endl;
switch (arithmetic) { case 1: bool addition(int num1, int num2); break; case 2: bool subtraction(int num1, int num2); break; case 3: bool multiplication(int num1, int num2); break; case 4: bool division(int num1, int num2); break; case 5: i = rand() % 4; if (i == 0) { bool addition(int num1, int num2); break; } else if (i == 1) { bool subtraction(int num1, int num2); break; } else if (i == 2) { bool multiplication(int num1, int num2); break; } else if (i == 3) { bool division(int num1, int num2); break; } break;
}
return 1; } // Resets current level to 1 if student does not pass that level. int reset(int currentLevel) { currentLevel = 1; return currentLevel; } //Function for right answers void right() { int r; r = rand() % 4; cout << endl; switch (r) { case 0: cout << "Very good! "; break; case 1: cout << "Excellent! "; break; case 2: cout << "Nice work! "; break; case 3: cout << "Keep up the good work! "; break; } cout << endl; } //Function for wrong answers void wrong() { int w; w = rand() % 4; cout << endl; switch (w) { case 0: cout << "No. Please try again. "; break; case 1: cout << "Wrong. Try once more. "; break; case 2: cout << "Don't give up! "; break; case 3: cout << "No. Keep trying. "; break; } cout << endl; } //Generates problem bool generateProblem(int level) { srand(time_t(0)); int MAXIMUM_TRIES = 10; int qustionCount = 1; double percentage; int correct = 0; bool success = false;
int min = (int)pow(10.0, level - 1); int max = (int)(pow(10.0, level) - min + 1); //Generate two random numbers based on level //Level -Random numbers in between 1 to 9 //Leve2 -Random numbers in between 10 to 99 //Leve3 -Random numbers in between 100 to 999 //Leve4 -Random numbers in between 1000 to 9999 //and so on... int num1 = rand() % max + min; int num2 = rand() % max + min; cout << "Level " << level << endl << endl;
while (qustionCount <= MAXIMUM_TRIES) { if (multiplication(num1, num2)) { right(); //Increment the question count qustionCount++; //increment the correct answer count correct++; num1 = rand() % (max - min) + min; num2 = rand() % (max - min) + min; } else { wrong(); //Increment the question count qustionCount++; } } //calculate the percentage of correct answers percentage = (correct / MAXIMUM_TRIES) * 100;
if (percentage >= 75) { cout << "Congratulations, you are ready for the next level! " << endl; success = true; } else { cout << "Please ask your teacher for extra help. " << endl; success = false; } return success; } //Main function int main() { int LEVEL, currentLevel; LEVEL = 1; currentLevel = LEVEL;
menu(); //The while loop continues playing the game for different levels while (true) { //generate two random numbers based on currentLevel bool success = generateProblem(currentLevel);
if (success) { //Increment the LEVEL by one LEVEL++; //set currentLevel to LEVEL currentLevel = LEVEL; } else { currentLevel = reset(currentLevel); cout << "Next Student " << endl; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
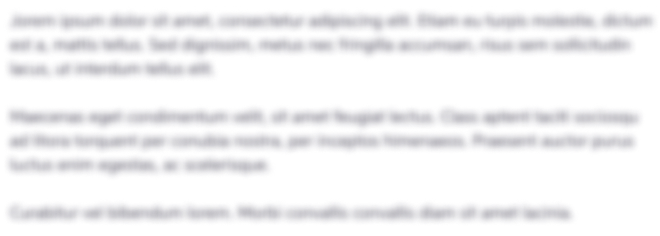
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started