Question
Need further help with this Java code for Computer science: The Orginal code is down below that needs modifying. Add a debugging bit. When this
Need further help with this Java code for Computer science: The Orginal code is down below that needs modifying.
Add a debugging bit. When this bit is set, the processor that we are simulating will be in debug mode. When in debug mode, our simulator should output the contents of the AC after every instruction.
LDI x <-- load the AC with the value x LDM y <-- load the AC with the value in memory location y ST y <-- store the value in the AC in memory location y
(If necessary, feel free to pass memory[] as an additional argument to the execute function or make memory[] a static class variable.) Change the code from your previous code to implement fixed length instructions: All instructions are now 2 ints in length (one int for the opcode and one for the operand). For example,
int m2[] = { 9, -5, CLR, 0, ADDI, 17, ADDI, 2, ST, 0, ADDM, 0, ADDM, 1, CLR, 0, HALT, 0 }; interpret( m2, 2 );
Answer the following questions.
Question A: What is the value of the PC and AC after each of the above instructions executes?
Question B: Consider all of the instructions that your machine executes. Which instructions are superfluous (i.e., can be implemented by a sequence of one or more of the other instructions)?
CODE:
public class Interpreter {
static int PC; //program counter holds address of next instr
static int AC; //the accumulator - a register for doing arithmetic
static boolean run_bit = true; //a bit that can be turned off to halt the machine
static int instr; //a holding register for the current instruction
static int instr_type; //the instruction type (opcode)
static int data_loc; //the address of the data, or -1 if none
static int data; //holds the current operand
static int CLR = 5;
static int ADDI = 10;
static int ADDM = 15;
static int HALT = 20;
//----------------------------------------------------------------------
private static int get_instr_type ( int opcode ) {
return opcode;
}
//----------------------------------------------------------------------
private static int find_data ( int opcode, int type, int memory[] ) {
type = PC;
if (opcode == ADDM) {
return memory[type];
}
if (opcode == ADDI) {
return type;
} else
return -1;
}
//----------------------------------------------------------------------
private static void execute ( int type, int data ) {
while (type == HALT) {
run_bit = false; //halts the processor
break;
}
while (type == ADDI) {
AC = AC + data; //adds the value x to AC
System.out.println(AC);
break;
}
while (type == CLR) {
AC = 0; //sets AC to zero
System.out.println(AC); //print out data
break;
}
while (type == ADDM) {
AC = AC + data; //add the value in memory location y to AC
System.out.println(AC);
break;
}
}
//------------------------------------------------------------------
//This procedure interprets programs for a simple machine. The machine
//has a register AC (accumulator), used for arithmetic. The interpreter
//keeps running until the run bit is turned off by the HALT instruction.
//The state of a process running on this machine consists of the memory,
//the program counter, the run bit, and the AC. The input parameters
//consist of the memory image and the starting address.
public static void interpret ( int memory[], int starting_address ) {
PC = starting_address;
run_bit = true;
while (run_bit) {
instr = memory[PC]; //fetch next instruction into instr
PC = PC + 1; //increment program counter
instr_type = get_instr_type( instr ); //determine instruction type
data_loc = find_data( instr, instr_type, memory ); //locate data (-1 if none)
if (data_loc >= 0) { //if data_loc is -1, there is no operand
data = memory[data_loc]; //fetch the data
}
execute( instr_type, data ); //execute instruction
}
}
//tests the program
public static void main(String[] args) {
int m2[] = { 2, -5, 15, CLR,
ADDI, 12, ADDI, 7, ADDM, 0, ADDM, 1, CLR, HALT };
System.out.println("First set: "); interpret(m2, 3);
int m3[] = { 4, 66, 22, 55, 13, CLR,
ADDI, 9, ADDM, 1, ADDI, 66, ADDM, 8, ADDI, 77, ADDM, 5, CLR, HALT };
System.out.println("Second set: "); interpret(m3, 3);
int m4[] = { 64, 78, 2, 99, 65, CLR,
ADDI, 7, ADDM, 0, ADDM, 3, ADDI, 79, CLR, ADDM, 2, ADDM, 6, CLR, HALT };
System.out.println("Third set: "); interpret(m4, 4);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
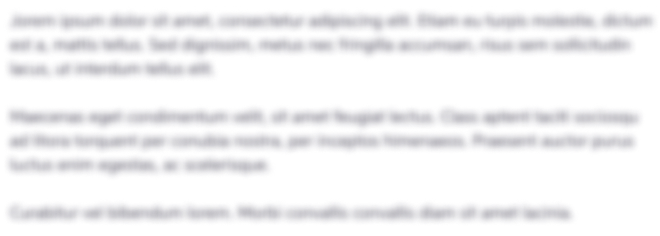
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started