Question
Need to add the code to convert infix expression to postfix expressions using stacks. After reading the expressions from the file, convert them to postfix
Need to add the code to convert infix expression to postfix expressions using stacks. After reading the expressions from the file, convert them to postfix form and evaluate the result. Generate the resulting infix and postfix conversion to the output file 'out.txt'. I am adding sample programs to output to file, but need to convert infix expressions to postfix after reading from file and outputing to output file. Also, evaluate the result and generate output to file. Also, in Step1.java (Line 59), Add the code to print the Array List st to the file which is giving me certain errors.
p4in.txt: (Input File):
2 + 3 ( 2 + 3) * 4 2 * 3 / (4 - 5) 2 / 3 + (4 - 5) 2 / 3 + 4 - 5 2 ^ 3 ^ 4 (2 ^ 3) ^ 4 2 * (3 / 4 + 5) (2 + 3) / (4 - 5) 2 / (3 - 4) * 5 2 - (3 / (4 - 5) * 6 + 0) ^ 1 (2 - 3 * 4) / (5 * 6 ^ 0) * 1 + 8
out.txt: Sample
Valid Expression: 9.5 - 4 * 3 ^ 2 / 6 + 8 * 4 Operator String: -*^/+*
Symbol Table: A 9.5 B 4.0 C 3.0 D 2.0 E 6.0 F 8.0 G 4.0
Variable String: ABCDEFG Infix of the expression: A-B*C^D/E+F*G
Input Infix Postfix Result
2 + 3 a+b ab+ 5
( 2 + 3) * 4 (a+b)*c ab+c* 20
2 * 3 / (4 - 5) a*b/(c-d) ab*cd-/ -6
2 / 3 + (4 - 5) a/b+(c-d) ab/cd-+ -0.33
2 / 3 + 4 - 5 a/b+c-d ab/c+d- -0.33
2 ^ 3 ^ 4 a^b^c abc^^ 2417851639229258300000000
(2 ^ 3) ^ 4 (a^b)^c ab^c^ 4096
2 * (3 / 4 + 5) a*(b/c+d) abc/d+* 11.5
(2 + 3) / (4 - 5) (a+b)/(c-d) ab+cd-/ -5
2 / (3 - 4) * 5 a/(b-c)*d abc-/d* -10
2 - (3 / (4 - 5) * 6 + 0) ^ 1 a-(b/(c-d)*e+f)^g abcd-/e*f+g^- 20
(2 - 3 * 4) / (5 * 6 ^ 0) * 1 + 8 (a-b*c)/(d*e^f)*g+h abc*-def^*/g*h+ 6
SymbolTable.java:
import java.util.ArrayList; import java.util.Scanner;
public class SymbolTable {
// Instance variables String strOperator; // Contains only the operators used in // expression String strVariable; // Contains only the variables (which take the // place of operands when symbol table is // complete ArrayList
/** * Constructor */ public SymbolTable(String expression) { // Scan the expression for invalid characters String expr = scan(expression);
if (!expr.equals("")) { // If expression contains valid characters then // build the symbol table this.table = new ArrayList
/** * Accepts the expression string and returns the valid expression which is * without spaces. Returns empty string is the expression contains invalid * character * * @param expr * - Expression to scan */ private String scan(String expression) { String operators = "\\^|\\*|/|\\+|-";
// Replace multiple consecutive spaces by a single space expression = expression.trim().replaceAll("\\s+", " ");
// Scanner to scan the expression Scanner line = new Scanner(expression);
while (line.hasNext()) { if (!line.hasNextDouble() && !line.hasNext(operators)) { // Close scanner line.close(); return ""; } line.next(); }
// Close scanner line.close();
return expression.replaceAll("\\s+", ""); }
/** * This method constructs the symbol table from the valid expression */ private void buildTable(String expression) { String allowedOperators = "^*+/-"; String operand = ""; for (char ch : expression.toCharArray()) { if (!operand.equals("") && allowedOperators.contains(ch + "")) { int size = this.table.size(); char symbol = (char)('A' + size); Variable var = new Variable(symbol, Double.parseDouble(operand)); // Add variable to the table this.table.add(var); // Add symbol to strVariable this.strVariable += symbol; // Add operator to strOperator this.strOperator += ch; operand = ""; } else { operand += ch; } } // Check for the last operand if (!operand.equals("")) { int size = this.table.size(); char symbol = (char)('A' + size); Variable var = new Variable(symbol, Double.parseDouble(operand)); // Add variable to the table this.table.add(var); System.out.print("\t"); // Add symbol to strVariable this.strVariable += symbol; } }
/** * This method returns the infix from strVariable and strOperator. * * @return */ public String buildInfix() { if (this.table.size() != 0) { StringBuffer infix = new StringBuffer(); for (int i = 0; i < this.strVariable.length(); i++) { // Append variable infix.append(this.strVariable.charAt(i)); // Append operator if (i < this.strOperator.length()) infix.append(this.strOperator.charAt(i)); } return infix.toString(); } return "Cannot build infix. Please check the expression."; }
/** * Prints the contents of the table */ @Override public String toString() { if (this.table.size() != 0) { StringBuffer sb = new StringBuffer(); for (Variable variable : this.table) { sb.append(variable + " "); } return sb.toString(); } return "Symbol table not generated. Please check the expression."; } }
Step1.java:
import java.io.BufferedWriter; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.OutputStreamWriter; import java.io.UnsupportedEncodingException; import java.io.Writer;
public class Step1 { public static void main(String[] args) { // Expression string String expression = "9.5 - 4 * 3 ^ 2 / 6 + 8 * 4";
// Create SymbolTable object SymbolTable st = new SymbolTable(expression); // Printing the required information in Console // Display my Name System.out.println("
writer.write("Variable String: " + st.strVariable); ((BufferedWriter) writer).newLine(); writer.write("Infix of the expression: " + st.buildInfix()); } catch (UnsupportedEncodingException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
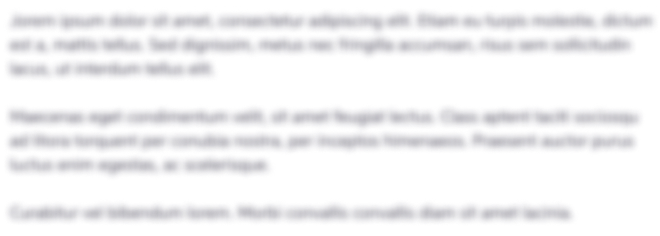
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started