Question
Part 1 (class GradeExams) and Part 2 (class GradeexamsUI) GradeExam: public final class GradeExams { /** * prevent instantiating this class */ private GradeExams() {}
Part 1 (class GradeExams) and Part 2 (class GradeexamsUI)
GradeExam:
public final class GradeExams
{
/**
* prevent instantiating this class
*/
private GradeExams() {}
/**
* Test driver
*
* @param args -unused-
*/
public static void main( String[] args )
{
char[] answerKey = "DBDCCDAEAD".toCharArray() ; // the correct answers
char[][] studentsAnswers = { // each student's answers
"ABACCDEEAD".toCharArray(),
"DBABCAEEAD".toCharArray(),
"EDDACBEEAD".toCharArray(),
"CBAEDCEEAD".toCharArray(),
"ABDCCDEEAD".toCharArray(),
"BBECCDEEAD".toCharArray(),
"BBACCDEEAD".toCharArray(),
"EBECCDEEAD".toCharArray(),
"EBECCDEEADX".toCharArray(),
"EBECCDEEA".toCharArray()
} ;
// descriptive introductory text
displayIntro() ;
// grade the exams: display the results on a per-student basis and summary statistics
gradeAllExams( answerKey, studentsAnswers );
}
/**
* Utility method to display identifying information and a description of what it does
*/
static void displayIntro()
{
System.out.println( "Comp 1050, Computer Science II" ) ;
}
/**
* Utility method to grade all exams and display summary statistics
*
* @param answerKey the set of correct answers
* @param allStudentsAnswers the answers for all of the students
*/
static void gradeAllExams( char[] answerKey,
char[][] allStudentsAnswers
)
{
// TODO - STUB - implement this method
}
/**
* Utility method to grade a single exam
*
* @param studentId the student's identification number
* @param answerKey the set of correct answers
* @param studentAnswers the student's answers
* @param correctAnswerCount counters for each question to track number of times answered
* correctly
* @return true indicates that we were able to grade the exam; false means the number of
* questions according to the answer key does not match the number of questions answered
* by the student - does not reflect whether the student 'passed'
*/
static boolean gradeAnExam( int studentId,
char[] answerKey,
char[] studentAnswers,
int[] correctAnswerCount
)
{
// TODO - STUB - implement this method
return false ;
}
/**
* Utility method to display the statistics for an exam
*
* @param correctAnswerCount number of correct responses per question
* @param studentCount number of students that took the exam
* @param gradedExamCount number of exams that were actually graded (
*/
static void reportStatistics( int[] correctAnswersCount,
int studentCount,
int gradedExamCount
)
{
// TODO - STUB - implement this method
}
}
GradeExamUI
import java.util.Scanner ;
public final class GradeExamsUI
{
/**
* prevent instantiating this class
*/
private GradeExamsUI() {}
/**
* Application to grade multiple-choice exams
*
* @param args -unused-
*/
public static void main( String[] args )
{
// descriptive introductory text
displayIntro() ;
// access the keyboard so user can provide the answer key and students' answers
Scanner keyboard = new Scanner( System.in ) ;
// get the answer key
char[] answerKey = getAnswerKey( keyboard ) ;
// get the students' answers
char[][] studentsAnswers = getStudentsAnswers( keyboard, answerKey ) ;
// release Scanner/keyboard resources
keyboard.close();
// grade the exams: displays the results on a per-student basis and summary statistics
GradeExams.gradeAllExams( answerKey, studentsAnswers );
}
/**
* Utility method to display identifying information and a description of what it does
*/
private static void displayIntro()
{
System.out.println( "Comp 1050, Computer Science II" ) ;
}
/**
*
* @param input
* @return the user-supplied answer key
*/
private static char[] getAnswerKey( Scanner input )
{
// TODO - STUB - replace the following, temporary data with user-supplied information.
char[] answerKey = { 'A', 'B', 'C' } ;
return answerKey ;
}
/**
*
* @param answerKey
* @return
*/
private static char[][] getStudentsAnswers( Scanner input,
char[] answerKey )
{
// TODO - STUB - replace the following, temporary data with user-supplied information.
char[][] studentsAnswers = { { 'A', 'B', 'C' },
{ 'A', 'C', 'C' },
{ 'A', 'B', 'C', 'D' },
{ 'A', 'B' }
} ;
return studentsAnswers ;
}
}
Comp 1050, Computer Science II Lab 2: Grade Exams Due: Su 2/18/2018 This lab grades multiple-choice exams given fixed (hard-coded) student answers and answer key. Information about each student's exam results are displayed, followed by a summary of the performance of all students on a per-question basis. Student Grades: The exam contains 10 questions The correct answers are DBDCCDAEAD Student 1 answered 7 correct ( 70%): -B-CCD-EAD Student 2 answered 6 correct ( 60%): DB--C--EAD Student 3 answered 5 correct ( 50%): --D-C--EAD Student 4 answered 4 correct ( 40%): -B Student 5 answered 8 correct ( 80%): -BDCCD-EAD Student 6 answered 7 correct ( 70%): -B-CCD-EAD Student 7 answered 7 correct ( 70%) : -B-CCD-EAD Student 8 answered 7 c rrect ( 70%) : -B-CCD-EAD Error: Student 9 answered 11 questions: EBECCDEEADX Error: Student 10 answered 9 questions: EBECCDEEA EAD Exam Statistics: 10 students took the exam, 8 exams were graded. Question # Times Correct ( 12%) ( 87%) ( 25%) ( 62%) ( 87%) ( 62%) ( 0%) (100%) (100%) (100%) 7 5 7 0 8 8 8 10 Comp 1050, Computer Science II Lab 2: Grade Exams Due: Su 2/18/2018 This lab grades multiple-choice exams given fixed (hard-coded) student answers and answer key. Information about each student's exam results are displayed, followed by a summary of the performance of all students on a per-question basis. Student Grades: The exam contains 10 questions The correct answers are DBDCCDAEAD Student 1 answered 7 correct ( 70%): -B-CCD-EAD Student 2 answered 6 correct ( 60%): DB--C--EAD Student 3 answered 5 correct ( 50%): --D-C--EAD Student 4 answered 4 correct ( 40%): -B Student 5 answered 8 correct ( 80%): -BDCCD-EAD Student 6 answered 7 correct ( 70%): -B-CCD-EAD Student 7 answered 7 correct ( 70%) : -B-CCD-EAD Student 8 answered 7 c rrect ( 70%) : -B-CCD-EAD Error: Student 9 answered 11 questions: EBECCDEEADX Error: Student 10 answered 9 questions: EBECCDEEA EAD Exam Statistics: 10 students took the exam, 8 exams were graded. Question # Times Correct ( 12%) ( 87%) ( 25%) ( 62%) ( 87%) ( 62%) ( 0%) (100%) (100%) (100%) 7 5 7 0 8 8 8 10
Step by Step Solution
There are 3 Steps involved in it
Step: 1
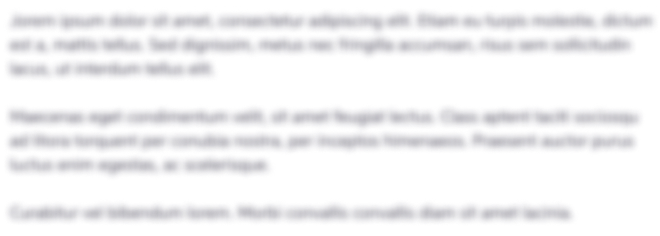
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started