Question
please help. java please create those three classes. these are the provided classes to help with execution import java.util.ArrayList; public class MessageQueue { private ArrayList
please help. java
please create those three classes.
these are the provided classes to help with execution
import java.util.ArrayList;
public class MessageQueue {
private ArrayList
public MessageQueue(){
this.messages = new ArrayList
}
public void addMessage(String s){
this.messages.add(s);
}
public String getMessage(){
if (this.messages.size() > 0){
return this.messages.remove(0);
}
return "";
}
}
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Util{
public static String getCurrentTime(){
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.sss");
Date date = new Date();
String time = dateFormat.format(date); //2016/11/16 12:08:43
return time;
}
}
Now, create a Messenger class that extends from Thread. The goal of the Messenger class is to add messages to the specified MessageQueue. It takes a MessageQueue as a parameter in its constructor, and then later adds to that MessageQueue when it is its turn to run. Because it is a Thread, we will need to put our implementation in the run() method. In the run() method, write a loop that iterates 20 times and inserts a different message into the MessageQueue each time. The message can be whatever you want, but make sure you include a unique identifier with each message (such as the message number being inserted). After inserting each message, the Messenger should sleep for a random amount of time between 0-1 seconds by calling Thread.sleep(). Upon inserting a new message into the MessageQueue, print the inserted message, along with a timestamp, to the console. Make sure the outputs are distinct from other print statements that we will write later. Create a Subscriber class that also extends from Thread. The goal of the Subscriber class is to query the messages in the specified MessageQueue. Again, make sure the Subscriber class takes a MessageQueue in its contractor. In the run() method, it should query the MessageQueue by calling the getMessage() method in the MessageQueue. It should continue to query in a loop until it has read 20 messages. The Subscriber thread should sleep for a random amount of time between 0-1 seconds after attempting to read a message by calling Thread.sleep(). If there is no message, do NOT increment the number of read messages. That will ensure that 20 messages will eventually be read before terminating. Output each message to the console after it has been read, along with a timestamp. If there is no message to be read, print a message to indicate that as well. Again, please make sure the outputs are unique from the other classes (for example, the Messenger class) Now, it is time for us to test the threads that we just wrote. Create a class called MessageTest. This is where we will write out main. In the main, create an instance of each of the classes above (MessageQueue, Messenger, and Subscriber). Make sure of the ExecutorService to help you manage the threads. Create a new executor by calling its constructor: Executors.newFixedThreadPool(size). Add the Messenger and Subscriber to the newly created executor by using the executor.execute(thread) method. There is no need to add MessageQueue because it is not a thread and only servers as our data structure. Do NOT explicitly call the start() method on the threads as they are now managed and executed by the executor. Make sure you call executor.shutdown() after adding the two threads to let the executor know that no more new tasks will be accepted (from the Oracle documentation). The executor.isTerminated() method can help you determine if the two tasks are finished. As long as the two threads are not done, use the Thread. yield() method to allow them to finish in a timely manner. Hint: An if statement only executes once, whereas a while loop keeps looking until the specified condition is met. Have an outer for loop in your main to run the program twice, since multi-threading does not always generate the same output. In each iteration, you will create a new instance for each class. Make sure all threads are finished before moving to the next iteration by using the Thread.yield() method (see the hint above)Step by Step Solution
There are 3 Steps involved in it
Step: 1
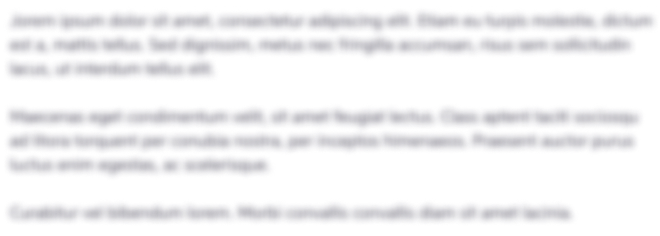
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started