Question
Please use c++ to solve this problem.This is a very long practice. I totally have no idea, so please write comments after important codes if
Please use c++ to solve this problem.This is a very long practice. I totally have no idea, so please write comments after important codes if you can.
---------------The Problem
We are going to work on making our own classes with private data members and special accessors. We are going to build a Circular Buffer class, a common data structure with well known accessors.
-------------Some Background
Our Circular Buffer will be a data structure that stores long values. A Circular Buffer is a fixed size FIFO (First In, First Out) data structure. It is essentially a line (a queue). First thing added (the READ position in the diagram) is the first thing read. The next thing added is at the WRITE position. It is the last thing added, the last thing read. The underlying data structure for this approach has a fixed size data structure. It can become empty, it can become full. It does not grow or shrink in size over the course of the run of the program.
Things you can do with your Circular Buffer:
1.You can add to the Circular Buffer. An element is added at the write position. The write position is then advanced.
2.You can remove an element. The element at the read position is removed. The read position is then advanced.
3.You can test if it is full.
4.You can test if it is empty.
------------------------------------------------------------------------------Here is the task:
Please make a CircBuf class with these characteristics and test it.
The Class:
The CircBuff class will have an underlying data member of your choice called buffer (a vector, a deque) of fixed size. This is a private data member, which will represent the underlying data array. buffer is private, you cannot access it from a main program using the class. Overall, we have 5 underlying elements in the CircBuf. Their need will be shown below.
1.size (the maximum size of the buffer)
2.count (the number of active elements in the buffer)
3.front (an index to the next element that will be read)
4.back (an index to where the next element will be written)
5.buffer (series of longs)
Interface, circbuf.h file:
1.CircBuf constructor, one argument, the fixed size buffer of long.
..............takes a default of 10, thus allowing for a default constructor.
2.long pop_front() member function, no parameters
..............if CircBuf is not empty, returns the long indexed by front and then advances front by one.
..............if the CircBuf is empty, throws a runtime_error, see the notes below about this.
3.bool remove() member function, no parameters
..............if CircBuf is not empty, advances front by one and returns true.
..............if the CircBuf is empty, returns false.
4.bool add(long) member function, single long parameter
..............if CircBuf is not full, places the parameter in buffer at the index indicated by back, advances the back by one and returns true.
..............if CircBuf is full, returns false.
5.bool empty() member function, no parameters
..............returns true if the CircBuf is empty, false otherwise.
6.bool full() member function, no parameters
..............returns true if the CircBuf is full, false otherwise.
7.ostream& operatorostream &out, const CircBuf &cb) This is a friend function (not a member). It prints the underlying buffer array and other elements of the class. Example output:
DUMP Front:0, Back:4, Cnt:2 1, 2, 1, 2,
Implementation, circbuf.cpp:
There are a number of ways to implement this data structure. The important thing to note is the circular nature of the buffer. The two indices: front (where elements are read from) and back (where elements are added). They can wrap around the buffer like we have done with clock arithmetic:
if back goes past the last index of the data structure, it "wraps around" to the first index using the modulus operator (%) based on the fixed buffer size
the same is true for front
in this way you can keep reusing the underlying buffer.
Notes:
1.front == back in two situations: full and empty. How to differentiate full from empty?
2.We talked about handling exceptions, but here is a reminder:
..........you #include
..........to use a particular error, you using std::runtime_error;
..........The C++ keyword is throw. When you throw an exception you are telling C++ and error occurred. That is, you have detected an error condition and are indicating the error to C++. You may or may not have a catcher on the other side to deal with the error, but that is not the focus today.
..........If you try to access the front of an empty CircBuf, you should:
throw runtime_error("Accessing an empty Circular Buffer");
..........There are a couple of predefined errors in C++, one is a runtime_error. That is as good as any other for this case.
..........When you throw the error, you are actually making an instance of the runtime_error class. The constructor can take a string argument which is stored in the error and can be used to help sort out the error.
..........There is an example of a catch (how to deal with an error) in the "main.cpp" if you want to see how it works.
Test:
Implement your class and get it to run with the provided main.cpp. You output should look exactly like the following:
Front:1 DUMP Front:1, Back:2, Cnt:1 1, 2, 0, 0, Front:2 Empty?:true DUMP Front:2, Back:2, Cnt:0 1, 2, 0, 0, Add 4 elements DUMP Front:2, Back:2, Cnt:4 29, 30, 27, 28, Remove all elements Elements removed: 27, 28, 29, 30, DUMP Front:2, Back:2, Cnt:0 29, 30, 27, 28, Fill er up Full?: true DUMP Front:2, Back:2, Cnt:4 4, 9, 0, 1, Drain it Empty?:true Yo, cannot access an empty buffer. Real error msg follows Accessing an empty Circular Buffer
-----------------------------------------Here is the main.cpp
#include using std::cout; using std::cin; using std::endl; #include using std::boolalpha; #include using std::string; #include using std::runtime_error; #include "circbuf.h" int main(){ const size_t sz = 4; CircBuf cb(sz); cout FIFO as a Circular Buffer Head (extract) Tail (insert)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
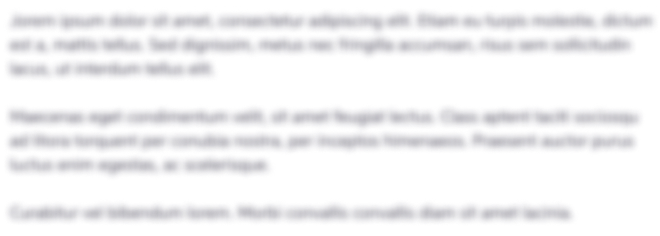
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started