Question
Problem. (Brute-force Implementation) Write a mutable data type BrutePointST that implements the above API using red-black BST (edu.princeton.cs.algs4.RedBlackBST). Corner cases. Throw a java.lang.NullPointerException if any
Problem. (Brute-force Implementation) Write a mutable data type BrutePointST that implements the above API using red-black BST (edu.princeton.cs.algs4.RedBlackBST). Corner cases. Throw a java.lang.NullPointerException if any argument is null. Performance requirements. Your implementation should support put(), get() and contains() in time proportional to the logarithm of the number of points in the set in the worst case; it should support points(), range(), and nearest() in time proportional to the number of points in the symbol table.
$ java BrutePointST 0.661633 0.287141 0.65 0.68 0.28 0.29 5 < data/input10K.txt st.empty()? false st.size() = 10000 First 5 values: 3380 1585 8903 4168 5971 7265 st.contains((0.661633, 0.287141))? true st.range([0.65, 0.68] x [0.28, 0.29]): (0.663908, 0.285337) (0.661633, 0.287141) (0.671793, 0.288608) st.nearest ((0.661633, 0.287141)) = (0.663908, 0.285337) st.nearest ((0.661633, 0.287141), 5): (0.663908, 0.285337) (0.658329, 0.290039) (0.671793, 0.288608) (0.65471, 0.276885) (0.668229, 0.276482)
import edu.princeton.cs.algs4.MinPQ; import edu.princeton.cs.algs4.Point2D; import edu.princeton.cs.algs4.Queue; import edu.princeton.cs.algs4.RectHV; import edu.princeton.cs.algs4.RedBlackBST; import edu.princeton.cs.algs4.StdIn; import edu.princeton.cs.algs4.StdOut;
public class BrutePointST
// Is the symbol table empty? public boolean isEmpty() { ... }
// Number of points in the symbol table. public int size() { ... }
// Associate the value val with point p. public void put(Point2D p, Value val) { ... }
// Value associated with point p. public Value get(Point2D p) { ... }
// Does the symbol table contain the point p? public boolean contains(Point2D p) { ... }
// All points in the symbol table. public Iterable
// All points in the symbol table that are inside the rectangle rect. public Iterable
// A nearest neighbor to point p; null if the symbol table is empty. public Point2D nearest(Point2D p) { ... }
// k points that are closest to point p. public Iterable
// Test client. [DO NOT EDIT] public static void main(String[] args) { BrutePointST
Step by Step Solution
There are 3 Steps involved in it
Step: 1
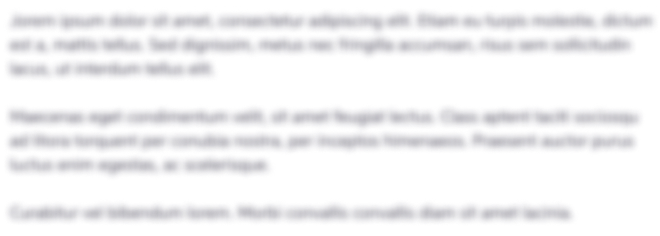
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started