Question
Problem: You are provided an almost-complete program that will read a list of players and a list of game results and produce the final standings.
Problem:
You are provided an almost-complete program that will read a list of players and a list of game results and produce the final standings. Your task is to finish the Tournament class such that it reads in the 2 input files and writes the output file in the proper order.
Requirements:
Implement the Tournament::Tournament(...) constructor provided such that it loads the data from the players input file into the m_Players vector and data from the games input file into the m_Games list without using any user-defined loops.
Implement the Tournament::GenerateResults() function such that it writes the list of players with their scores in descending order by player score without using any user-defined loops.
Submit your updated tournament.h file (and only the tournament.h file) to Blackboard.
Notice that GenerateResults is a const function. This means you cannot modify the data in the Tournament class within it. Take note of the necessary copy-constructors.
You will need to use std::sort. We will not go over the details of this function until later in the semester. For now, all you need to know is that when you pass in the proper iterators and use std::greater
For this assignment, the IDs of the players will match their position in the array. That is, an ID of 0 will correspond to m_Players[0].
tournament.h (only thing that needs to be changed)
#ifndef __TOURNAMENT_H__ #define __TOURNAMENT_H__
#include #include
#include "player.h" #include "game.h"
class Tournament { private: std::vector
void GenerateResults(std::ostream& os) const { // TODO Implement GenerateResults to // 1) Calculate each player's score // 2) Sort the players by score // 3) Write the sorted output to the output stream // All without using custom loops! } };
#endif // __TOURNAMENT_H__
game.h
ifndef __GAME_H__ #define __GAME_H__
#include
class Game { private: unsigned int m_WhitePlayerID; unsigned int m_BlackPlayerID; int m_Score; // 1 = white wins, 0 = draw, -1 = black wins public: Game() : m_WhitePlayerID(0), m_BlackPlayerID(0), m_Score(0.0) {}
unsigned int GetWhitePlayerID() const { return m_WhitePlayerID; }
unsigned int GetBlackPlayerID() const { return m_BlackPlayerID; }
float GetScoreForPlayer(unsigned int id) const { float result = 0.0; if (id == m_WhitePlayerID && m_Score == 1) { result = 1.0; } else if (id == m_BlackPlayerID && m_Score == -1) { result = 1.0; } else if ((id == m_WhitePlayerID || id == m_BlackPlayerID) && m_Score == 0) { result = 0.5; } return result; }
friend std::istream& operator>>(std::istream& is, Game& g); friend std::ostream& operator<<(std::ostream& os, const Game& g); };
std::istream& operator>>(std::istream& is, Game& g) { is >> g.m_WhitePlayerID >> g.m_BlackPlayerID >> g.m_Score; return is; }
std::ostream& operator<<(std::ostream& os, const Game& g) { os << g.m_WhitePlayerID << " " << g.m_BlackPlayerID << " " << g.m_Score; return os; }
#endif // __GAME_H__
main.cpp
include
#include "tournament.h"
int main(int argc, char** argv) { if (argc != 4) { std::cout << "Usage: assignment01 [player input file] [game input file] [output file]" << std::endl; return 0; }
std::ifstream finPlayers(argv[1]); std::ifstream finGames(argv[2]); std::ofstream fout(argv[3]);
std::istream_iterator
Tournament tournament(beginPlayer, endPlayer, beginGame, endGame); tournament.GenerateResults(fout);
return 0;
player.h
Problem:
You are provided an almost-complete program that will read a list of players and a list of game results and produce the final standings. Your task is to finish the Tournament class such that it reads in the 2 input files and writes the output file in the proper order.
Requirements:
Implement the Tournament::Tournament(...) constructor provided such that it loads the data from the players input file into the m_Players vector and data from the games input file into the m_Games list without using any user-defined loops.
Implement the Tournament::GenerateResults() function such that it writes the list of players with their scores in descending order by player score without using any user-defined loops.
Submit your updated tournament.h file (and only the tournament.h file) to Blackboard.
Notice that GenerateResults is a const function. This means you cannot modify the data in the Tournament class within it. Take note of the necessary copy-constructors.
You will need to use std::sort. We will not go over the details of this function until later in the semester. For now, all you need to know is that when you pass in the proper iterators and use std::greater
For this assignment, the IDs of the players will match their position in the array. That is, an ID of 0 will correspond to m_Players[0].
tournament.h (only thing that needs to be changed)
#ifndef __TOURNAMENT_H__ #define __TOURNAMENT_H__
#include #include
#include "player.h" #include "game.h"
class Tournament { private: std::vector
void GenerateResults(std::ostream& os) const { // TODO Implement GenerateResults to // 1) Calculate each player's score // 2) Sort the players by score // 3) Write the sorted output to the output stream // All without using custom loops! } };
#endif // __TOURNAMENT_H__
game.h
ifndef __GAME_H__ #define __GAME_H__
#include
class Game { private: unsigned int m_WhitePlayerID; unsigned int m_BlackPlayerID; int m_Score; // 1 = white wins, 0 = draw, -1 = black wins public: Game() : m_WhitePlayerID(0), m_BlackPlayerID(0), m_Score(0.0) {}
unsigned int GetWhitePlayerID() const { return m_WhitePlayerID; }
unsigned int GetBlackPlayerID() const { return m_BlackPlayerID; }
float GetScoreForPlayer(unsigned int id) const { float result = 0.0; if (id == m_WhitePlayerID && m_Score == 1) { result = 1.0; } else if (id == m_BlackPlayerID && m_Score == -1) { result = 1.0; } else if ((id == m_WhitePlayerID || id == m_BlackPlayerID) && m_Score == 0) { result = 0.5; } return result; }
friend std::istream& operator>>(std::istream& is, Game& g); friend std::ostream& operator<<(std::ostream& os, const Game& g); };
std::istream& operator>>(std::istream& is, Game& g) { is >> g.m_WhitePlayerID >> g.m_BlackPlayerID >> g.m_Score; return is; }
std::ostream& operator<<(std::ostream& os, const Game& g) { os << g.m_WhitePlayerID << " " << g.m_BlackPlayerID << " " << g.m_Score; return os; }
#endif // __GAME_H__
main.cpp
include
#include "tournament.h"
int main(int argc, char** argv) { if (argc != 4) { std::cout << "Usage: assignment01 [player input file] [game input file] [output file]" << std::endl; return 0; }
std::ifstream finPlayers(argv[1]); std::ifstream finGames(argv[2]); std::ofstream fout(argv[3]);
std::istream_iterator
Tournament tournament(beginPlayer, endPlayer, beginGame, endGame); tournament.GenerateResults(fout);
return 0;
player.h
#ifndef __PLAYER_H__ #define __PLAYER_H__
#include
class Player { private: unsigned int m_ID; unsigned int m_Rating; std::string m_LastName; std::string m_FirstName; float m_Score; public: Player() : m_ID(0), m_Rating(0), m_Score(0.0) {}
unsigned int GetID() const { return m_ID; }
void SetID(unsigned int i) { m_ID = i; }
unsigned int GetRating() const { return m_Rating; }
void SetRating(unsigned int r) { m_Rating = r; }
std::string GetLastName() const { return m_LastName; }
void SetLastName(const std::string& s) { m_LastName = s; }
std::string GetFirstName() const { return m_FirstName; }
void SetFirstName(const std::string& s) { m_FirstName = s; }
float GetScore() const { return m_Score; }
void SetScore(float f) { m_Score = f; }
bool operator> (const Player& rhs) const { return m_Score > rhs.m_Score; }
friend std::istream& operator>>(std::istream& is, Player& p); friend std::ostream& operator<<(std::ostream& os, const Player& p); };
std::istream& operator>>(std::istream& is, Player& p) { is >> p.m_ID >> p.m_Rating >> p.m_LastName >> p.m_FirstName >> p.m_Score; return is; }
std::ostream& operator<<(std::ostream& os, const Player& p) { os << p.m_ID << " " << p.m_Rating << " " << p.m_LastName << " " << p.m_FirstName << " " << p.m_Score; return os; }
#endif // __PLAYER_H__
results.txt
3 2840 Carlsen Magnus 7 4 2834 Caruana Fabiano 7 5 2797 Vachier-Lagrave Maxime 7 8 2780 Aronian Levon 6 9 2780 Giri Anish 6 2 2795 Nakamura Hikaru 4 6 2820 Mamedyarov Shakhriyar 3 0 2475 Bartholomew John 2.5 7 2804 Ding Liren 2 1 2550 Tang Andrew 0.5
players.txt
0 2475 Bartholomew John 0.0 1 2550 Tang Andrew 0.0 2 2795 Nakamura Hikaru 0.0 3 2840 Carlsen Magnus 0.0 4 2834 Caruana Fabiano 0.0 5 2797 Vachier-Lagrave Maxime 0.0 6 2820 Mamedyarov Shakhriyar 0.0 7 2804 Ding Liren 0.0 8 2780 Aronian Levon 0.0 9 2780 Giri Anish 0.0
games.txt
0 1 1 2 3 -1 4 5 0 6 7 1 8 9 0 2 0 1 1 8 -1 9 6 0 3 4 0 5 7 1 0 3 -1 9 1 1 4 2 0 6 5 -1 8 7 1 4 0 1 1 2 -1 5 3 0 6 8 -1 7 9 -1 0 5 -1 6 1 1 4 9 0 8 2 0 3 7 1 6 0 0 1 3 -1 7 2 1 4 8 1 9 5 0 0 7 0 5 8 0 2 9 -1 3 6 1 1 4 -1 8 0 1 7 1 0 9 3 0 5 2 1 4 6 1 0 9 0 1 5 -1 6 2 -1 3 8 0 4 7 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
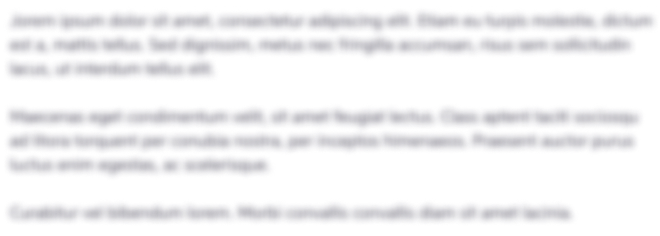
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started