Question
Project 6 Software Implementation Using Test Driven Development Mission: You were hired to implement usingJava a Train system simulation, designed by a developer who suddenly
Project 6
Software Implementation
Using Test Driven Development
Mission:
You were hired to implement usingJava a Train system simulation, designed by a developer who suddenly left the team. You are provided with the design specification document (Project6-Train-SDS.doc) and unit test suite (tests.zip). You are to assume that design description is correct for what each class and method is supposed to do and so are the JUnit tests and your Java code is supposed to match them.
Objectives:
You must implement the system using the given design and make sure that all the unit tests pass against your code. Your program must implement the complete design to include all the classes, methods, constructors, constants, and attributes, and the input and output specification exactly. You cannot redesign or change the system.
Steps:
Create a new project in Eclipse and import the unit tests (tests.zip) and data files into your projects src. Add JUnit library to your Eclipse project
Note that you will have syntax errors in the tests until you create all the classes
The unit test suite is divided into the basic and advanced tests for each Java class.
You should first implement (for each class) the attributes, constants, and empty methods (method signatures) to pass the basic tests.
Then you should start implementing each method running the appropriate tests against it.
To run an individual test, highlight the test method name, right click on it, select Run As and select JUnit Test
Trains simulate method has been implemented for you. Copy and paste the code in simulate.txt to your Train class.
Run the code and unit tests against them until all defects are fixed
For default file tests under TestSimulatorAdvanced you will need to run them individually with and without that file being on your system (C:/train/customer-data.txt). Check comments for tests which tests require the file and which not
Once all the unit tests are working, create a sample input file and run the program with it. Capture the screenshot of the output
Warning: If you use Scanner for reading console and/or file, do not close it because when you try to use again it will not work.
Resources:
Proj6-MSIT660-GradingCriteria.doc lists the grading criteria
You can use any code examples under My Course Content->Code Examples
Deliverables:
Your program files - all .java files (one java file per class)
Sample data file data.txt
Output screenshot paste into Word doc your-name-Output.docx
----------------------------------------------------------------------------------------------------------------------------------------------------------
Software Design Specification
for
Simple Train Simulator
Version 3.0
Prepared by Leva Kurginyan;
Modified by Dr Rand Mcfadden
<05>
Table of Contents
1. Introduction............................................................................................................................... 1
1.1 Purpose................................................................................................................................... 1
1.2 Intended Audience and Reading Suggestions............................................................................. 1
1.3 Product Scope.......................................................................................................................... 1
1.4 Overview................................................................................................................................. 1
2. Design Considerations............................................................................................................... 2
2.1 Assumptions and Dependencies................................................................................................ 2
2.2 General Constraints.................................................................................................................. 2
2.3 Development Methods.............................................................................................................. 2
3. System Architecture.................................................................................................................. 2
3.1 High level Overview of System Architecture (class diagram)...................................................... 2
4. Human Interface Design........................................................................................................... 4
4.1 Interface description................................................................................................................. 4
4.2 Screenshots of Interface............................................................................................................ 4
4.2.1 Prompt for stops and loop if incorrect................................................................................. 4
4.2.2 Prompt for pathname and loop if not exist........................................................................... 5
4.2.3 Check input data and loop if incorrect................................................................................ 5
4.2.4 Display output as simulation runs....................................................................................... 5
5. Detailed System Design............................................................................................................. 6
5.1 Simulator Class........................................................................................................................ 6
5.2 Train class............................................................................................................................... 8
5.3 Customer class....................................................................................................................... 10
Revision History
Name | Date | Reason For Changes | Version |
Leva Kurginyan | 07/22/14 | Instructors feedback updated to Elevator Simulation | 1.1 |
Renata Rand Mcfadden | 07/24/14 | Modified for Train Simulation | 2.0 |
Renata Rand McFadden | 05/09/2015 | Modified for TDD Project | 3.0 |
1. Introduction
1.1 Purpose
This is the Software Design Specification for the simple Train Simulator. This document will define the design of the simulator, by breaking down the project into components to describe in detail what the purpose of each component is and how it will be implemented. It will contain specific information about the expected input, output, and functionality. Also it will explain system constraints, assumptions, dependencies and interface.
1.2 Intended Audience and Reading Suggestions
This Software Design Specification is intended for:
Development team who will develop the first version of the system.
Developers who can review projects capabilities and more easily understand where their efforts should be targeted to improve or add more features to it.
Product testers can use this document as a base for their testing strategy. Also it might be used as a tool for verification and validation of the final product.
1.3 Product Scope
Simple Train Simulator system will be a simple train simulation, implemented with object-oriented approach using Java. It will simulate a train traveling to multiple stops (in a loop starting at stop 1 to stop N) with customers entering and exiting the train. The train will not stop if there are no customers waiting to enter or exit the train at that stop.
User of the program will need to input how many stops the train will have and the absolute path of text file with all customer data. While the simulation is running, it will display data to the console with train stops and customers entering and leaving the train. At the end of the simulation, the program will display how many stops it made to service all customers.
1.4 Overview
The remainder of this document includes 4 chapters.
The second one introduces system constraints and assumptions about the product.
The third chapter gives high level overview of system architecture.
The fourth chapter provides the description of the system interface.
The final fifth chapter provides detailed system design inclooding main data structures, atributes, and metodes used in each class.
2. Design Considerations
2.1 Assumptions and Dependencies
None.
2.2 General Constraints
Implementation environment
Project must be written in Java using Object Orientated approach.
Hardware
None.
2.3 Development Methods
Simple Train Simulator must be implemented using Object Orientated approach.
3. System Architecture
3.1 High level Overview of System Architecture (class diagram)
The three main components of Simple Train Simulator are: Simulator class, Train class and Customer class. Simulator is responsible for prompting and parsing user input data and running simulation. It creates an instance of Train class and N number (depending on data file) of instances of Customer class. It then runs the Train methods for the simulation.
Simulator prompts user for number of stops and the input file with customer data. Parses the file and created a list of customers using Customer class. Creates an instance of Train and runs its methods for the simulation.
Customer stores the four values parsed from file (id, arrival, enter, exit) for the customer. Sets up constants and status variable to determine status where CUST_NOT_PROCESSED means the customer has not been processed yet; CUST_ENTERED means_ the customer is on the train; and CUST_EXITED means the customer was processed and left the train.
Train - stores the number of stops train will have and the customer list. It also has an attribute for storing how many stops the train made as it processed the customers. The Simulator will create an instance of Train and set these values using the constructor. The simulate() method runs the strategy printing the appropriate output. The displayStops method prints the message with the number of stops the train made to process all customers and how long it took.
4. Human Interface Design
4.1 Interface description.
Simple Train Simulator will have text based user interface with textual output and textual prompts where user needs to type in a response.
When program starts, user gets prompted for number of stops the train has on its route: Enter number of stops the train has on its route (must be greater than 1): .
On invalid input, user will get error message Invalid input, try again. " and will be prompted to enter the number of stops again.
On correct input user gets prompted for customer data file name: Enter absolute path for data file or for default (C:/train/customer-data.txt) press Enter: .
If file does not exist, user will get error message File not found, try again. " and will be prompted to input the file path again.
If file has non-integer as one of the first four values, user will get error message Each line must have four integers. Try again. and will be prompted to input the file path again.
If any of the data in the customer file is not valid, user will get error message Data in input file is not correct. Try again. Then user will be prompted to enter another file path.
After user inputs all necessary correct information simulation begins.
During simulation information about each step of simulation (current time, current stop, customers entering train and customers leaving train) is printed out for user.
When simulation is done information about number of stops that were made and how long it took is printed out. The display will be Train made x stops and it took y time units to process all customers with x and y the actual values from the simulation
Program terminates.
4.2 Screenshots of Interface
4.2.1 Prompt for stops and loop if incorrect
Enter number of stops the train has on its route (must be greater than 1): 0
Invalid input, try again
Enter number of stops the train has on its route (must be greater than 1):
Enter number of stops the train has on its route (must be greater than 1): n
Invalid input, try again
Enter number of stops the train has on its route (must be greater than 1):
4.2.2 Prompt for pathname and loop if not exist
Enter absolute path for data file or for default (C:/train/customer-data.txt) press Enter:
blah
File not found, try again.
Enter absolute path for data file or for default (C:/train/customer-data.txt) press Enter:
4.2.3 Check input data and loop if incorrect
# id numbers are duplicate in input file (same for other constraints)
Enter absolute path for data file or for default (C:/train/customer-data.txt) press Enter:
C:/train/customer-bad-id.txt
Data in input file is not correct. Try again.
Enter absolute path for data file or for default (C:/train/customer-data.txt) press Enter:
# non-integer in the input file
Enter absolute path for data file or for default (C:/train/customer-data.txt) press Enter:
C:/train/customer-bad-arrival2.txt
Each line must have four integers. Try again.
Enter absolute path for data file or for default (C:/train/customer-data.txt) press Enter:
4.2.4 Display output as simulation runs
For input file (C:\train\customer-data.txt) with contents:
10 1 1 5
11 1 2 5
12 3 3 1
13 10 4 6
Output is:
Enter number of stops the train has on its route (must be greater than 1): 7
Enter absolute path for data file or for default (C:/train/customer-data.txt) press Enter:
Current Time=1 Current Stop=1
Customer enters train: id=10
Current Time=2 Current Stop=2
Customer enters train: id=11
Current Time=3 Current Stop=3
Customer enters train: id=12
Current Time=5 Current Stop=5
Customer exits train: id=10
Customer exits train: id=11
Current Time=8 Current Stop=1
Customer exits train: id=12
Current Time=11 Current Stop=4
Customer enters train: id=13
Current Time=13 Current Stop=6
Customer exits train: id=13
Train made 7 stops and it took 13 time units to process all customers
5. Detailed System Design
5.1 Simulator Class
This class is responsible for:
Prompting user for all necessary inputs
Reading data file and parsing it
Checking for invalid input
Creating and storing instances of Customer class into ArrayList
Creating an instance of Train class
Running simulation by calling Trains simulate method
Attributes None
Methods
Method | Description |
main() | Runs the program |
void run(int, ArrayList |
|
int getStopsFromUser() | Prompts user for number of stops, checks for invalid data and re-prompts, returns the valid value of stops |
File getInputFile() | Prompts user for input file and checks that file exists. Loops prompting until user gives path for existing file. |
ArrayList | Parses and checks for invalid data. If file has anything invalid, it returns null list. If file has valid values, creates instances of Customer and creates an ArrayList of type Customer and returns the list |
Uses/Interactions
Creates instance of Train class.
Creates instances of Customer class - as many as necessary based on data file.
Simulator class is not used by any other class
Constraints
Number of stops for the train must be entered as numeric (integer) value and greater than 1.
Data file, which is specified by user, must exists and have correct data for each customer.
Customer data constraints:
Each customer must have a unique id, greater than 1, and it must be an integer
The arrival time at the train must be greater than 0 and must be an integer. It represents the time the customer arrives at the train.
The enter stop value must be greater than 0 and an integer. It has to be less than or equal to the highest stop the train has. It representing the stop number of the train where customer wants to enter the train. Stops start at 1 and go up to the N stops that user specified.
The exit stop value must be greater than 0 and integer. It has to be less than or equal to the highest stop the train has. It representing the stop number of the train that customer wants to exit the train.
Customer cannot enter and exit the train at the same stop.
Resources
The only resource that is used by this class is data file specified by user.
Processing
getStopsFromUser(): int
Prompts user to enter number of stops.
Checks input (must be integer > 1) and gives error message if it is not. Loops until correct value is entered.
Returns the obtained value to caller
getInputFile(): File
Loop until the path given is for file that exists
Prompts user for the input file path
If no file is given (user presses enter) then use the default path name (C:/train/customer-data.txt)
Use given path to create File instance
Checks if file exists.
If file does not exist gives an error message and loop until correct path is entered.
Return File instance
checkFile(int stops, File file): ArrayList
Creates empty list (ArrayList
In while loop
Reads file line by line
Parses the four integer values per line
Checks data that it meets the above constraints. If data is incorrect, stops parsing, gives error message, and returns null
Creates instance of customer class (using data from single line from data file)
Stores instance of customer into list.
Exits loop when no more lines to read.
Returns list
run(int stops, ArrayList
Create an instance of Train and pass through constructor stops and custList
Call Trains simulate method
Call Trains displayStops method
main() method:
Creates an instance of Simulation class
Creates custList of type ArrayList
Calls Simulators getStopsFromUser() method and saves the return value in variable (stops)
Loops as long as custList is null
Calls Simulators getInputFile() method. Save the returned File instance
Call Simulators checkFile() method passing it stops and instance of File. Save returned value in custList
Call Simulators run method passing it the stops and custList
5.2 Train class
This class is responsible for:
Picking up customers
Dropping off customer
Moving train (one stop at a time)
Calculating number of stops and the time it took for train to process all customers made and to print out results.
Class Attributes
Attribute | Description |
stops : int | Used to store the number of stops train has on its route. |
madeStops: int | Used to store number of stops the train made to process all customers. |
currTime: int | Used to store the time for the train made to process all customers. |
custList : arrayList | Used to store list of customers to process in the simulation. |
Methods
Method | Description |
Train(int, ArrayList | Constructor to pass values of stops and customer list from Simulator and to initialize Trains attributes |
simulate()
| Runs the train and processes customers entering and exiting the train. Train starts at stop 1 and travels to stop N. The route is a loop so then the train goes to stop 1 again and it keeps running until there are no more customers to process. |
displayStops()
| Displays the message of how many stops the train made and how long it took to process all customers |
Uses/Interactions
The only class that uses Train class is the Simulator class. Train uses Customer class as it processes the list
Constraints
Preconditions all necessary values are passed to instance of Train class by Simulator class (stops, custList).
Processing
Train() constructor:
All attributes are initialized
simulate() method:
Train starts running at current time = 1 and current stop =1.
Make loopAgain = true (Boolean to control the loop)
Loop until no more customers to process Loop for each customer in the list
Check if there is any customers to process (status does not equal Customer CUST_EXITED). If yes then make loopAgain set to true so that when finishes processing this iteration, goes through loop again
Print message with current time and current stop only if there is customer to enter or exit the train
If customer not processed yet and his arrival time is less than or equal to current time and his enter stop is same as current stop, have customer enter the train (customers status gets set to Customer.CUST_ENTERED). Print message about customer entering train.
If customer is in train already (status== Customer.CUST_ENTERED) and exit stop is equal to current stop, have customer exit the train (status gets set to Customer.CUST_EXITED). Print message about customer exiting train.
If a customer entered or exited train, then increment by 1 the madeStop and update currTime
Increment local variable keeping track of time and local variable to keep track of current stop
If current stop == last stop (N), assign 1 to current stop for the train to loop
displayStops() method:
Print message Train made X stops and it took Y time units to process all customers using value of madeStops for X and value of currTime for Y.
5.3 Customer class
This class is responsible for storing and returning (when it is requested) customer information such as customer id, arrival time, enter stop, exit stop, and customers status.
Constants
Constant | Description |
CUST_NOT_PROCESSED: static final int | Assigned value of 0 and means customer has not been processed yet |
CUST_ENTERED: static final int | Assigned value of 1 and means customer entered the train |
CUST_EXITED: static final int | Assigned value of 2 and means customer exited the train and is done processing |
Attributes
Attribute | Description |
id : int | Used to store customers ID |
arrival : int | Used to store customers arrival time at the train |
enter : int | Used to store the stop that customer will enter train |
exit : int | Used to store the stop that customer will exit train |
status : int | Used to track customers status using the constants |
Methods
Method | Description |
Customer(int Custid, int arrivalTime, int enterStop, int exitStop) | Constructor method to set attributes passed from Simulator It also sets status attribute to CUST_NOT_PROCESSED |
void setStatus(int status) | Used to set customers status to the value passed by caller. |
int getStatus() | Returns customers current status |
int getId() | Returns customers ID |
int getArrival() | Returns customers arrival time |
int getEnter() | Returns entered stop |
int getExit() | Returns exit stop |
Uses/Interactions
Customer class is used by Simulator and Train classes.
This class does not use any other classes.
Constraints
All constraints are already described under Simulator class
Processing
All attributes are initialized by constructor method.
When getId() method is called it returns value of id attribute.
When getArrival () method is called it returns value of arrival attribute
When getEnter () method is called it returns value of enter attribute
When getExit () method is called it returns value of exit attribute
When getStatus () method is called it returns value of status attribute
When setStatus(status) method is called it sets passed parameter value as a value of status attribute.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
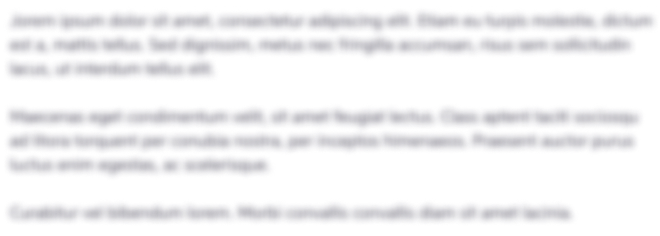
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started