Question
Python All the cards you'll be creating here will have a rank (a number between 0 and 9) and a suit (either stars, suns, moons,
Python
All the cards you'll be creating here will have a rank (a number between 0 and 9) and a suit (either stars, suns, moons, or comets). Some cards are worth more than other cards. Stars are worth more than suns, which are worth more than moons, which are worth more than comets. If the suits are different, then the rank doesn't matter. So the 2 of suns is worth more than the 8 of comets. If the suits are the same, then the card with the higher rank is worth more. So the 5 of stars is worth more than the 0 of stars.
Create a class definition for a playing Card. A playing card needs to store information about its suit(stars, suns, moons, or comets) and rank (0-9). It's up to you to decide the specific details of how things will be represented internally, but to practice encapsulation, make sure that your attributes are all private.
The Card class should have a constructor that can take either two strings (e.g.,Card("0","comets") or Card("7","Star")) or an integer and a string (e.g.,Card(9,"MOONS")). The constructor should not be case sensitive, and it shouldn't care whether or not the suit is plural.
There should be two accessor methods: .get_rank() (which should return the rank as an integer), and .get_suit() (which should return the suit as a string (plural, and in lowercase)). So for example, if you created a Card with the command card1 = Card(0, "comet"), then card1.get_rank() should return 0 and card1.get_suit() should return"comets". Similarly, if you created a Card with the command card2 = Card("5", "moons"), then card2.get_rank() should return 5 and card1.get_suit() should return"moons".
The class should have appropriate .__str__() and .__repr__() methods. You can choose the details of formatting, but as always, the .__str__() method should produce a string suitable for displaying to the user*, and the .__repr__() method should produce a string that you could use as a Python command to create a Card.
*You have lots of options here. You could go with something descriptive (e.g., "7 of stars"), or you could use the unicode escape sequences for the symbols representing a star, sun, moon, or comet (e.g., "7"). Here are those escape sequences, if you want them: \u2600: (sun), \u263D: (moon), \u2605: (star), \u2604: (comet).
You should be able to use == and != to compare Cards. Two Cards should be "equal" if they have the same rank and suit. You should be able to compare cards using >, <, >=, and <=. The "greater" card is the one that is worth more, according to the description at the top of this assignment.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
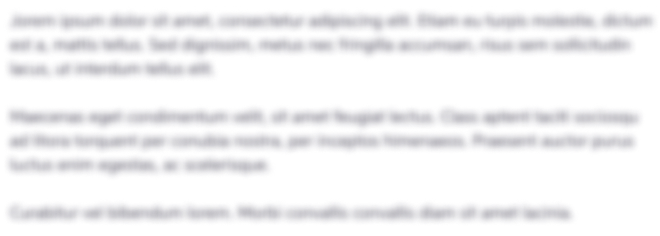
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started