Question
PYTHON: In this question, we will write a program to play the Pig-Game. The Pig-Game is a jeopardy dice game. The object is to be
PYTHON:
In this question, we will write a program to play the Pig-Game. The Pig-Game is a jeopardy dice game. The object is to be the first player to reach 100 points. Each players turn consists of repeatedly rolling a die. After each roll, the player is faced with two choices: roll again, or hold (decline to roll again). If the player rolls a 1, the player scores nothing in that turn, and it becomes the opponents turn.
If the player rolls a number other than 1, the number is added to the players turn total and the players turn continues. If the player holds, the turn total, the sum of the rolls during the turn, is added to the players score, and it becomes the opponents turn. You can read some more about this game at: o https://en.wikipedia.org/wiki/Pig_(dice_game)
o http://cs.gettysburg.edu/projects/pig/index.html You should implement 3 classes: Die, PigGamePlayer, and PigGame
1. The Die class: Will be used to represent a die Each Die instance should have the following data-members:
number_of_faces Will hold the number of faces that the die has. It should be set to 6 by default, unless a different value is given at construction time. curr_face_value Will hold the current face value. A Die object should support following methods:
__init__(self, faces=6) Will initialize a new Die instance.
__repr__(self) Will return a string with the current value showed on the die.
roll(self) Will modify curr_face_value with a random die roll outcome.
2. The PigGamePlayer class: Will be used to represent a player of the Pig-Game Each PigGamePlayer instance should have the following data-members:
name Will hold the players name (of type str)
die Will hold the die that will be used by the player (of type Die)
score Will hold the players score A PigGamePlayer object should support following methods:
__init__(self, name) Will initialize a new PigGamePlayer instance, for a player named name.
play_turn(self) Will interact with the user to play a single turn in a Pig-Game.
3. The PigGame class: Will be used to represent a Pig-Game game Each PigGame instance should have the following data-members:
player1 Will hold the first player (of type PigGamePlayer)
player2 Will hold the second player (of type PigGamePlayer) A PigGame object should support following methods:
__init__(self, player1_name, player2_name)
Will initialize a new PigGame instance, used to play the Pig-Game by 2 players, named player1_name and player2_name. play(self)
Will interact with the user to play the Pig-Game.
You are given the following main function:
def main():
name1 = input("Player #1, enter your name: ")
name2 = input("Player #2, enter your name: ")
game1 = PigGame(name1, name2) game1.play()
Player #1, enter your name: >? Arya Player #2, enter your name: >? Sansa Arya's turn: You rolled 3. Your score for this turn is: 3 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 2. Your score for this turn is: 5 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 3. Your score for this turn is: 8 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 6. Your score for this turn is: 14 Roll again? (type 'r' for roll, or 'h' for hold): >? h You scored 14 points this turn. Your total score is 14 Sansa's turn: You rolled 6. Your score for this turn is: 6 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 2. Your score for this turn is: 8 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 3. Your score for this turn is: 11 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 4. Your score for this turn is: 15 Roll again? (type 'r' for roll, or 'h' for hold): >? h You scored 15 points this turn. Your total score is 15 Arya's turn: You rolled 6. Your score for this turn is: 6 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 1. You scored 0 points this turn. Your total score is 14 Sansa's turn: You rolled 6. Your score for this turn is: 6 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 5. Your score for this turn is: 11 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 6. Your score for this turn is: 17 Roll again? (type 'r' for roll, or 'h' for hold): >? h You scored 17 points this turn. Your total score is 32 Arya's turn: You rolled 1. You scored 0 points this turn. Your total score is 14 Sansa's turn: You rolled 2. Your score for this turn is: 2 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 3. Your score for this turn is: 5 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 4. Your score for this turn is: 9 Roll again? (type 'r' for roll, or 'h' for hold): >? h You scored 9 points this turn. Your total score is 98 Arya's turn: You rolled 4. Your score for this turn is: 4 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 3. Your score for this turn is: 7 Roll again? (type 'r' for roll, or 'h' for hold): >? r You rolled 2. Your score for this turn is: 9 Roll again? (type 'r' for roll, or 'h' for hold): >? h You scored 9 points this turn. Your total score is 87 Sansa's turn: You rolled 4. Your score for this turn is: 4 Roll again? (type 'r' for roll, or 'h' for hold): >? h You scored 4 points this turn. Your total score is 102 Sansa won!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
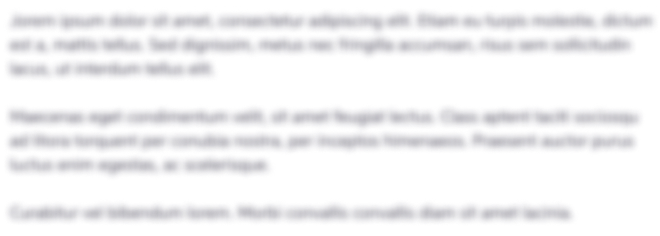
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started