Question
Question: Your program should open a file, read information from the file, and store that information in your linked list implementation. It should also print
Question: Your program should open a file, read information from the file, and store that information in your linked list implementation. It should also print this information once the program has finished running.
The file will contain information on n books (0 n 1000). Your program should read each book from the file, store the information in the associated class objects (book in Book, date in Date, etc.), create a new Node to store that information, and then add the new Node to a linked list. Once your program has finished reading the information it should print out the contents of the linked list formatted as shown in the example at the end of this file.
The following classes must be defined by your program:
class Date : Store information pertaining to a Date
class Book : Store information pertaining to a Book
class Node : Used to construct link list and hold Book objects
class LinkedList : Perform various operations (insert, print, etc.) on the linked list.
The following public members must be defined by the Date class:
unsigned int day;
unsigned int month;
unsigned int year;
The following public functions must be defined by the Date class:
Date(void); : constructor taking no arguments
~Date(void); : deconstructor taking no arguments
The following public members must be defined by the Book class:
string title;
string author;
Date published;
string publisher;
float price;
string isbn;
unsigned int pages;
unsigned int copies;
The following public functions must be defined by the Book class:
Book(void); : constructor taking no arguments
~Book(void); : deconstructor taking no arguments
The following private members must be defined by the Node class:
Book *book; : reference to Book structure
Node *next : reference to next Node in linked list
The following public members must be defined by the Node class:
Node(void); : constructor taking no arguments
Node(Book*); : constructor taking reference to predefined Book as argument
Node(Book*,Node*); : constructor taking references to a predefined Book and Node
~Node(void); : deconstructor taking no arguments
The following private members must defined by the LinkedList class:
Node *head; : reference to first Node in list
Node *tail; : reference to last Node in list
The following public functions must be defined by the LinkedList class:
LinkedList(void); : constructor taking no arguments
LinkedList(Book*) : constructor taking reference to predefined Book
~LinkedList(void) : deconstructor taking no arguments
void insert_front(Book*) : insert Book reference into front of list
void insert_rear(Book*) : insert Book reference into end of list
void print_list(void) : print the contents of the list
** Do not change names
The file will be structured as follows:
Title (string)
Author (string)
Published (unsigned int unsigned int unsigned int)
Publisher (string)
Price (float)
ISBN (string)
Pages (unsigned int)
Copies(unsigned int)
** All information stored in the file will not exceed the bounds of its associated type e.g. the number of pages will not exceed the bounds of an unsigned integer.
You may also assume each line ends with a newline ( ) character. Be careful of the differences between the way Windows and Linux systems terminate the line of a text file. Also, do not hard code your program to read 1000 books from the text file; it should only allocate enough space to hold the exact number of books that are stored in a given file.
Example:
$ cat input.txt
Magician: Apprentice
Raymond E. Feist
12 1 1993
Spectra (January 1, 1994)
5.02
0553564943
512
1
Magician: Master
Raymond E. Feist
12 1 1993
Spectra (January 1, 1994)
7.99
0553564935
499
1
$ g++ book_inventory_linked_list.cpp o submission
$ ./submission
Title: "Magician: Master"
Author: Raymond E. Feist
Published: 12/1/1993
Publisher: Spectra (January 1, 1994)
Price: $7.99
ISBN: 0553564935
Pages: 499
Copies: 1
Title: "Magician: Apprentice"
Author: Raymond E. Feist
Published: 12/1/1993
Publisher: Spectra (January 1, 1994)
Price: $5.02
ISBN: 0553564943
Pages: 512
Copies: 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
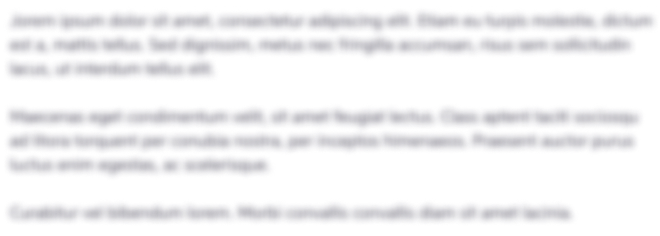
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started