Question
Recursive Maze Solver Help Refresh your knowledge of File I/O. Use recursion to find a path through a maze. Practice throwing and handling exceptions. Description
Recursive Maze Solver Help
Refresh your knowledge of File I/O.
Use recursion to find a path through a maze.
Practice throwing and handling exceptions.
Description
This assignment creates a maze that has a leprechaun in search of a pot of gold at the end of a rainbow. Some squares are empty while others are blocked by trees. Your code must find the path between the leprechaun and the pot of gold, without running into any trees or going outside the maze.
I need help implementing the following methods in the MazeSolver class:
loadMaze
main
findPath
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Scanner;
public class MazeSolver {
/**
* Exception thrown when path is complete
*/
public static class MazeSolvedException extends Exception {
private static final long serialVersionUID = 1L;
MazeSolvedException() {
super("Found the pot of gold!");
}
}
/**
* Stores the user interface
*/
private static UserInterface gui;
/**
* Data structures for maze
*/
private static char[][] maze;
/**
* This is the starting point for your program.
* This method calls the loadMaze method to read the maze file.
*
- Implement the {@link MazeSolver#loadMaze(String)} method first,
* if correctly implemented the user interface should display it correctly.
*
- After the //YOUR CODE HERE! comment write code to find the row and column of the leprechaun,
* which is then used for the initial call to {@link MazeSolver#findPath(int, int)}.
*
- Since code has been provided to CALL the method {@link MazeSolver#findPath(int, int)},
* no other code in the main method is needed.
*
*
* @param args set run configurations to either choose or change the maze you are using
* @throws FileNotFoundException exception thrown when program unable to recognize file name
*/
public static void main(String[] args) throws FileNotFoundException {
// Load maze from file
loadMaze(args[0]);
// Find leprechaun in maze
int currentRow = -1;
int currentCol = -1;
// YOUR CODE HERE!
// Instantiate graphical user interface
gui = new UserInterface(maze);
try {
// Solve maze, using recursion
findPath(currentRow, currentCol);
// Impossible maze, notify user interface
gui.sendStatus(CallbackInterface.SearchStatus.PATH_IMPOSSIBLE, -1, -1); // Cannot solve
} catch (MazeSolvedException e) {
// Maze solved, exit normally
}
}
/**
* Reads a maze file into the 2-dimensional array declared as a class variable.
*
* @param filename the name of the maze file.
* @throws FileNotFoundException exception thrown when program unable to recognize file name
*/
static void loadMaze(String filename) throws FileNotFoundException {
}
/**
* This method calls itself recursively to find a path from the leprechaun to the pot of gold.
* It also notifies the user interface about the path it is searching.
* Here is a list of the actions taken in findPath, based on the square it is searching:
*
*
- If the row or column is not on the board, notify the user interface calling
* sendStatus with PATH_ILLEGAL, and return false.
*
- If maze[row][col] == 'G', then you have found a path to the pot of gold, notify the user
* interface by calling sendStatus with PATH_COMPLETE and throw the MazeSolvedException to terminate execution.
*
- If maze[row][col] == 'S', then the current square is already part of a valid path,
* do not notify the user interface, just return false.
*
- If maze[row][col] == 'W', then the current square is already part of an invalid path,
* not notify the user interface, just return false.
*
- If maze[row][col] == '#', then the current square contains a blocking tree,
* do not notify the user interface, just return false.
*
- If current square contains a space or 'L', notify the user interface by calling sendStatus with PATH_VALID,
* then recursively call findPath with the row and column of the surrounding squares,
* in order: UP, RIGHT, DOWN, and LEFT.
*
- If any of the recursive calls return true, return true from the current invocation of findPath,
* otherwise notify the user interface by calling sendStatus with PATH_INVALID, and return false.
*
*
*
* @param row the current row of the leprechaun
* @param col the current column of the leprechaun
* @return true for a valid path, false otherwise
* @throws MazeSolvedException exception thrown when maze is complete
*/
public static boolean findPath(int row, int col) throws MazeSolvedException {
// YOUR CODE HERE!
return false;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
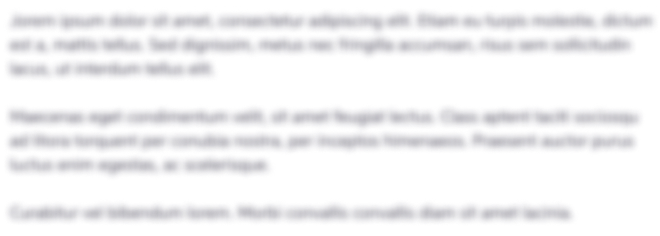
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started