Question
Stuck on a java program You are to write an application to presell a limited number of movie tickets. The simple user interface will be
Stuck on a java program
You are to write an application to presell a limited number of movie tickets. The simple user interface will be contained in Program.java and the object to implement this is referred to as TicketSeller and consists of only the following public methods:
public TicketSeller(int initialTicketAllotment) - Specify the number of tickets you want to sell.
public int requestTickets(int ticketRequest) - Allows buyer to request a specific number of tickets. Returns the number of tickets remaining if the number requested is within the max allowable and does not exceed the number remaining. Returns TicketSeller.TOO_MANY_TICKETS_REQUESTED if there are fewer remaining tickets than requested or the number of requested exceeds TicketSeller.MAXIMUM_TICKETS_ALLOWED. Since the normal result is going to be zero or more tickets (up to the maximum allowed), use a negative number to represent the constant value for too many tickets requested. Note that one of these constants is private and one is public. Which one needs to be exposed to the user?
public int getNumberOfBuyers() - Tracks number of ticket buyers. You only count buyers who have fulfilled a request. Errors don't count.
Your Program.java should contain code to test your TicketSeller object.
Note:
Each buyer can buy no more than as 4 tickets.
Your requests for tickets will always be positive integers.
You can pre-sell 0 tickets. Note that all requests but a request for 0 tickets are requesting too many tickets.
Getting Started
We are going to do this exercise by writing the object that solves the problem first (in a source file called TicketSeller.java) and then testing it using code we write into Program.java. Using the techniques shown on the web page titled "How to Start Every Project in this Class" create a source file called TicketSeller.java as well as a file called Program.java.
There is no code to copy for the assignment. You get to do it all! Don't forget to provide proper Javadoc documentation.
Testing Your Code
Your test code for this project will be in Program.java and will implement a simple user interface. Here is a sample run:
Input the number of tickets you want to sell: 8
Input requested tickets: 1 Thank you for your purchase. There are 7 tickets remaining.
Input requested tickets: 2 Thank you for your purchase. There are 5 tickets remaining.
Input requested tickets: 5 Too many tickets requested, please try again.
Input requested tickets: 9 Too many tickets requested, please try again.
Input requested tickets: 3 Thank you for your purchase. There are 2 tickets remaining.
Input requested tickets: 3 Too many tickets requested, please try again.
Input requested tickets: 2 Thank you for your purchase. There are no tickets remaining.
You have had 4 buyers.
The text in blue bold refers to user inputs. Notice that only the valid ticket purchases make someone count as a buyer. Don't worry about other inputs; you'll always get positive integers.
Once you've written your code, run the code by single clicking on Program.java in the package explorer and selecting Run->Run from the menu or using the keyboard shortcut. Examine the output. Does it do what you want? If not, how can you modify the code to do what you want?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
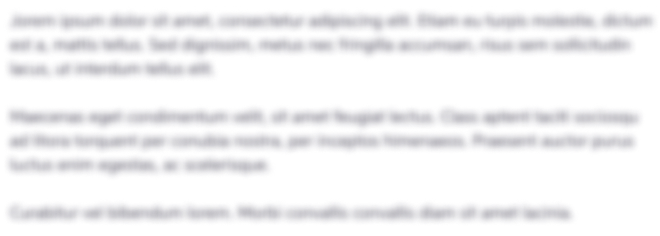
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started