Question
Summary In this lab, you add the input and output statements to a partially completed Java program. When completed, the user should be able to
Summary
In this lab, you add the input and output statements to a partially completed Java program. When completed, the user should be able to enter a year, a month, and a day to determine if the date is valid. Valid years are those that are greater than 0, valid months include the values 1 through 12, and valid days include the values 1 through 31.
Instructions
Notice that variables have been declared for you.
Write the simulated housekeeping() method that contains input statements to retrieve a year, a month, and a day from the user.
Add statements to the simulated housekeeping() method that convert the String representation of the year, month, and day to ints.
Include the output statements in the simulated endOfJob()method. The format of the output is as follows:
month/day/year is a valid date. or month/day/year is an invalid date.
Execute the program entering the following date:
month = 5, day = 32, year =2014
Observe the output of this program. Execute the program entering the following date:
month = 9, day = 21, year = 2002
Observe the output of this program.
/** * The java program BadDate.java that prompts * user to enter year, month and day and finds * if the user given date is valid or not * and display on a message dialog box. * */ //BadDate.java import javax.swing.JOptionPane; public class BadDate { public static void main(String args[]) { String yearString; String monthString; String dayString;
// Get the year, then the month, then the day yearString=housekeeping("Enter a Year : "); monthString=housekeeping("Enter a Month : "); dayString=housekeeping("Enter a Day : "); //calling detailLoop detailLoop(yearString,monthString,dayString);
}
private static void detailLoop(String yearString, String monthString, String dayString) { int year; int month; int day; boolean validDate = true; final int MIN_YEAR = 0, MIN_MONTH = 1, MAX_MONTH = 12, MIN_DAY = 1, MAX_DAY = 31;
// Convert Strings to integers year=Integer.parseInt(yearString); month=Integer.parseInt(monthString); day=Integer.parseInt(dayString);
// Check to be sure date is valid if( year <= MIN_YEAR ) // invalid year validDate = false; else if ( month < MIN_MONTH || month > MAX_MONTH ) // invalid month validDate = false; else if ( day < MIN_DAY || day > MAX_DAY ) // invalid day validDate = false; String result=""; // This is the work of the endOfJob() method // Test to see if date is valid and output date and whether it is valid or not if( validDate == true ) { result="Date DD/MM/YYYY : "+day+"/"+month+"/"+year+" is valid";
JOptionPane.showMessageDialog(null, result, "Result", JOptionPane.INFORMATION_MESSAGE);
} else { result="Date DD/MM/YYYY : "+day+"/"+month+"/"+year+" is in-valid"; // Output statement JOptionPane.showMessageDialog(null, result, "Result", JOptionPane.INFORMATION_MESSAGE);
}
}
//Method that takes a string and prompts user to enter input private static String housekeeping(String prompt) { String yearString=JOptionPane.showInputDialog(null,prompt); return yearString; } // end of main() method
} // end of BadDate class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
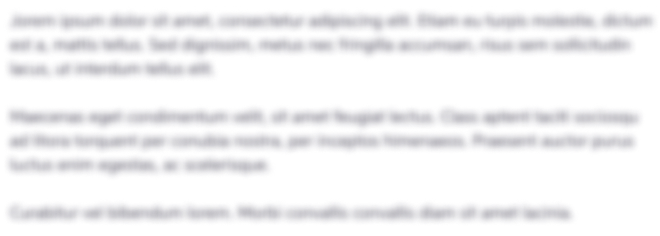
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started