Task 1: Weapons (6 marks) In order to survive in the Hungry Games, our tributes would...
Fantastic news! We've Found the answer you've been seeking!
Question:
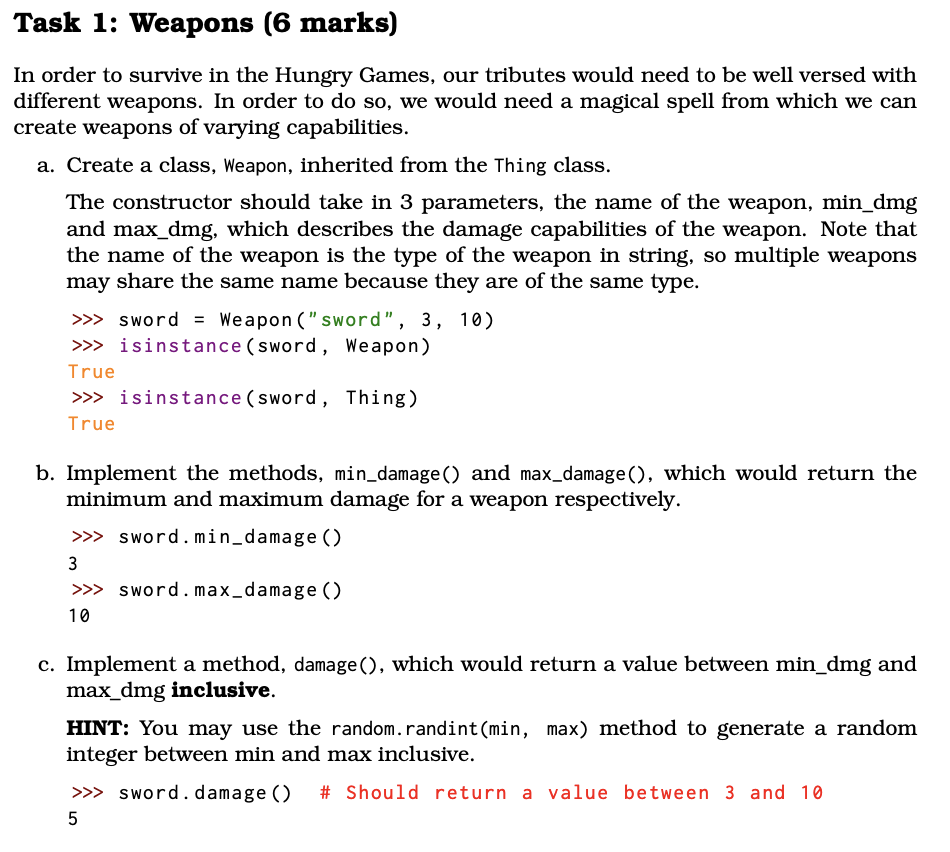
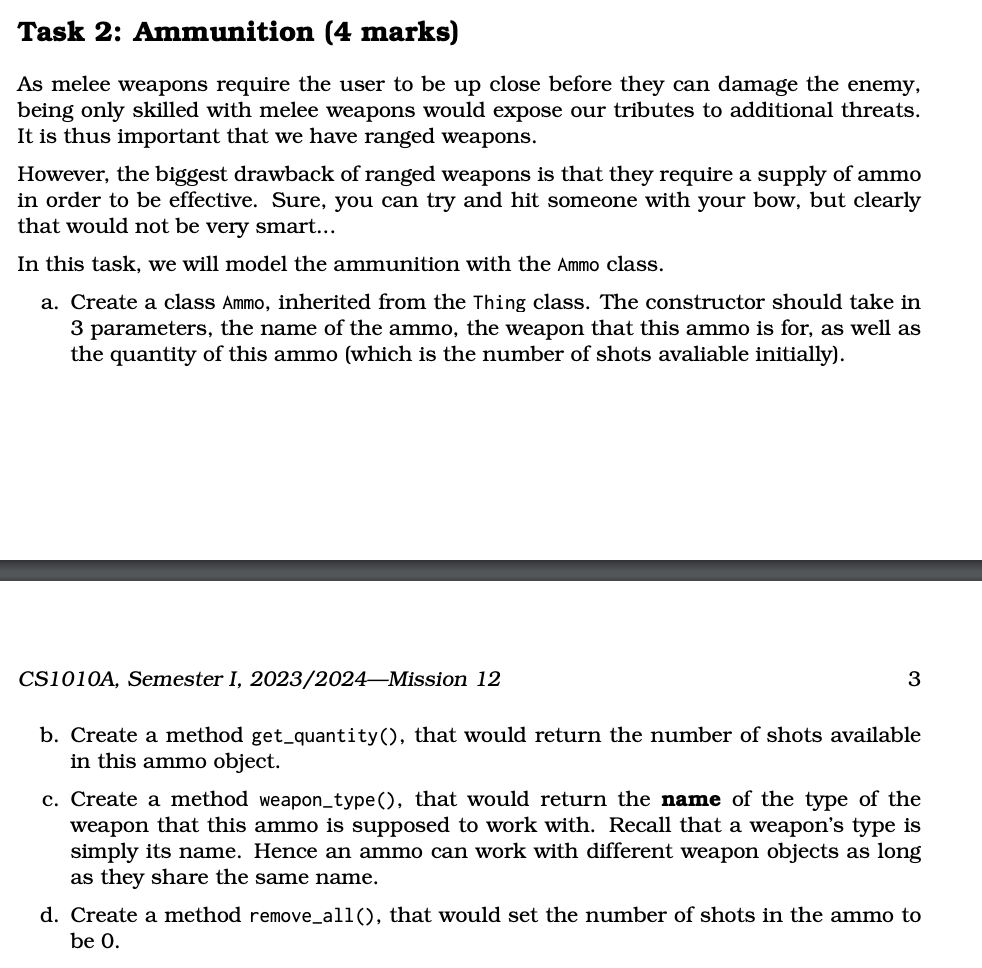
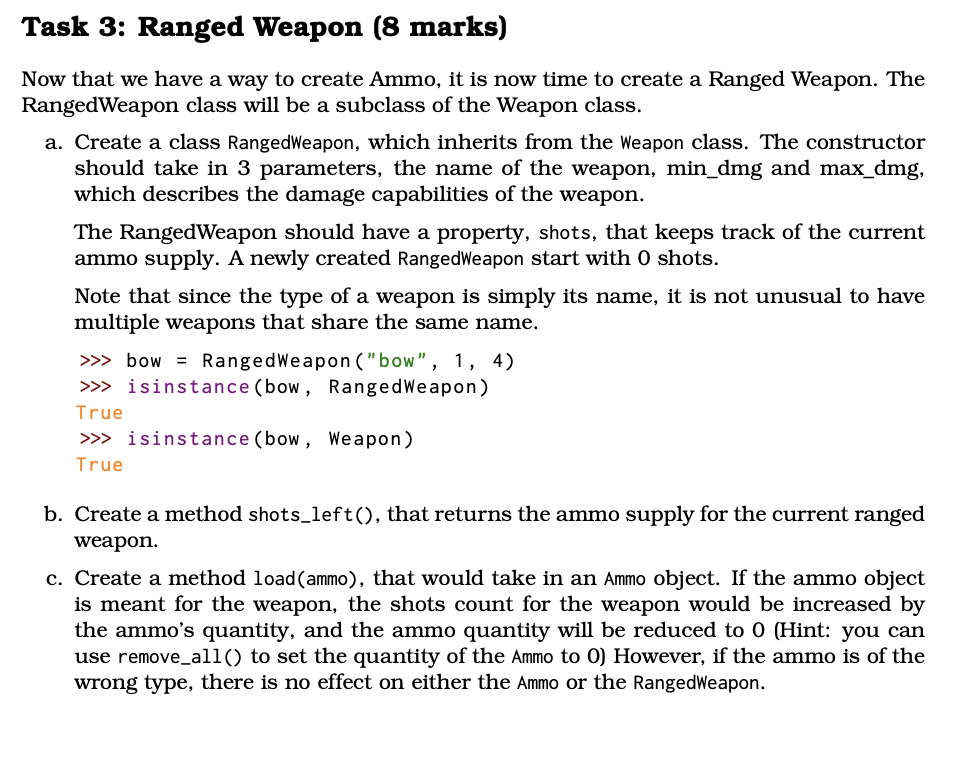
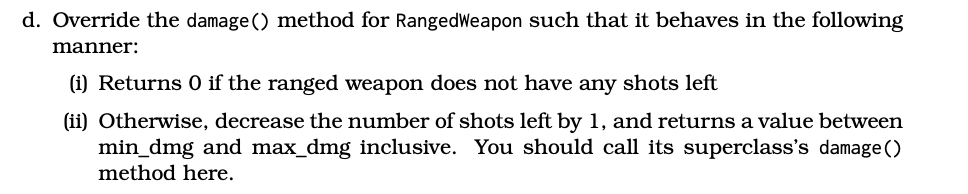
Transcribed Image Text:
Task 1: Weapons (6 marks) In order to survive in the Hungry Games, our tributes would need to be well versed with different weapons. In order to do so, we would need a magical spell from which we can create weapons of varying capabilities. a. Create a class, Weapon, inherited from the Thing class. The constructor should take in 3 parameters, the name of the weapon, min_dmg and max_dmg, which describes the damage capabilities of the weapon. Note that the name of the weapon is the type of the weapon in string, so multiple weapons may share the same name because they are of the same type. >>> sword = Weapon ("sword", 3, 10) >>> isinstance (sword, Weapon) True >>> isinstance (sword, Thing) True b. Implement the methods, min_damage () and max_damage(), which would return the minimum and maximum damage for a weapon respectively. >>> sword.min_damage () 3 >>> sword.max_damage () 10 c. Implement a method, damage (), which would return a value between min_dmg and max_dmg inclusive. HINT: You may use the random.randint(min, max) method to generate a random integer between min and max inclusive. >>> sword. damage () # Should return a value between 3 and 10 5 Task 2: Ammunition (4 marks) As melee weapons require the user to be up close before they can damage the enemy, being only skilled with melee weapons would expose our tributes to additional threats. It is thus important that we have ranged weapons. However, the biggest drawback of ranged weapons is that they require a supply of ammo in order to be effective. Sure, you can try and hit someone with your bow, but clearly that would not be very smart... In this task, we will model the ammunition with the Ammo class. a. Create a class Ammo, inherited from the Thing class. The constructor should take in 3 parameters, the name of the ammo, the weapon that this ammo is for, as well as the quantity of this ammo (which is the number of shots avaliable initially). CS1010A, Semester I, 2023/2024-Mission 12 3 b. Create a method get_quantity (), that would return the number of shots available in this ammo object. c. Create a method weapon_type(), that would return the name of the type of the weapon that this ammo is supposed to work with. Recall that a weapon's type is simply its name. Hence an ammo can work with different weapon objects as long as they share the same name. d. Create a method remove_all(), that would set the number of shots in the ammo to be 0. Task 3: Ranged Weapon (8 marks) Now that we have a way to create Ammo, it is now time to create a Ranged Weapon. The RangedWeapon class will be a subclass of the Weapon class. a. Create a class RangedWeapon, which inherits from the Weapon class. The constructor should take in 3 parameters, the name of the weapon, min_dmg and max_dmg, which describes the damage capabilities of the weapon. The RangedWeapon should have a property, shots, that keeps track of the current ammo supply. A newly created RangedWeapon start with 0 shots. Note that since the type of a weapon is simply its name, it is not unusual to have multiple weapons that share the same name. >>> bow = RangedWeapon ("bow", 1, 4) >>> isinstance (bow, RangedWeapon) True >>> isinstance (bow, Weapon) True b. Create a method shots_left(), that returns the ammo supply for the current ranged weapon. c. Create a method load(ammo), that would take in an Ammo object. If the ammo object is meant for the weapon, the shots count for the weapon would be increased by the ammo's quantity, and the ammo quantity will be reduced to 0 (Hint: you can use remove_all() to set the quantity of the Ammo to 0) However, if the ammo is of the wrong type, there is no effect on either the Ammo or the RangedWeapon. d. Override the damage () method for RangedWeapon such that it behaves in the following manner: (i) Returns 0 if the ranged weapon does not have any shots left (ii) Otherwise, decrease the number of shots left by 1, and returns a value between min_dmg and max_dmg inclusive. You should call its superclass's damage () method here. Task 1: Weapons (6 marks) In order to survive in the Hungry Games, our tributes would need to be well versed with different weapons. In order to do so, we would need a magical spell from which we can create weapons of varying capabilities. a. Create a class, Weapon, inherited from the Thing class. The constructor should take in 3 parameters, the name of the weapon, min_dmg and max_dmg, which describes the damage capabilities of the weapon. Note that the name of the weapon is the type of the weapon in string, so multiple weapons may share the same name because they are of the same type. >>> sword = Weapon ("sword", 3, 10) >>> isinstance (sword, Weapon) True >>> isinstance (sword, Thing) True b. Implement the methods, min_damage () and max_damage(), which would return the minimum and maximum damage for a weapon respectively. >>> sword.min_damage () 3 >>> sword.max_damage () 10 c. Implement a method, damage (), which would return a value between min_dmg and max_dmg inclusive. HINT: You may use the random.randint(min, max) method to generate a random integer between min and max inclusive. >>> sword. damage () # Should return a value between 3 and 10 5 Task 2: Ammunition (4 marks) As melee weapons require the user to be up close before they can damage the enemy, being only skilled with melee weapons would expose our tributes to additional threats. It is thus important that we have ranged weapons. However, the biggest drawback of ranged weapons is that they require a supply of ammo in order to be effective. Sure, you can try and hit someone with your bow, but clearly that would not be very smart... In this task, we will model the ammunition with the Ammo class. a. Create a class Ammo, inherited from the Thing class. The constructor should take in 3 parameters, the name of the ammo, the weapon that this ammo is for, as well as the quantity of this ammo (which is the number of shots avaliable initially). CS1010A, Semester I, 2023/2024-Mission 12 3 b. Create a method get_quantity (), that would return the number of shots available in this ammo object. c. Create a method weapon_type(), that would return the name of the type of the weapon that this ammo is supposed to work with. Recall that a weapon's type is simply its name. Hence an ammo can work with different weapon objects as long as they share the same name. d. Create a method remove_all(), that would set the number of shots in the ammo to be 0. Task 3: Ranged Weapon (8 marks) Now that we have a way to create Ammo, it is now time to create a Ranged Weapon. The RangedWeapon class will be a subclass of the Weapon class. a. Create a class RangedWeapon, which inherits from the Weapon class. The constructor should take in 3 parameters, the name of the weapon, min_dmg and max_dmg, which describes the damage capabilities of the weapon. The RangedWeapon should have a property, shots, that keeps track of the current ammo supply. A newly created RangedWeapon start with 0 shots. Note that since the type of a weapon is simply its name, it is not unusual to have multiple weapons that share the same name. >>> bow = RangedWeapon ("bow", 1, 4) >>> isinstance (bow, RangedWeapon) True >>> isinstance (bow, Weapon) True b. Create a method shots_left(), that returns the ammo supply for the current ranged weapon. c. Create a method load(ammo), that would take in an Ammo object. If the ammo object is meant for the weapon, the shots count for the weapon would be increased by the ammo's quantity, and the ammo quantity will be reduced to 0 (Hint: you can use remove_all() to set the quantity of the Ammo to 0) However, if the ammo is of the wrong type, there is no effect on either the Ammo or the RangedWeapon. d. Override the damage () method for RangedWeapon such that it behaves in the following manner: (i) Returns 0 if the ranged weapon does not have any shots left (ii) Otherwise, decrease the number of shots left by 1, and returns a value between min_dmg and max_dmg inclusive. You should call its superclass's damage () method here.
Expert Answer:
Answer rating: 100% (QA)
Heres the implementation of the requested Weapon class python import random c... View the full answer
Related Book For
Business Communication Essentials a skill based approach
ISBN: 978-0132971324
6th edition
Authors: Courtland L. Bovee, John V. Thill
Posted Date:
Students also viewed these programming questions
-
Among the BRFSS participants, the linear relationship between body-mass index (BMI) BMI and drinking frequency is to be compared between those who reported any exercise in the previous month...
-
Design a Java class that represents a cache with a fixed size. It should support operations like add, retrieve, and remove, and it should evict the least recently used item when it reaches capacity.
-
Planning is one of the most important management functions in any business. A front office managers first step in planning should involve determine the departments goals. Planning also includes...
-
As shown in Fig. 4.60, a horizontal beam is hinged to the wall at point A. The length of the beam is 1 = 2 m and it weighs W = 150 N. Point C is the center of gravity of the beam and it is...
-
CC10Natalie is also thinking of buying a van that will be used only for business. The cost of the van is estimated at $36,500. Natalie would spend an additional $2,500 to have the van painted. In...
-
Let μn denote the nth central moment of a random variable X. Two quantities of interest, in addition to the mean and variance, are The value α3 is called the skewness and...
-
Using the data in Table 8.8, calculate the profit multipliers and review your answers by producing a full profit sensitivity analysis report.
-
Selected accounts from the adjusted trial balance of Daniel's Sports Equipment on September 30, 2010, the fiscal year end. The company's beginning merchandise inventory was $81,222 and ending...
-
Lou Barlow, a divisional manager for Sage Company, has an opportunity to manufacture and sell one of two new products for a five- year period. His annual pay raises are determined by his division's...
-
In Alahmadi During a cold winter day, wind at 55 km/h is blowing parallel to a 4-m-high and 10-m-long wall of a house. If the air outside is at 5°C and the surface temperature of the wall is...
-
William, 31 years old received Canada Recovery Benefits in 2021 during the Covid 19 pandemic. He reported $6000 of income on his 2021n return. In 2022, william received a letter of redetermination...
-
FUNCTIONAL DEPENDENCY A set of Functional Dependencies for a data model has been documented in a Functional Dependency Diagram shown below. Each attribute is shown in a rectangle with an arrow...
-
DATABASE ALTERNATIVES Represent the following Bible verses on strength and courage from the New King James Version using XML notation: [3 marks] 1 Chronicles 16:11 Look to the Lord and his strength;...
-
Q13.A European call with strike $20 expires in one month. The underlying asset of this call has current value $22. The yearly volatility is 30% and the current interest rate is 4% pa. Using the...
-
Q1 database management system List and explain the methods used for resolution the external hashing collision
-
A. Write a short code to implement the simple semaphore operation sem Wait(semaphore S) Answer: B. Write a short code to implement the simple semaphore operation semSignal(semaphore S) Answer: C....
-
Required information Exercise 5-5 (Algo) Changes in Variable Costs, Fixed Costs, Selling Price, and Volume [LO5-4] [The following information applies to the questions displayed below.] Data for...
-
Data on weekday exercise time for 20 females, consistent with summary quantities given in the paper An Ecological Momentary Assessment of the Physical Activity and Sedentary Behaviour Patterns of...
-
The consumer reviews on Yelp (www.yelp.com) can be a promotional boon to any local business-provided the reviews are positive, of course. Negative reviews, fair or not, can affect a company's...
-
What is parallel construction, and why is it important?
-
How can you distinguish yourself from other candidates in a screening interview and still keep your responses short and to the point? Explain. Discuss.
-
Chelsea Fabricating applies variable overhead to products on the basis of standard direct labor hours. Presented is selected information for last month when 10,000 units were produced. Required Solve...
-
Presented are partial flexible cost budgets for various levels of output. Required Solve for items a though n. Direct materials Direct labor.. Variable overhead.. Fixed overhead.. Total Rate per unit...
-
Petra Company uses standard costs for cost control and internal reporting. Fixed costs are budgeted at \($36,000\) per month at a normal operating level of 10,000 units of production output. During...

Study smarter with the SolutionInn App