Question
This assignment demonstrates your understanding of the various issues around Synchronization. Before attempting this project, be sure you have completed all of the reading assignments,
This assignment demonstrates your understanding of the various issues around Synchronization. Before attempting this project, be sure you have completed all of the reading assignments, hands-on labs, discussions, and assignments to date. For this weeks Homework I want you to go back to the week 2 sample provided as an attachment. You can take either the C# or Java version provided in the Activity and I want you to fix it for me. If you run the sample multiple times it will give different final values. There is a timing issue with the sample which would be a major problem if it actually was a production program for a bank. I want you to fix the program so that you get 800 as the final value every time it is run. For this I want you to use one of the Java constructs like synchronize, countdownlatch or semaphore. C# has similar constructs. I do not want you changing the accountbalance from being a static nor do I want you to remove the initialize to 0 in the threads. If you are going to synchronize you want to synchronize as small a section as possible. It does little good to synchronize a large part of a threaded program... because it might as well not be threaded. Finally, I want you to replace the sleep in the main with some other mechanism to force the main to wait until all child threads are finished. Run the program 3 times and make sure you show the final output in the documentation.
this is the code
//********************************************************************** // File: threadproj.java // Description: // The purpose of this file is illustrate the suspending and resuming of // a thread. // Author: Mike Tarquinio // //********************************************************************** package cmis412; //********************************************************************** // class: ThreadClient // // description: // This class is a simple illustration of a CPU bound thread // //********************************************************************* class ThreadClient extends Thread { // local counter long counter = 0; public void mySuspend() { synchronized (this) { this.suspend(); } } synchronized public void myResume() { synchronized (this) { this.resume(); } } // ****************************************************************** // method: account // // parameters: // none // // return: // none // // description: // Constructor. // // ******************************************************************** public void ThreadClient() { System.out.println("ThreadClient constructed"); } // end ThreadClient // ****************************************************************** // method: run // // parameters: // none // // return: // none // // description: // This is the run method. It constains the code that will actually // be run when the thread is started. // ******************************************************************** public void run() { // simple for loop for (long iii = 1; iii < 100000000000L; iii++) { counter++; } // end for synchronized (this) { System.out.println("ThreadClient Run Loop - done"); } } // end run } // end ThreadMain // ********************************************************************** // class: threadproj // // description: // This class is the public class for this file. It contains the // main and is the controller for the other threads. This could // be where you would implement your scheduling algorithm // // ********************************************************************* public class threadproj { // ****************************************************************** // method: main // // parameters: // String args[] - command line parameters // // return: // none // // description: // This is the main for this class. It is a static method and thus // is part of the class but is not part of any of the instantiated // objects. It is where a thread could be created and started. // This is the first code that gets run when this file is run. // ******************************************************************** public static void main(String args[]) throws InterruptedException { System.out.println("ThreadProj Start"); // create a new instantiation of a ThreadClient class ThreadClient threadClient = new ThreadClient(); System.out.println("ThreadMain - at start counter = :" + threadClient.counter); // start the just created ThreadClient object. This will kick off // the threads run method. threadClient.start(); System.out.println("ThreadMain after start"); while (threadClient.isAlive()) { Thread.sleep(1); if (threadClient.isAlive()) { System.out .println("ThreadMain suspend: counter:" + threadClient.counter); threadClient.mySuspend(); } if (threadClient.isAlive()) { System.out.println("ThreadMain before resume: counter:" + threadClient.counter); threadClient.myResume(); System.out.println("ThreadMain resume: counter:" + threadClient.counter); } } // end while System.out.println("ThreadMain - at exit counter = :" + threadClient.counter); System.out.println("ThreadMain Exit"); } } // end ThreadMain
Step by Step Solution
There are 3 Steps involved in it
Step: 1
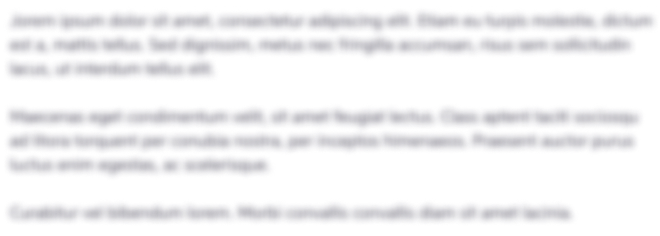
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started