Question
This is all done in C code using Netbeans IED This lab does not have a problem scenario. It is just an exercise in reading
This is all done in C code using Netbeans IED
This lab does not have a problem scenario. It is just an exercise in reading in data from file that has an unknown number of ids and codes. Because of this, you need to use dynamic allocation of memory to properly analyze the data. The strategy that will be followed is 1. Count the number of ids - open the file for the sole purpose of counting the number of unique IDs. This will be done in a function that takes a filename as an argument and returns the number of ids. 2. Count the number of codes - open the file for the sole purpose of counting the number of unique codes. This will be done in a function that takes a filename as an argument and returns the number of codes. 3. Read in the data for analysis - open the file with the purpose of updating an allocated array that contains the sales information identified by an id and code. This will be done in a function that takes a filename, the number of ids and the number of codes. After the file is opened and the data loaded to a properly sized and allocated block of memory, it will print out a report of all the totals, as shown at the end of this write-up.
It is recommended that you do this program one step at a time as enumerated above. Do not proceed to the 2 step before you have successfully completed step one and definitely do not
proceed to the last step until the first two are successfully completed. You have been given starter code that included the prototypes for the function you must define and use. DO NOT CHANGE ANYTHING in the main function of this code. You will lose points if changes are made to prototypes or code in main function.
###############################// Starter Code //####################################################
#include
#include
int count_ids(char fileName[]);
int count_codes(char fileName[]);
void printReport(char fileName[], int num_ids, int num_codes);
int main(int argc, char** argv) {
int number_ids;
int number_codes;
char fileName[] = "sales_person_Data_1.csv";
//////////////////////////////////////////////////////
///// When the functions are complete, uncomment the
///// corresponding code below This is the only
///// change allowed in this main function
//////////////////////////////////////////////////////
///// un-comment the two lines below to test STEP 1
//////////////////////////////////////////////////////
// number_ids = count_ids(fileName);
// printf(" There are %d IDS",number_ids);
//////////////////////////////////////////////////////
///// un-comment the two lines below to test STEP 2
//////////////////////////////////////////////////////
// number_codes = count_codes(fileName);
// printf(" There are %d codes ",number_codes);
//////////////////////////////////////////////////////
///// un-comment the line below to test STEP 3
//////////////////////////////////////////////////////
// printReport(fileName,number_ids, number_codes);
}
###########################################################################################
Step 1: Count the number of ids:
This step will implement the function count_ids. The prototype is included in the starter code. Do not change this prototype. The code that will successfully implement this function is similar (if not identical) to the code we did in lecture with the pizza tips problem. The only difference is that you are asked to provide this code in a function that returns the number of ids found. You are required to test the allocation to make sure it has been successfully allocated. In this function you are required to start the allocation process with memory that will hold 4 values (array_size = 4). It is recommended that you double the size of your block of memory each time you run out of space. You are given 2 data files that each have a different number of IDs and codes, as well as total data, to work with sales_person_data_1.csv sales_person_data_2.csv
.csv is in excel
#############################// sales_person_data_1.csv //##########################################
550 b 21.98 | ||
673 d 14.45 | ||
561 f 13.2 | ||
673 d 20.85 | ||
558 e 29.04 | ||
558 b 23.88 | ||
561 f 17.51 | ||
561 e 14.45 | ||
707 e 30.57 | ||
558 d 37.69 | ||
707 f 32.11 | ||
673 b 25.67 | ||
610 b 39.71 | ||
558 c 21.62 | ||
561 f 21.44 | ||
673 d 24.28 | ||
707 c 10.66 | ||
550 f 25.96 | ||
561 d 30.42 | ||
673 e 27.79 | ||
610 e 26.45 | ||
558 b 23.18 | ||
550 a 30.75 | ||
610 e 16.07 | ||
550 b 23.34 | ||
558 b 27.62 | ||
561 e 16.33 | ||
707 d 19.97 | ||
707 e 32.03 | ||
561 f 30.06 | ||
561 f 18.96 | ||
610 e 33.56 | ||
673 a 20.86 | ||
707 c 14.88 | ||
550 c 31.63 | ||
610 c 36.46 | ||
673 d 18.75 | ||
550 b 34.93 | ||
550 c 25.19 | ||
550 a 33.99 | ||
673 a 32.92 | ||
561 e 10.25 | ||
561 f 18.42 | ||
558 a 38.24 | ||
673 e 10.3 | ||
561 e 27.06 | ||
558 d 22.31 | ||
707 d 28.64 | ||
558 f 35.62 | ||
610 f 24.71 | ||
673 e 25.95 | ||
558 b 32.07 | ||
673 c 12.3 | ||
558 d 10.41 | ||
673 d 21.16 | ||
673 a 39 | ||
707 c 28.81 | ||
561 f 21.89 | ||
707 b 21.56 | ||
610 f 14.57 | ||
561 b 39.96 | ||
673 c 23.95 | ||
550 a 39.8 | ||
550 e 21.4 | ||
707 e 10.1 | ||
558 f 27.53 | ||
673 d 22.6 | ||
561 d 21.9 | ||
610 e 30.56 | ||
550 f 14.34 | ||
673 d 10.71 | ||
561 b 29.4 | ||
673 c 16.03 | ||
558 a 25.35 | ||
558 c 25.01 | ||
561 d 27.63 | ||
561 f 21.54 | ||
707 f 11.93 | ||
558 e 34.39 | ||
707 e 13.5 | ||
673 e 26.55 | ||
610 b 34.23 | ||
558 f 17.3 | ||
561 f 10.88 | ||
673 a 16.7 | ||
707 c 31.94 | ||
550 d 37.96 | ||
561 c 33.78 | ||
673 d 13.61 | ||
610 a 37.49 | ||
558 f 20.12 | ||
550 b 12.35 | ||
610 a 17.23 | ||
550 e 36.23 | ||
558 b 35.6 | ||
561 a 33.87 | ||
707 f 19.51 | ||
707 c 17.35 | ||
561 e 39.6 | ||
561 d 22.63 | ||
######################################// sales_person_data_2.csv //###################################
564 j 71.72 | ||
689 a 95.07 | ||
651 e 87.3 | ||
571 c 23.66 | ||
547 i 55.99 | ||
783 h 34.79 | ||
766 a 41.62 | ||
547 h 95.3 | ||
580 j 47.8 | ||
747 c 70.97 | ||
580 g 61.39 | ||
564 f 17.1 | ||
747 c 91.3 | ||
571 d 59.48 | ||
699 g 53.41 | ||
699 g 39.37 | ||
667 j 24.88 | ||
571 h 78.08 | ||
571 c 40.93 | ||
576 c 59.02 | ||
741 h 24.1 | ||
766 h 62.69 | ||
699 e 23.87 | ||
651 c 97.9 | ||
549 j 51.11 | ||
572 d 19.91 | ||
576 c 65.51 | ||
547 e 83.73 | ||
645 e 47.31 | ||
564 i 96.98 | ||
545 d 53.29 | ||
545 h 46.82 | ||
547 j 90.6 | ||
572 g 34.34 | ||
576 h 49.25 | ||
719 c 20.41 | ||
766 c 41.42 | ||
576 f 96.82 | ||
545 a 18.43 | ||
683 c 85.11 | ||
645 b 87.14 | ||
651 d 25.11 | ||
667 j 48.2 | ||
747 b 79.93 | ||
564 i 51 | ||
683 d 10.69 | ||
580 c 89.95 | ||
766 j 88.89 | ||
576 d 36.55 | ||
683 g 63.59 | ||
741 f 95.34 | ||
651 a 34.81 | ||
699 g 26.53 | ||
667 d 26.83 | ||
564 g 68.21 | ||
747 h 38.89 | ||
547 h 71.07 | ||
547 g 39.86 | ||
580 h 85.25 | ||
571 c 15.41 | ||
741 f 44.73 | ||
545 h 61.6 | ||
719 e 57.4 | ||
571 g 69.74 | ||
766 a 36.68 | ||
667 a 63.15 | ||
699 j 25.79 | ||
545 j 29.13 | ||
545 i 34.32 | ||
549 h 88.99 | ||
689 j 53.85 | ||
699 g 38.84 | ||
667 a 93.86 | ||
766 h 87.82 | ||
645 g 85.77 | ||
549 e 11.53 | ||
747 j 14.37 | ||
683 a 47.82 | ||
766 g 33.26 | ||
651 c 97.21 | ||
580 g 28.96 | ||
699 b 66.98 | ||
549 h 69.78 | ||
683 c 98.36 | ||
549 j 53.61 | ||
719 i 75.88 | ||
572 h 83.21 | ||
667 a 94.4 | ||
547 g 68.35 | ||
549 h 44.32 | ||
571 h 21.16 | ||
683 h 20.35 | ||
683 j 90.94 | ||
747 j 40.12 | ||
699 h 74.56 | ||
683 i 84.66 | ||
580 b 44.27 | ||
572 f 87.41 | ||
645 e 49.14 | ||
580 j 55.7 | ||
783 f 86.4 | ||
651 a 67.72 | ||
651 j 24.96 | ||
576 j 53.65 | ||
576 c 42.58 | ||
547 e 47.06 | ||
683 g 76.79 | ||
766 h 61.67 | ||
580 b 39.53 | ||
683 b 56.34 | ||
783 g 37.98 | ||
545 h 25.01 | ||
783 c 86.2 | ||
667 c 10.67 | ||
576 c 82.19 | ||
564 b 24.96 | ||
651 f 53.51 | ||
547 j 27.85 | ||
645 d 25.29 | ||
766 a 24.18 | ||
651 i 56.25 | ||
576 h 64.52 | ||
747 h 45.01 | ||
719 c 69.18 | ||
580 a 90.52 | ||
689 d 61.49 | ||
576 e 90.7 | ||
564 a 23.73 | ||
571 e 93.29 | ||
545 h 40.19 | ||
547 h 88.86 | ||
580 g 74.18 | ||
766 i 70.95 | ||
651 j 24.18 | ||
719 h 38.24 | ||
667 f 97.94 | ||
576 g 99.33 | ||
766 i 25.52 | ||
667 d 78.04 | ||
572 h 64.09 | ||
651 e 19.13 | ||
571 e 69.16 | ||
689 b 21.72 | ||
549 i 86.99 | ||
571 a 59.73 | ||
719 i 84.53 | ||
689 c 87.53 | ||
741 b 82.35 | ||
572 e 12.51 | ||
564 g 34.84 | ||
747 d 41.05 | ||
651 d 62.96 | ||
766 c 51.15 | ||
783 d 57.02 | ||
545 h 53.32 | ||
564 i 44.31 | ||
572 d 57.22 | ||
576 c 36.5 | ||
545 b 15.52 | ||
783 h 48.77 | ||
783 j 15.68 | ||
689 c 96.62 | ||
580 c 99.9 | ||
741 b 43.24 | ||
547 h 68.34 | ||
689 h 63.42 | ||
576 i 49.94 | ||
719 b 45.21 | ||
564 a 65.28 | ||
699 d 44.82 | ||
564 j 60.23 | ||
667 f 99.12 | ||
719 e 37.67 | ||
719 e 68.09 | ||
547 i 80.08 | ||
545 h 47.39 | ||
571 e 46.33 | ||
673 d 56.66 | ||
719 e 30.39 | ||
719 i 68.97 | ||
580 d 12.38 | ||
571 j 46.03 | ||
673 f 77.11 | ||
564 f 78.34 | ||
747 j 52.97 | ||
667 a 86.41 | ||
580 h 82.05 | ||
576 e 16.42 | ||
719 h 91.75 | ||
719 e 64.25 | ||
683 b 38.63 | ||
572 d 57.72 | ||
667 b 66.73 | ||
699 b 64.2 | ||
576 h 17.96 | ||
580 e 58.94 | ||
547 j 81.1 | ||
564 c 96.98 | ||
545 a 19.84 | ||
741 b 10.65 | ||
545 g 36.04 | ||
719 f 97.25 | ||
576 e 83.72 | ||
571 e 72.87 | ||
719 h 27.61 | ||
547 e 63.85 | ||
699 a 15.2 | ||
683 e 35.89 | ||
741 e 27.97 | ||
741 e 87.83 | ||
683 j 44.58 | ||
576 h 30.44 | ||
651 g 31.46 | ||
699 a 58.14 | ||
549 c 88.41 | ||
667 g 82.41 | ||
783 e 48.27 | ||
766 f 77.93 | ||
576 a 73.09 | ||
549 g 16.64 | ||
651 e 99.5 | ||
580 b 47.38 | ||
576 h 76.37 | ||
547 e 28.38 | ||
651 g 26.25 | ||
651 j 67.58 | ||
571 e 70.73 | ||
547 b 61.56 | ||
549 i 68.13 | ||
576 c 39.14 | ||
576 d 37.14 | ||
651 b 20.83 | ||
580 j 48.95 | ||
549 h 95.6 | ||
572 d 33.85 | ||
576 c 43.99 | ||
545 f 83.34 | ||
580 a 37.04 | ||
571 e 68.11 | ||
549 g 54.22 | ||
576 d 15.97 | ||
564 c 92.6 | ||
667 a 78.83 | ||
545 c 44.08 | ||
719 b 89.17 | ||
572 j 98.61 | ||
667 h 15.83 | ||
576 i 47.26 | ||
547 i 40.68 | ||
571 e 40.78 | ||
673 d 79.72 | ||
766 g 72.34 | ||
651 j 58.56 | ||
651 j 13.82 | ||
689 a 39.01 | ||
689 h 17.84 | ||
572 i 27.24 | ||
783 b 32.85 | ||
683 a 37.17 | ||
683 i 73.25 | ||
667 h 62.62 | ||
699 g 11.4 | ||
545 h 38.93 | ||
741 c 62.86 | ||
572 e 42.44 | ||
547 i 64.27 | ||
683 i 87.39 | ||
651 j 12.42 | ||
766 a 68.93 | ||
580 f 89.91 | ||
580 a 47.67 | ||
673 a 81.66 | ||
572 e 62.27 | ||
699 i 32.48 | ||
667 c 52.74 | ||
580 h 74.37 | ||
667 a 32.47 | ||
545 e 55.48 | ||
673 c 47.94 | ||
651 c 56.57 | ||
545 i 35.04 | ||
783 a 84.14 | ||
699 b 85.85 | ||
645 g 83.34 | ||
547 d 22.3 | ||
580 i 13.68 | ||
683 h 77.55 | ||
683 j 20.42 | ||
545 c 78.75 | ||
689 j 48.06 | ||
572 j 76.5 | ||
549 j 88.07 | ||
673 g 73.91 | ||
651 f 21.13 | ||
572 j 53.25 | ||
741 j 15.18 | ||
564 c 68.8 | ||
651 c 11.68 | ||
545 c 27.37 | ||
571 e 26.39 | ||
580 j 21.05 | ||
545 j 72.26 | ||
667 h 81.7 | ||
572 j 37.5 | ||
545 c 96.24 | ||
564 a 38.72 | ||
719 b 51.39 | ||
547 g 31.7 | ||
719 f 66.5 | ||
576 b 30.87 | ||
576 g 94.98 | ||
766 j 25.84 | ||
673 j 10.99 | ||
564 g 53.17 | ||
645 a 57.21 | ||
576 j 93.41 | ||
#################################################################################################
Because you are using a single dimensional array in this part of the program, you can use array notation to assign values to the allocated memory. You should also free the memory before you leave the function. The pseudo code for the general process of counting unique ids is given below. It should be noted that only variables that are crucial to the process are named. This are other variables you will need such as pointers and counter. Figure1
Step 2: Count the number of codes:
This step is very similar to the first with the exception that the data type is char and not int. The prototype is included in the starter code. Do not change this prototype. The logic of the function is exactly the same as in the function of step 1, with this exception.
Step 3: Read in the data for analysis:
This step requires that you pass the function the correct number of ids and codes as well as the filename. It will then open the file and load the id array, the code array and the sales array to be used to create a report on the totals for each id-code combination. For this step, you will be implementing allocated memory for a 2D array as well as as the two single dimension arrays for the ids and codes. For the 2D array you will need to use pointer math to access individual elements. You cannot use 2D array notation as you can for the single dimensional array. To accomplish this data analysis, you can use the same pattern as laid out in the pseudo code with a few exceptions. Because you know exactly how many ids and codes you have, you can allocate the correct amount immediately and you can eliminate lines 13 through 16 in the pseudo code. However you will need to find the index for both the id and the code so lines 6 - 12 in the pseudocode will have to be repeated for finding the code index (lift it from the count_codes function). Once you have found both the code and the id indices, you can update the correct element of the 2D sales array using pointer math. Once the arrays are fully loaded, you will have to make a report as shown below. You will need to use pointer math to
print out the sales values. The two report shown are for the two data files you have been provided with. The table for the data file : sales_person_Data_1.csv
Figure 2
The table for the data file : sales_person_Data_2.csv
Figure 3
Check List ?PLEASE HELP! this is a very difficult problem that a lot of my class has been struggleing with anyway you can help is Immensly appreciated. Thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
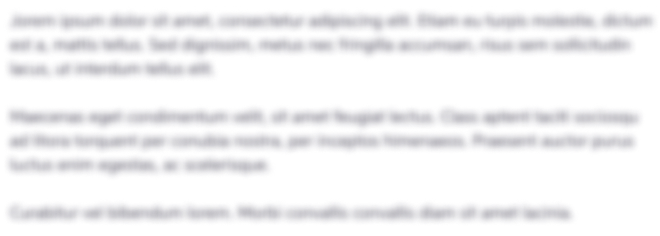
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started