Question
This week, you will update your week 6 program and implement a subclass to handle the calculations. The local power company has decided that in
This week, you will update your week 6 program and implement a subclass to handle the calculations.
The local power company has decided that in order to better serve their customers and encourage power savings, they will charge their customers based upon their power consumption each month. In doing so, they have asked your software company to make a simple online application that their customers can run in order to get an idea how much their bills may be for the month..
Write a program that will ask for the customers last and current meter reading in Kilowatt Hours (KwHs). Determine the amount of usage for the month and calculate a subtotal (before tax) and a total amount due (tax included) using the following constraints.
Constraints
Rate A: For 500 KwHs or less = $0.0809 / KwH
Rate B: For 501 to 900 KwHs = $0.091 / KwH
Rate C: For greater than 900 KwHs = $0.109 / KwH
Utilities Tax is 3.46% regardless of usage.
Requirements
Create a separate (external to the main class) subclass MyUtility()
Rate and tax variables should be private to the subclass
Use a constructor to initialize the default reading values to 0
Use another constructor to set the reading values
Use set and get methods in MyUtility() to determine the usage, rate, subtotal, tax, and final bill
Format all output as follows:
o Usage to 1 decimal place, KhWs o Rate to 4 decimal places, monetary o Subtotal to 2 decimal places, monetary o Taxes to 2 decimal places, monetary o Total to 2 decimal places, monetary
Implement a loop to return and enter new values (run the program again) if the user wishes to Hints
Set methods can do all the computation
Set methods can call other set methods
The type of loop can be of your choosing
Make sure you use Java coding conventions
Expected Output
Below is a sample run with three iterations. User input is in red.
Welcome to the City Power Bill Calculator! Please enter your previous meter reading: 750 Please enter your current meter reading: 1250
Your usage was: 500.0 KwHs Your rate was: $0.0809/KwH Your subtotal is: $40.45 Taxes are: $1.40 Your total bill this month is: $41.85
Would you like to calculate a new usage? (Y for Yes, N to exit): y
Please enter your previous meter reading: 750 Please enter your current meter reading: 1350.63
Your usage was: 600.6 KwHs Your rate was: $0.0910/KwH Your subtotal is: $54.66 Taxes are: $1.89 Your total bill this month is: $56.55
Would you like to calculate a new usage? (Y for Yes, N to exit): y
Please enter your previous meter reading: 750.39
Please enter your current meter reading: 1655.37
Your usage was: 905.0 KwHs Your rate was: $0.1090/KwH Your subtotal is: $98.64 Taxes are: $3.41 Your total bill this month is: $102.06
Would you like to calculate a new usage? (Y for Yes, N to exit): n
Thank you for using this program. Goodbye!
HERE IS MY CODE:
//Imports import java.util.Scanner; //Begin Class AS public class AS { //Begin Main Method public static void main(String[] args) { String choice; // get readings from customer do { System.out.println("Welcome to the City Power Bill Calculator!"); System.out.print("Please enter your previous meter reading:"); Scanner input = new Scanner(System.in); double Previous = input.nextDouble(); System.out.print("Please enter your current reading:"); double Current = input.nextDouble(); // call 1st method to determine usage System.out.printf("Your usage was %.1f KhWs ", getusage(Current, Previous)); // assign usage determination from previous method and determine rate double usage = getusage(Current, Previous); // determine rate final double rate; if (usage <= 500) { rate = 0.0809; } else if (usage > 500 && usage <= 900) { rate = 0.091; } else { rate = 0.109; } // output rate System.out.printf("Your rate was $%.4f/KhWs ", rate); // get subtotal from 2nd method System.out.printf("Your subtotal is $%.2f ", getsubtotal(usage, rate)); // assign subtotal and tax percent to variable double subtotal = getsubtotal(usage, rate); double taxperc = 0.0346; // determine tax from method System.out.printf("Your tax was $%.2f ", gettax(subtotal, taxperc)); //assign tax and total to variable double tax = gettax(subtotal, taxperc); double total = (subtotal + tax); // determine total for bill System.out.printf("Your total bill this month is $%.2f ", total); System.out.println("Would you like to run again? y or n"); choice = input.next(); }while (choice.equalsIgnoreCase("y")); System.out.println("Thank you for using the program! Goodbye");
} //End Main Method /** * method to determine usage * @param c * @param p * @return */ private static double getusage(double c, double p) { double usage; usage = (c - p); return usage; }
/** * method to determine subtotal * @param u * @param r * @return */ private static double getsubtotal(double u, double r) { double subtotal; subtotal = (u * r); return subtotal; } /** * method to determine tax * @param s * @param t * @return */ static final double gettax(double s, double t) { double tax; tax = (s * t); return tax; } //End Main Method } //End Class AS
**************************************************************************************************
//Begin Subclass MyUtility public class MyUtility {
private double rate;
private double tax;
private double usage, subtotal, finalBill;
public MyUtility() {
usage = tax = subtotal = finalBill = rate = 0;
}
/** * * @param rate * * @param tax * */ public MyUtility(double current, double previous) {
usage = current - previous;
setRate(usage);
}
public double getRate() {
return rate;
}
private void setRate(double usage) {
if (usage <= 500) {
rate = 0.0809;
} else if (usage > 500 && usage <= 900) {
rate = 0.091;
} else {
rate = 0.109;
}
setSubtotal();
}
public double getTax() {
return tax;
}
public void setTax() {
double taxperc = 0.0346;
tax = subtotal * taxperc;
}
public double getUsage() {
return usage;
}
public void setUsage(double usage) {
this.usage = usage;
}
public double getSubtotal() {
return subtotal;
}
public void setSubtotal() {
subtotal = usage * rate;
setFinalBill();
}
public double getFinalBill() {
return finalBill;
}
public void setFinalBill() {
finalBill = subtotal + tax;
} } //End Subclass MyUtility
***************************************************************************
My teachers comments:
Hi Alexis, Requirements of the assignment and whether you met them. You updated your week 6 program to utilize a subclass to handle all meter calculations. You wrote a program that output a bill to companies based on the amount of power they used and with a specific rate based on the power used. You asked the user for their last month meter reading and the current month meter reading. This included a loop which continuously asked if the user desired. (yes) 10/10 You created a separate subclass named MyUtility(). (yes) 10/10 You created private member variables for rate and tax in your MyUtility class. (yes) 10/10 You created/modified the default constructor to initialize the default reading values to 0. (yes) 10/10 You created an overloaded constructor to set the reading values. (yes) 10/10 You created and utilized get/set methods in your MyUtility class to determine the usage, rate, subtotal, tax, and final bill calculations (no, never invoked these from the main method) 0/30 The output was formatted according to requirements. (not correct, basically submitted the week 6 assignment with a separate class, but, never used the MyUtility class) 0/10 Your program compiled and executed correctly. There were no logic errors. You also provided comments at the top of your file and throughout the source code. (see above) 0/10 Please email me if you have any questions.
Can someone help me with this. I do not understand what is wrong.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
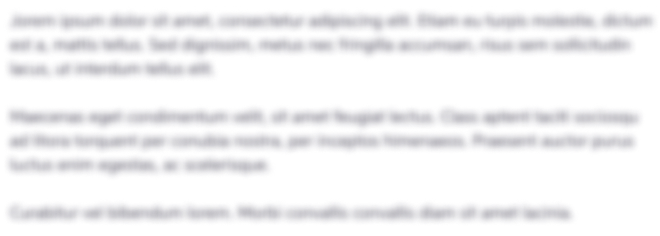
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started