Question
we have to put code for isEmpty, size, and toString under ArrayStack.java, as well as creating a new method that will display all of the
we have to put code for isEmpty, size, and toString under ArrayStack.java, as well as creating a new method that will display all of the elements of the stack in a straight line. They should be able to handle any exceptions. Then, create a driver (ArrayTest.java) to create a menu that will push, pop, peek, display, and exit successfully.
EmptyCollectionException.java
package jsjf.exceptions;
/** * Represents the situation in which a collection is empty. * * @author Java Foundations * @version 4.0 */ public class EmptyCollectionException extends RuntimeException { /** * Sets up this exception with an appropriate message. * @param collection the name of the collection */ public EmptyCollectionException(String collection) { super("The " + collection + " is empty."); } }
ArrayStack.java
package jsjf;
import jsjf.exceptions.*; import java.util.Arrays;
/** * An array implementation of a stack in which the bottom of the * stack is fixed at index 0. * * @author Java Foundations * @version 4.0 */ public class ArrayStack
private int top; private T[] stack;
/** * Creates an empty stack using the default capacity. */ public ArrayStack() { this(DEFAULT_CAPACITY); }
/** * Creates an empty stack using the specified capacity. * @param initialCapacity the initial size of the array */ public ArrayStack(int initialCapacity) { top = 0; stack = (T[])(new Object[initialCapacity]); }
/** * Adds the specified element to the top of this stack, expanding * the capacity of the array if necessary. * @param element generic element to be pushed onto stack */ public void push(T element) { if (size() == stack.length) expandCapacity();
stack[top] = element; top++; }
/** * Creates a new array to store the contents of this stack with * twice the capacity of the old one. */ private void expandCapacity() { stack = Arrays.copyOf(stack, stack.length * 2); }
/** * Removes the element at the top of this stack and returns a * reference to it. * @return element removed from top of stack * @throws EmptyCollectionException if stack is empty */ public T pop() throws EmptyCollectionException { if (isEmpty()) throw new EmptyCollectionException("stack");
top--; T result = stack[top]; stack[top] = null;
return result; }
/** * Returns a reference to the element at the top of this stack. * The element is not removed from the stack. * @return element on top of stack * @throws EmptyCollectionException if stack is empty */ public T peek() throws EmptyCollectionException { if (isEmpty()) throw new EmptyCollectionException("stack");
return stack[top-1]; }
/** * Returns true if this stack is empty and false otherwise. * @return true if this stack is empty */ public boolean isEmpty() { // To be completed as a Programming Project return true; // temp }
/** * Returns the number of elements in this stack. * @return the number of elements in the stack */ public int size() { // To be completed as a Programming Project return 0; // temp }
/** * Returns a string representation of this stack. * @return a string representation of the stack */ public String toString() { // To be completed as a Programming Project return ""; // temp } } StackADT.java
package jsjf;
/** * Defines the interface to a stack collection. * * @author Java Foundations * @version 4.0 */ public interface StackADT
/** * Removes and returns the top element from this stack. * @return the element removed from the stack */ public T pop();
/** * Returns without removing the top element of this stack. * @return the element on top of the stack */ public T peek();
/** * Returns true if this stack contains no elements. * @return true if the stack is empty */ public boolean isEmpty();
/** * Returns the number of elements in this stack. * @return the number of elements in the stack */ public int size();
/** * Returns a string representation of this stack. * @return a string representation of the stack */ public String toString(); }
ArrayTest.java (What i have so far)
package jsjf;
import java.util.Scanner;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * * @author 18569 */ public class ArrayTest { public static void main(String[] args) { Scanner input = new Scanner(System.in); ArrayStack
Step by Step Solution
There are 3 Steps involved in it
Step: 1
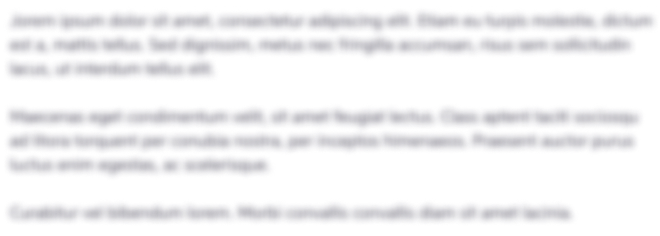
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started