Question
Write a French/English dictionary lookup program. Read a list of pairs of English and French words from a file specified by the user. English/French words
Write a French/English dictionary lookup program. Read a list of pairs of English and French words from a file specified by the user. English/French words should be exact matches (don't try to find partial matches). Use the supplied EnglishFrenchDictionary.java class as your main class. Fill in the missing code in the DictionaryTable.java class to read the input file and perform the searches. Add code to the DictionaryTable read() method to:
read pairs of lines (English word is on the first line, and the corresponding French word is on the second line), and for each pair of lines:
create an Item object for the English word and French word to store in the byEnglish array list
create an Item object for the French word and corresponding English word to store in the byFrench array list.
After reading all the words, sort the byEnglish and byFrench array lists so we can use binary search on them.
Add code to the DictionaryTables findFrench() method to:
Create an Item object with the specified English word and null for the French word (because we dont know it yet)
Use the Collections.binarySearch() method to search for the matching Item in the byEnglish array list
If a matching Item is found, return the items value which is the corresponding French word (hint: use getValue()).
Add similar code to the DictionaryTables findEndlish() method to:
Create an Item object with the specified French word and null for the English word (because we dont know it yet)
Use the Collections.binarySearch() method to search for the matching Item in the byFrench array list
If a matching Item is found, return the items value which is the corresponding English word (hint: use getValue()).
Two files have been supplied for testing your program: shortdictionary.txt and longdictionary.txt. shortdictionary.txt has just a few words in it for quick testing, and longdictionary.txt has over 500 entries for more extensive testing. The files have the format of an English word on one line, followed by the French word on the next line:
eight
huit
Amazon
amazone
april
avril
Arctic
arctique
British
britannique
. . .
A sample run of the program:
Enter the name of the dictionary file:
shortdictionary.txt
Lookup by E)nglish word, F)rench word, Q)uit?
F
Enter French word:
huit
English word: eight
Lookup by E)nglish word, F)rench word, Q)uit?
E
Enter English word:
eight
French word: huit
Lookup by E)nglish word, F)rench word, Q)uit?
Java Files Needed:
DictionaryTable:
package Homework6; import java.util.ArrayList; import java.util.Collections; import java.util.Scanner;
/** A table for lookups and reverse lookups */ public class DictionaryTable { private ArrayList
/** Constructs a DictionaryTable object. */ public DictionaryTable() { byEnglish = new ArrayList
// When finished, be sure to sort the byEnglish and byFrench lists!
} /** Looks up an item in the table. @param english the key to find @return the value with the given key, or "Not Found" if no such item was found. */ public String findFrench(String english) { // TODO: Search the byEnglish array list for an Item with a matching english word, // and returns its value.
// This is the default result: return "Not Found"; }
/** Looks up an item in the table. @param french the value to find @return the key with the given value, or "Not Found" if no such item was found. */ public String findEnglish(String french) { // TODO: Search the byFrench array list for an Item with a matching french word, // and returns its value.
// This is the default result: return "Not Found"; } }
EnglishFrenchDictionary:
package Homework6; import java.io.IOException; import java.io.FileReader; import java.util.Scanner;
/* The input file has the format of English word on one line * followed by French word on the next line. The pairs continue * until the end of the file. */ public class EnglishFrenchDictionary { public static void main(String[] args) throws IOException { Scanner in = new Scanner(System.in); System.out.println("Enter the name of the dictionary file: "); String fileName = in.nextLine(); DictionaryTable table = new DictionaryTable(); FileReader reader = new FileReader(fileName); table.read(new Scanner(reader)); boolean more = true; while (more) { System.out.println("Lookup by E)nglish word, F)rench word, Q)uit?"); String cmd = in.nextLine(); if (cmd.equalsIgnoreCase("Q")) more = false; else if (cmd.equalsIgnoreCase("E")) { System.out.println("Enter English word:"); String n = in.nextLine(); System.out.println("French word: " + table.findFrench(n)); } else if (cmd.equalsIgnoreCase("F")) { System.out.println("Enter French word:"); String n = in.nextLine(); System.out.println("English word: " + table.findEnglish(n)); } } } }
Item:
package Homework6; /** An item with a key and a value. */ public class Item implements Comparable
/** Constructs an Item object. @param k the key string @param v the value of the item */ public Item(String k, String v) { key = k; value = v; } /** Gets the key. @return the key */ public String getKey() { return key; } /** Gets the value. @return the value */ public String getValue() { return value; }
public int compareTo(Item otherObject) { Item other = (Item) otherObject; return key.compareTo(other.key); } }
Two Files Associated With Program:
longdictionary:
eight huit Amazon amazone april avril Arctic arctique British britannique Cinderella Cendrillon Denmark Danemark Englishman Anglais Germany Allemagne India Inde Ireland Irlande Italy Italie January janvier July juillet June juin London Londres Mexico Mexique Miss demoiselle Mister monsieur North nord Pacific Pacifique Saturday samedi September septembre Spain Espagne Sunday dimanche Thursday jeudi Tuesday mardi Wednesday mercredi abdicate abdiquer aberration erreur ablaze ardant abscissa abscisse absolve absoudre abstinence abstention accept accepter acceptance abord accidental accidentel accompany accompagner accuracy exactitude acrobat acrobate actor acteur actress actrice adjective adjectif affluence richesse agoraphobia agoraphobie allergy allergie analysis analyse analyst analyste ancient antique and et angel ange anguish angoisse ankle cheville anniversary anniversaire annotation note announce annoncer annual annuel antelope antilope antibiotic antibiotique apiary rucher apple pomme apricot abricot architect architecte astronaut astronaute atom atome atomic atomique attractive affriolant authorize autoriser aware conscient away loin awkward maladroit bacon lard baker boulanger bakery boulangerie banker banquier barley orge beard barbe bee abeille believe croire beverage boisson bird oiseau blanket couverture boat barque bronze airain bucket seau cabbage chou cabinet armoire candidate candidat candle bougie carpet tapis caution alerter cautious prudent ceiling plafond cellar cave cheese fromage cherry cerise chocolate chocolat cider cidre cigar cigare coal charbon community commune commute aiguiller companion accompagnateur competition concours complexion teint conceited frivole conceive concevoir conflict conflit connection ligue costume complet cougar puma countryside campagne courage abattage cow vache crawl ramper dad papa daughter fille deaf sourd deal dispenser death mort debt dette deep profond deer cerf delicious savoureux deliver fournir dentist dentiste dictionary dictionnaire difficult difficile dog chien dolphin dauphin door porte downstairs dessous drip goutte driver chauffeur eagle aigle election choix enemy ennemi engrave graver expect attendre fabric textile family famille feast banqueter feather plume fight batailler filter filtrer five cinq fog brouillard forest bois forget oublier forgive excuser fork fourchette forty quarante four quatre fourteen quatorze fox renard fresh frais garden jardin glove gant golf golfe guest convive guilty coupable handkerchief mouchoir handlebars gouvernail handsome beau heavy lourd home domicile house maison identical identique imposter charlatan include contenir inconvenient difficile incredible invraisemblable index liste infant enfant integer entier interrupt interrompre interval intervalle interview entrevue jacket veste jewel bijou kettle chaudron keyboard clavier kingdom royaume kitchen cuisine laboratory laboratoire lake lac large grand lawyer avocat learn apprendre lemon citron lettuce laitue life vie loaf pain magician sorcier management administration manure engrais massage masser massive massif matrimony mariage mattress matelas mild doux mint menthe money argent month mois moon lune morning matin mountain mont music musique name nom nephew neveu nine neuf nitrogen azote no non north nord odor odeur oil huile open ouvrir orchestra orchestre ordinary ordinaire owl chouette pain douleur paradox paradoxe parrot perroquet patrolman gendarme peanut arachide pear poire pencil crayon perfect accompli permanent continuel permission autorisation physician docteur plastic plastique pocket poche policy politique polite courtois prepay affranchir prescription recette previously auparavant price prix printer imprimeur proof preuve proud altier pull tirer purple pourpre quickly rapidement quietly paisiblement quilt coudre radish radis radius rayon rapid prompt raspberry framboise raw brut reach aboutir recipe recette recommend recommander referee arbitre regal royal rent loyer request demander rescue sauver reverse inverse rhythm rythme ribbon ruban rigid raide rinse rincer riot bagarre river fleuve rope corde salary appointements salmon saumon scar balafre scissors ciseaux screwdriver tournevis search recherche season saison secret arcane sell vendre send adresser seven sept shallow superficiel shirt chemise shore bord sick malade silent silencieux sixteen seize sixty soixante sky ciel slow lent small petit soldier soldat sort acabit space espace sparrow moineau speak parler split fendre springtime printemps spy espion stain salir standard drapeau statute statut steak bifteck steel acier stomach estomac stone pierre strawberry fraise street rue strong fort sturdy robuste succeed abouter sugar sucre swan cygne swine cochon syrup sirop talent aptitude ten dix thirteen treize thirty trente three trois threshold seuil tickle chatouiller tiger tigre tile carreau tiny miniscule today aujourd'hui tomato tomate tomorrow demain tooth dent topical actuel towel serviette treasure cassette tree arbre trip voyage tuna thon turkey dindon twelve douze twenty vingt twin jumeau uncle oncle understand comprendre unhappy malheureux unimportant mineur unintelligible abracadabrant universe univers unkind maussade unlock ouvrir upstairs dessus vehicle bagnole verb verbe very bien vinegar vinaigre visitor visiteur vocabulary dictionnaire voice voix vowel voyelle waist taille wash laver we nous weapon arme weather temps week semaine whale baleine wheat froment wheel roue wine vin wire fil wireless radio wolf loup woman femme wonderful merveilleux wood bois word mot worker ouvrier world monde writer auteur yellow jaune yes oui
shortdictionary:
twelve douze twenty vingt ten dix thirteen treize thirty trente three trois four quatre fourteen quatorze five cinq sixteen seize sixty soixante seven sept nine neuf eight huit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
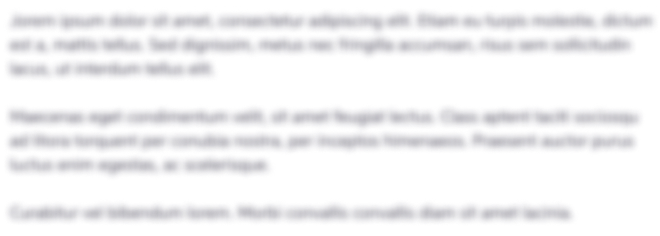
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started