Question
Write a program that is composed of one source file named Lab3b.cpp . The program instantiates and uses instances of a class with non-default constructors.
Write a program that is composed of one source file named Lab3b.cpp. The program instantiates and uses instances of a class with non-default constructors. The program must be written in accordance to the following plan:
Description
You should include a program description at the top of the main program according to the template. You may simply use this sentence, "This program instantiates and uses instances of a class with non-default constructors."
Write A Class
Declare a class named NutritionData that contains these private fields
foodName (string)
servingSize (int)
calFromCarb (double)
calFromFat (double)
calFromProtein (double)
totalCalories (double)
Use the data types in parentheses for the fields.
Each field should have a comment documenting what it is for.
Add the default constructor.
Add a constructor that accepts these data: food name (string), serving size (int), cal from carb (double), cal from fat (double) and cal from protein (double).
Add mutator member functions to set the fields. One member function for each field except totalCalories. Each member function should begin with the word 'set' followed by the field name with the first letter changed to uppercase. Each member function should have a comment above the declaration describing its purpose.
Add accessor member functions to return the fields. One member function for each field. Each member function should begin with the word 'get' followed by the field name with the first letter changed to uppercase. Each member function should have a comment above the declaration describing its purpose.
Write the definition for the default constructor. The default constructor initializes the fields so that the food name is an empty string and all other fields are 0.0.
Write the definition for the other constructor which sets all the fields based on the parameters received. It sets the total calories field based on the given three calories.
Write the definition for every member function. When one of the mutator functions for the calories is set, it should also update the totalCalories.
Write the main function as follows:
Define and initialize an array named nutritionData using the following data:
Apples raw, 110, 50.6, 1.2, 1.0
Bananas, 225, 186, 6.2, 8.2
Bread pita whole wheat, 64, 134, 14, 22.6
Broccoli raw, 91, 21.9, 2.8, 6.3
Carrots raw, 128, 46.6, 2.6, 3.3
Use a range-based for loop to print the nutrition data for all foods to the screen using the accessor functions.
Test The Program
The output should look exactly as follows:
Food Name: Apples raw Serving Size: 110 g Calories Per Serving: 52.8 Calories From Carb: 50.6 Calories From Fat: 1.2 Calories From Protein: 1 Food Name: Bananas Serving Size: 225 g Calories Per Serving: 200.4 Calories From Carb: 186 Calories From Fat: 6.2 Calories From Protein: 8.2 Food Name: Bread pita whole wheat Serving Size: 64 g Calories Per Serving: 170.6 Calories From Carb: 134 Calories From Fat: 14 Calories From Protein: 22.6 Food Name: Broccoli raw Serving Size: 91 g Calories Per Serving: 31 Calories From Carb: 21.9 Calories From Fat: 2.8 Calories From Protein: 6.3 Food Name: Carrots raw Serving Size: 128 g Calories Per Serving: 52.5 Calories From Carb: 46.6 Calories From Fat: 2.6 Calories From Protein: 3.3
Submit Lab3b.cpp
Step by Step Solution
There are 3 Steps involved in it
Step: 1
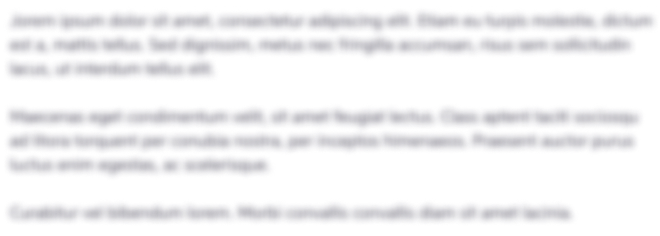
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started