Question
Write a Python program to manage the students enrolled in a unit. The program will display a menu that allows the user to perform different
Write a Python program to manage the students enrolled in a unit. The program will display a menu that allows the user to perform different operations repeatedly. The menu must include the following items:
1. Add the details of a student to the system, including the student number, the student's surname, the student's given name, and the unit mark
2. Search for students based on the student number or student name and display the details of the matching students. The name search must be case-insensitive and must allow for a partial match, for example, the search key "john" would be matched by "John Smith" and "Elton Johns".
3. Display the names of the students with a given unit grade(HD, D, C, P and N). Use Murdoch University's grade system to convert marks to grades.
4. delete a student's record from the system
5. quit: to quit the program
You should use a list to store the information about all students. In the list, each list item is a dictionary containing one student record.
Question 2 : Load student records from the file and saves them to the file
Revised your solution to Question 1 above to add additional features: when the program starts, it first loads the student records from the file students.csv inside the current directory. In addition, the user can load additional student records from a CSV file and save the student records maintained by the system to a CSV file. Correspondingly, two more menu items should be added to the menu:
6. Load student records from a CSV file. The program should prompt the user to provide a file path. The student records from the file are added to those student records already in the memory. Check for duplicates of student records (ie, records with the same id): in case of a duplicate, the one from the file should be ignored.
7. Save the student records in the current system to a CSV file. The program should prompt the user to enter a file path. If the file exists, warn the user and give the user the option of either
changing the file name, overwriting the file, or cancelling the operation.
You may use Python's built-in module csv to handle CSV files.
For your convenience, I have created a file containing the data of 150 students. The information inside the file is completely fictitious and random. You are required to test your program using multiple and different files, including this file.
Question 3 : Display grade distribution in charts
Revised your solution to Question 2 above by adding the following additional features:
- to increase the system efficiency, replace the list used to store the student records with an ndarray from the NumPy module..
- to display the grade distribution using a pie chart. The pie chart must have a title and labels for each grade.
- to display a bar chart showing the percentage of students in each of the following marks ranges: 0-29, 30-39, 40-49, 50-59, 60-69, 70- 79, 80-89 and 90-100. The bar chart must have a title and labels for x-axis and y-axis.
To incorporate the above features, add two additional menu items to the program menu:
8. display grade distribution
9. display marks distribution
You may use Python modules numpy and matplotlib for this question.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Below is a Python program that implements the features described in all three parts of your question This program uses a menu to manage student records loadsave records fromto CSV files and display grade and marks distributions using NumPy and Matplotlib First make sure you have the NumPy and Matplotlib libraries installed You can install them using pip if you havent already pip install numpy matplotlib Now heres the Python program import csv import numpy as np import matplotlibpyplot as plt students Load student records from the CSV file def loadstudentrecordsfilename try with openfilename moder as file reader csvDictReaderfile for row in reader studentsappendrow printStudent records loaded from the file except FileNotFoundError printFile not found Save student records to a CSV file def savestudentrecordsfilename try with openfilename modew newline as file fieldnames studentnumber surname givenname unitmark writer csvDictWriterfile fieldnamesfieldnames writerwriteheader for student in students writerwriterowstudent printStudent records saved to the file except PermissionError printPermission denied to save the file Add a student record ...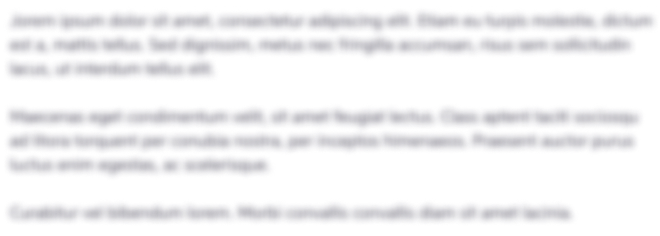
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started