Question
Write testmain. CPP to verify that functions in arrayFunctions are valid. There is no need to open the folder in testmain. CPP, you should use
Write testmain. CPP to verify that functions in arrayFunctions are valid. There is no need to open the folder in testmain. CPP, you should use string as sample data. I've already written arrayFunctions. The CPP and main. CPP, but I don't know how to verify that arrayFunction works without opening the folder.Requirements are:
Write a C++ program that reads in an unknown number of integer arrays from a file. Your program will need to store each array temporaily in a dynamically allocated array for processing, and display, to the screen, the largest, smallest, average, and median values of each array. After each array is processed, your program should free the memory allocated for that array. In addition to the processing done on each array, your program needs to display the overall largest and smallest values of all arrays (this can be done with out a function within the main function).
VLA's (Variable Length Arrays) are restricted from use. If your program uses a VLA, your solution will loose 25% of the points.
Memory leaks will be monitored closely. If your solution leaks memory, your solution will loose 25% of the points.
Your program will need to open a file named "arrays.txt" to obtain the arrays.
format of arrays.txt:
numberOfValuesvalue1 value2 ...valueN numberOfValues value1 value2 ...valueN arrays.txt (sample provided below)
5 1 2 3 4 5 4 10 2 32 14 3 23 41 2
Here is my code:
proj2-arrayFunctions.h
#ifndef PROJ2_ARRAYFUNCTIONS_H #define PROJ2_ARRAYFUNCTIONS_H
void bubbleSort(int *array, int size); int largestValue(int *array, int size); // used for largest in single array int smallestValue(int *array, int size); // used for smallest in single array double averageValue(int *array, int size); int medianValue(int *array, int size); //choose the smaller value if an evenly sized array
#endif
proj2-arrayFunctions.cpp
using namespace std;
/** * bubbleSort the data * * the function sort data using bubble sort * * Parameter * * array is the pointer array that store data * size is the size of the array * * output * return none * reference parameter: array * stream none */ void bubbleSort(int *array, int size){
// use first for loop to set the i for(int i = 0; i < size - 1; i++) { // use this loop to set the j for(int j = 0; j < size - 1 - i; j++) { // to check if we find the smaller number if(array[j] > array[j+1]) { // found a smaller value, switch them swap (array[j], array[j+1]); } } } } /** * find the largestValue in array * * the function find the largestValue in array * * Parameter * * array is the pointer array that store data * size is the size of the array * * output * return int * reference parameter: array * stream none */ int largestValue(int *array, int size){ int large = array[0]; for ( int i = 1; i < size; i++ ){ if ( large < array[i]){ large = array[i]; } } return large; } /** * find the smallestValue in array * * the function find the smallestValue in array * * Parameter * * array is the pointer array that store data * size is the size of the array * * output * return int * reference parameter: array * stream none */ int smallestValue(int *array, int size){ int small = array[0]; for ( int i = 1; i < size; i++ ){ if ( small > array[i]){ small = array[i]; } } return small; } /** * find the averageValue in array * * the function find the averageValue in array * * Parameter * * array is the pointer array that store data * size is the size of the array * * output * return double * reference parameter: array * stream none */ double averageValue(int *array, int size){ double avg = 0; for ( int i = 0; i < size; i++ ){ avg += array[i]; } return avg/size; } /** * find the medianValue in array * * the function find the medianValue in array * * Parameter * * array is the pointer array that store data * size is the size of the array * * output * return int * reference parameter: array * stream none */ int medianValue(int *array, int size){ int medianNum; bubbleSort(array,size); if ( size % 2 == 1){ medianNum = array[ size / 2 ]; } else { medianNum = array[( size / 2 ) - 1 ]; } return medianNum; }
main.cpp:
#include
using namespace std;
int main () { fstream inputFile; int arraySize; int *numArray; int count = 0; // used to initial the overall Max and Min int largestNum, smallestNum; // open the file inputFile.open("arrays.txt"); // check if the file opened if (inputFile){ // read first arraySize inputFile >> arraySize; // while loop until reach the end of the file while (!inputFile.eof()){ // use new to create space for the array numArray = new int[arraySize]; //read date into the array for(int i=0;i
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
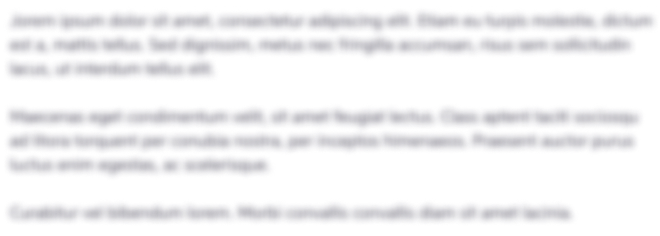
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started