Question
Your FIGHT SIMULATOR will have two opponents: an attacker (that only launches attacks) and a defender (that can only defend against the attacks). There's three
Your FIGHT SIMULATOR will have two opponents: an attacker (that only launches attacks) and a defender (that can only defend against the attacks). There's three types of attacks categorized by height: high, medium and low. Typically you should use class constants for unchanging values (i.e., public final static int HIGH = 1;) but because this is your first Object-Oriented program this requirement is waived for this assignment, constants that are local to a particular method may be used instead).
Similar to the attacks, there's three types of defenses: high, medium and low. If the height of an attack and a defense match then the attack is blocked. Otherwise the attack is counted as a 'hit'. The simulation runs a fixed number of attack-defenses sequences (from 1 - 100 'rounds' of attacks and defenses). The user will determine the number of rounds when the simulation first runs. If a value outside the range is entered then the default number of rounds (ten) will be executed (no re-querying for a new value needed).
Attacker control: The basic version of the program will have an attacker which has an equal probability at attacking at the three heights - you can use integer rather than real number distributions for determining the probabilities. That is, you can call the 'nextInt' method instead of the 'nextFloat' method of class Random. The advanced version will allow user input to specify the probabilities: When the program first runs, the user can also determine the proportion of attacks that originate at the three different heights (e.g., high = 50%, medium = 30%, low = 20%). These three proportions must sum to 100% otherwise the program will use the defaults (again there's an equal probability of each type of attack). Hint: you don't need to use percentages to have an even three way split between the 3 heights (image if you were able simulate the rolling of a 9 sided dice with a Java program...).
Defender control: The defender will be entirely controlled by the computer. The 'unskilled' defender has an equal chance of employing one of the three types of defenses. A program that implements an 'intelligent' defender will analyze the pattern of attacks and adjust the probability of each type of defense being employed over time. (For example if the attacker always throws high attacks then the defender will eventually extrapolate the pattern and use high defenses more frequently). It is up to you to decide how many rounds are needed before the defender begins to adjust to the pattern of attacks. And the value determining the adjustment period can be hard-coded (fixed) into the program (no user input needed at run-time). The minimum number of rounds needed to adapt should be one (with zero attacks thrown there is no pattern to analyze). Prior to analyzing and determining the pattern of attacks the defender should have an equal probability of employing each type of defense.
Exactly what constitutes 'intelligent' for this assignment? Because this isn't a formal class in Artificial Intelligence the definition is fairly broad: as long the program demonstrates the ability to somehow adapt to the pattern of attackers and the marker can clearly see the pattern as the program executes (the display of statistics each round will be very useful for communicating this). If for instance the attacker throws only medium attacks, after the learning period is over the defender should eventually employ only medium height defenses (or at least have an extremely high probability of employing this type of defense).
The program will tally a number of statistics and display them during each round *and* at the end of the simulation.
The information that your program MUST display:
1)Statistics displayed each round: the round number, the type of attack and defense chosen, the result of the attack-defense combination (hit or block).
2)Statistics displayed when the program ends: the total number of successful attacks and blocks and the probabilities of each type of attack-defense at the end (the values for the attacker won't change but an intelligent defender's proportions should go from equal values to more closely reflect the proportions of the attacker).
3)These outputs must not only present the needed information but must also be reasonably formatted. Real number values must display with two places of precision. The program output is even more crucial when you have implemented an intelligent defender. The marker must be able to see the effects of the defender adapting to the pattern of attacks otherwise you may not get credit for your work.
The program will consist of 3 Java source code files (Attacker, Defender, Manager). Each class definition must be in it's own file with the file name matching the class name. :
1) The 'Manager' is not just the start or the 'driver' of the program (contains the 'main()' method) but as the name implies it also acts as an intermediary between the other two classes (e.g., communicates to the defender the type of attack generated by the attacker). The other two classes can send messages (invoke methods) to each other if necessary.
2) The 'Attacker' will be responsible for generating attacks so it should track all information associated with attacking (e.g., numbers of each attack type generated).
3) Likewise the 'Defender' will receive the attack generated by the attacker, generate a defense and determine the result of the attack-defense combination. Also the defender should track information associated with defense (e.g., the numbers of each type of defense used). Since the information for the attacks and defenses must be tracked by this class the methods for displaying the round-by-round report and the end of simulation report should be displayed here.
PLEASE NOTIFY ME IF YOU'RE STARTED DOING THE PROGRAM!
Example of program:
Number of attack rounds : 100
Enter the percentages for the number of attacks that will be directed : low, medium , high. The total of the three percentages must sum to 100%
Percentage of attacks that will be aimed low: 0
Percentage of attacks that will be aimed at medium height: 0
Percentage of attacks that will be aimed high: 100
Combat begins
_______________
Round 1. Attacker: High Defender: Low Hit
Round 2. Attacker: High Defender: Low Hit
Round 3. Attacker: High Defender: High Block
Round 4. Attacker: High Defender: Low Hit
Round 5. Attacker: High Defender: Medium Hit
Etc...
Round 100. Attacker: High Defender: High Block
Summary of Combat
Total hits: 14 Total blocks: 86
Attacker proportions: Low 0.00% Medium 0.00% High 100.00%
Defender proportions: Low 9.00% Medium 2.00% High 89.00%
If you need any specification pls write,and pls do it as soon as possible pls
Step by Step Solution
There are 3 Steps involved in it
Step: 1
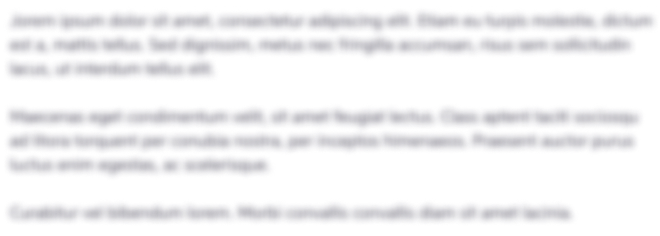
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started