Question
Your task for the first assignment is to write a program that will work out what the local time is in your town so when
Your task for the first assignment is to write a program that will work out what the local time is in your town so when phoning home you don't call too early or too late and wake your family.
To do this, you will write a function to calculate the local time in a given town (in Australia or New Zealand), given the current time in UTC.
You will also be required to write a series of tests for this function.
The main task in this assignment to write a function, get_local_time, which, given as input a town in Australia or New Zealand and a date and time in UTC, returns the local time in that town.
The town will be one of these 14 towns: Adelaide, Brisbane, Broken Hill, Canberra, Darwin, Eucla, Hobart, Lord Howe Island, Melbourne, Perth, Sydney, Auckland, Christchurch, Wellington.
Initial code:
// time2code.c // Assignment 1, COMP1511 18s1: Time To Code. // // This program by YOUR-NAME-HERE (z5555555) on INSERT-DATE-HERE // // Version 1.0.2: Add version numbers and header comment. // Version 1.0.1: Fix day/time variable mix-up in main function. // Version 1.0.0: Assignment released. #include#include // You must use these #defines - DO NOT CHANGE THEM #define TOWN_ADELAIDE 0 #define TOWN_BRISBANE 1 #define TOWN_BROKEN_HILL 2 #define TOWN_CANBERRA 3 #define TOWN_DARWIN 4 #define TOWN_EUCLA 5 #define TOWN_HOBART 6 #define TOWN_LORD_HOWE_IS 7 #define TOWN_MELBOURNE 8 #define TOWN_PERTH 9 #define TOWN_SYDNEY 10 // New Zealand #define TOWN_AUCKLAND 11 #define TOWN_CHRISTCHURCH 12 #define TOWN_WELLINGTON 13 // Australia #define TIMEZONE_AWST_OFFSET 800 // Australian Western Standard Time #define TIMEZONE_ACWST_OFFSET 845 // Australian Central Western Standard Time #define TIMEZONE_ACST_OFFSET 930 // Australian Central Standard Time #define TIMEZONE_ACDT_OFFSET 1030 // Australian Central Daylight Time #define TIMEZONE_AEST_OFFSET 1000 // Australian Eastern Standard Time #define TIMEZONE_AEDT_OFFSET 1100 // Australian Eastern Daylight Time #define TIMEZONE_LHST_OFFSET 1030 // Lord Howe Standard Time #define TIMEZONE_LHDT_OFFSET 1100 // Lord Howe Daylight Time // New Zealand #define TIMEZONE_NZST_OFFSET 1200 // NZ Standard Time #define TIMEZONE_NZDT_OFFSET 1300 // NZ Daylight Time // returned by get_local_time #define INVALID_INPUT (-1) // ADD YOUR #defines (if any) here int get_local_time(int town, int utc_month, int utc_day, int utc_time); void run_unit_tests(void); // ADD PROTOTYPES FOR YOUR FUNCTIONS HERE // DO NOT CHANGE THIS FUNCTION // This main function won't be marked as part of the assignment // It tests the functions you have to write. // Do not change this main function. If it does not work with // your functions you have misunderstood the assignment. int main(void) { int call_get_local_time = 0; printf("Enter 0 to call run_unit_tests() "); printf("Enter 1 to call get_local_time() "); printf("Call which function: "); scanf("%d", &call_get_local_time); if (!call_get_local_time) { printf("calling run_unit_tests() "); run_unit_tests(); printf("unit tests done "); return 0; } int town = 0; int month = 0; int day = 0; int time = 0; printf("Enter %d for Adelaide ", TOWN_ADELAIDE); printf("Enter %d for Brisbane ", TOWN_BRISBANE); printf("Enter %d for Broken_hill ", TOWN_BROKEN_HILL); printf("Enter %d for Canberra ", TOWN_CANBERRA); printf("Enter %d for Darwin ", TOWN_DARWIN); printf("Enter %d for Eucla ", TOWN_EUCLA); printf("Enter %d for Hobart ", TOWN_HOBART); printf("Enter %d for Lord Howe Island ", TOWN_LORD_HOWE_IS); printf("Enter %d for Melbourne ", TOWN_MELBOURNE); printf("Enter %d for Perth ", TOWN_PERTH); printf("Enter %d for Sydney ", TOWN_SYDNEY); printf("Enter %d for Auckland ", TOWN_AUCKLAND); printf("Enter %d for Christchurch ", TOWN_CHRISTCHURCH); printf("Enter %d for Wellington ", TOWN_WELLINGTON); printf("Which town: "); scanf("%d", &town); printf("Enter UTC month as integer 1..12: "); scanf("%d", &month); printf("Enter UTC day as integer 1..31: "); scanf("%d", &day); printf("Enter UTC time as hour * 100 + minutes "); printf("Enter UTC time as integer [0..2359]: "); scanf("%d", &time); int local_time = get_local_time(town, month, day, time); if (local_time == INVALID_INPUT) { printf("invalid input - local time could not be calculated "); } else { printf("local_time is %d ", local_time); } return 0; } // Given an Australian town and a 2018 UTC time // return the local time in the Australian town // // time is returned as hours*100 + minutes // INVALID_INPUT is return for invalid inputs // // utc_month should be 1..12 // utc_day should be 1..31 // utc_time should be 0..2359 // utc_time is hours*100 + minutes // // note UTC year is assumed to be 2018 // note UTC seconds is assumed to be zero // int get_local_time(int town, int utc_month, int utc_day, int utc_time) { // CHANGE THIS FUNCTION // YOU ARE NOT PERMITTED TO USE ARRAYS, LOOPS, PRINTF OR SCANF // SEE THE ASSIGNMENT SPECIFICATION FOR MORE RESTRICTIONS return utc_time + TIMEZONE_AEDT_OFFSET; } // ADD YOUR FUNCTIONS HERE // ADD A COMMENT HERE EXPLAINING YOUR OVERALL TESTING STRATEGY // run unit tests for get_local_time void run_unit_tests(void) { // 2 example unit tests // UTC midnight on 20th March -> time in Sydney: 11am AEDT assert(get_local_time(TOWN_SYDNEY, 3, 20, 0) == 1100); // 42 is not a valid month assert(get_local_time(TOWN_SYDNEY, 42, 0, 0) == INVALID_INPUT); // ADD YOUR ASSERT STATEMENTS HERE // you should add at least 40 more assert statements to this function // with a comment for each test explaining it // there should be comment before this function // explaining your overall testing strategy }
The date will be in 2018.
You are also required to write a function, run_unit_tests, which tests get_local_time on valid and invalid inputs.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
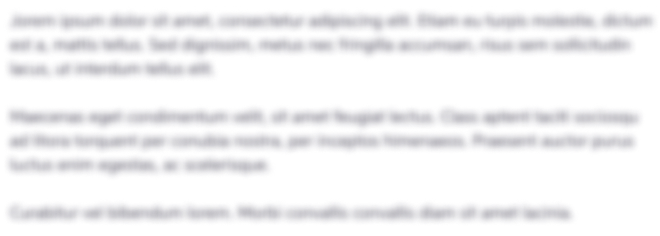
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started