Question
Please help Create classes that implement the Comparable interface Test the classes by sorting an ArrayList of objects that implement the Comparable interface Understand the
Please help
Create classes that implement the Comparable interface
Test the classes by sorting an ArrayList of objects that implement the Comparable interface
Understand the use of methods to breakdown a problem into smaller pieces to code and test
Read in object instance variable values from a file
Take a detailed requirements and system design specification and code up a small program
Read this Requirements and System Design document carefully. Being able to translate this into code is an important piece of this exercise. After reading the document, if you still don't understand this lab, then please:
Read it again, more slowly (perhaps out loud).
While reading it, take notes. Writing something down often helps you to understand it better.
Email me with specific questions. Just saying "I don't understand" won't cut it.
Come to the Open Lab on Wednesday night.
Make an appointment with the lead instructor See Faculty Information
You will be using most of this code again in Lab 9.
Read the following documents before attempting this Lab:
LessonsProjectsQuizzes
CodeStyleGuidelines
CreateProjectPrograms
Lesson3Slides and Textbook Chapters 11-13
Lesson 3 Source Code
Worth 70% of Lab/Quiz grade.
Submission
Name your project Lab3.
Name the classes PersonSort, Person, and MyDate.
Name the zip file Lab3.zip and submit it to Blackboard.
Do not submit any rar files.
I do not grade programs that do not compile or run.
Email me broken code all zipped up as you would submit it.
Do not submit broken code.
The Lab3 project folder should contain the following files:
PersonSort.java
Person.java
MyDate.java
Requirements Specification and System Design
This program creates two classes that implement the Comparable interface. To understand interfaces, see slides 119-137 (also book, Google etc). You do not have to provide the code for the Comparable interface, as it is provided by the Java API in the java.lang package.
The Person and MyDate classes will be tested in a third class that creates a group of Person objects from data in a file. These Person objects will be placed in an ArrayList and the ArrayList is sorted using two popular sorting algorithms. The sorted lists are displayed.
There is example code in the Lesson 3 Source Code and Lecture slides for most of this program. Use Khan Academy online to find good explanations of selection and insertion sort algorithms.
The Comparable Interface
The Comparable interface is a very commonly implemented interface in Java
The Comparable interface provides a single method that can be used to compare two objects: int compareTo (E obj), where E is the class type implementing the interface.
For this project both MyDate and Person implement the Comparable interface and therefore need to have a declaration and implementation (code) for the compareTo method.
For MyDate code up: int compareTo (MyDate obj)
For Person code up: int compareTo (Person obj)
Create a project called Lab3. Create a tester class called PersonSort and two classes that implement the Comparable interface one called the Person class and the other called the MyDate class. The Personclass has a name and a birthdate. The name is a String object and the birthdate is a MyDate object.
MyDate Class
The MyDate class has three private instance variables:
int month (1-12)
int day (1-31)
int year.
It implements the Comparable interface. In the compareTo() method, make sure you check for year, month and day in that order. This class has two other methods: a three-parameter constructor that initializes the three instance variables (month, day and year) and an override of Objects toString() method that displays the date as month, day, and year.
So the MyDate class has three public methods:
MyDate (int month, int day, int year)
int compareTo (MyDate obj)
String toString()
DO NOT create any other instance variables or methods. This is an immutable class.
Person Class
The Person class has two private instance variables:
String name
MyDate birthday
It implements the Comparable interface. In the compareTo() method, compare the birthdays first, and then the names, using the compareTo() methods of the MyDate and String classes. It has two other methods: a four- parameter constructor that initializes the instance variables (name and birthday) passing three of the parameters to the MyDate birthday object in order to initialize it and an override of Objects toStringmethod that displays the name and the birthday (using the toString method of the birthday object).
So the Person class has three public methods:
Person (String name, int month, int day, int year)
int compareTo (Person obj)
String toString()
DO NOT create any other instance variables or methods. This is an immutable class.
PersonSort Class
The PersonSort class is the tester class for this program. It has four static methods: main, populate, selectionSort and insertionSort.
The main method
creates an ArrayList (ArrayList
populates it from a file that is supplied to you
copies the ArrayList to another one
uses the first ArrayList and sorts it using an insertion sort algorithm
displays the sorted ArrayList
uses the second ArrayList and sorts it using a selection sort algorithm
displays the sorted ArrayList
The populate method reads in the Person data from a file (see below for the format). See slide 99 for a good example of how to do this. DO NOT assume that you know how many records are in the file. It creates a Person object from each line in the file and adds the object to the first ArrayList.
The selectionSort method sorts the ArrayList using the selection sort algorithm.
The insertionSort method sorts the ArrayList using the insertion sort algorithm.
You must implement these sorting algorithms. A version of this code is containined in the Lesson 3 Source Code. You are not permitted to use the sort method of the Collections framework, or its swap method.The Person objects must be sorted, first by birthday and then by name.
The filename of the supplied file is Persons.txt. Place it in the src folder of either Eclipse or IntelliJ. Create a global static constant to hold the file name that will be passed to the File object for reading the file. DO NOT assume that you know how many records are in the file.
static final String PERSON_FILE = ./src/Persons.txt;
The format of the file is: name month day year
You may use a try/catch block or let main throw the FileIO exception.
Sample Output:
List sorted by selection sort
Matt: 9-8-1950
Tom: 8-21-1952
Matt: 9-8-1952
Mark: 9-12-1952
Mary: 10-2-1954
Glenn: 11-27-1970
Chris: 12-27-1970
Glenda: 12-27-1971
Jenell: 12-27-1971
Madon: 9-2-1976
Jacob: 4-17-1978
Crystal: 12-12-1978
Christine: 7-29-1981
April: 7-2-1983
Thiec: 8-30-1984
Jane: 3-1-1985
Hen: 4-1-1985
Jan: 4-2-1986
Yung: 4-2-1986
Ashton: 8-10-1995
Cassidy: 8-5-1997
Chaz: 9-10-1998
Merli: 9-10-1998
Terra: 1-16-1999
Annie: 8-28-2002
Phoenix: 10-25-2004
Lilly: 12-7-2008
Bella: 8-28-2010
Elijah: 3-19-2013
Suri: 8-14-2014
List sorted by insertion sort
Matt: 9-8-1950
Tom: 8-21-1952
Matt: 9-8-1952
Mark: 9-12-1952
Mary: 10-2-1954
Glenn: 11-27-1970
Chris: 12-27-1970
Glenda: 12-27-1971
Jenell: 12-27-1971
Madon: 9-2-1976
Jacob: 4-17-1978
Crystal: 12-12-1978
Christine: 7-29-1981
April: 7-2-1983
Thiec: 8-30-1984
Jane: 3-1-1985
Hen: 4-1-1985
Jan: 4-2-1986
Yung: 4-2-1986
Ashton: 8-10-1995
Cassidy: 8-5-1997
Chaz: 9-10-1998
Merli: 9-10-1998
Terra: 1-16-1999
Annie: 8-28-2002
Phoenix: 10-25-2004
Lilly: 12-7-2008
Bella: 8-28-2010
Elijah: 3-19-2013
Suri: 8-14-2014
This is the Persons.txt file
Merli 9 10 1998 Elijah 3 19 2013 Christine 7 29 1981 Annie 8 28 2002 Bella 8 28 2010 Matt 9 8 1952 Cassidy 8 5 1997 Crystal 12 12 1978 Glenda 12 27 1971 Hen 4 1 1985 Jacob 4 17 1978 Matt 9 8 1950 Jenell 12 27 1971 Lilly 12 7 2008 April 7 2 1983 Yung 4 2 1986 Madon 9 2 1976 Chris 12 27 1970 Mary 10 2 1954 Jane 3 1 1985 Mark 9 12 1952 Chaz 9 10 1998 Phoenix 10 25 2004 Suri 8 14 2014 Terra 1 16 1999 Tom 8 21 1952 Ashton 8 10 1995 Thiec 8 30 1984 Jan 4 2 1986 Glenn 11 27 1970
Step by Step Solution
There are 3 Steps involved in it
Step: 1
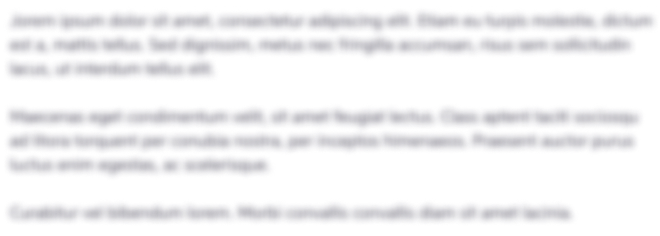
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started