Question
Project Requirements Create a class that simulates the functionality of a simple car. You can do simple things like driving, adding gas, changing oil and
Project Requirements
Create a class that simulates the functionality of a simple car. You can do simple things like driving, adding gas, changing oil and honking the horn. The cars oil should be changed every 5,000 miles.
Class Specifications
The design specifications of a class include:
- declarations for a list of instance fields (think of these as nouns) and
- method declarations (think of these as verbs), including parameters, which determine how objects are used.
Implement a class, called Car, with the following instance variables/fields and methods. Exact spelling is required for the name of the Car class and the headers of all the methods within the Car class. Do not make any changes to the following requirements. If you do so, the automated tests that we provide you (see below) will likely fail.
Class Fields/Instance Variables
Declare meaningful names with appropriate data types for each of these private instance variables:
- String for the make of the car (e.g. Ford)
- String for the model of the car (e.g. Explorer)
- an integer for the year of the car
- a double for the odometer
- a double for the mileage of the next oil change
- a double for the gallons of gas in the tank
- a double for miles per gallon
- a constant for the gas tank capacity
private static final double TANK_CAPACITY = 12.5;
- a constant for the number of miles in between oil change.
private static final int MILES_BETWEEN_OIL_CHANGE = 5000;
- an object of the DecimalFormat class to format the numbers (miles and gas)
private static DecimalFormat df = new DecimalFormat ("###,##0.00");
Phase 1 (20 pts)
Constructors
- public Car () this is the default constructor; it assigns the following initial values to the instance variables:
- make: Ford
- model: Explorer
- year of the car: 2020
- miles per gallon: 29.0
- fills the tank
- sets the odometer to zero
- sets the next oil change reminder.
- public Car (String make, String model, int y, double mpg) - this constructor sets the make, model, year and miles per gallon to the provided input parameters and sets the rest of the instance variables to the same values as the default constructor does.
Accessor Methods
- public String getMake ( ) - return the make of the car.
- public String getModel ( ) - return the model of the car.
- public double getMpg ( ) - return the miles per gallon.
- public int getYear ( ) - return the year of the car
- public double getMileageNextOilChange ( ) - return mileage of next oil change
- public double checkOdometer ( ) - return the current mileage.
- public double checkGasGauge ( ) - return the amount of gas in the tank.
Mutator Methods
- public void setMake (String make) set the make of the car to the value of the input parameter
- public void setModel (String model) set the model of the car to the value of the input parameter
- public void setMpg (double value) set the miles per gallon of the car to the value of the input parameter
- public void setYear (int y) set the year of the car to the value of the input parameter
- public void honkHorn ( ) - print a message more creative than "beep beep!"
Software Testing TestCar class (10 pts)
Software developers must plan to write tests, from the very beginning of the project, to ensure that their solution is correct. BlueJ allows you to instantiate objects and invoke individual methods. You can carefully check each method and compare actual results with expected results. However, this gets monotonous. Another approach is to write a main method that calls all the other methods.
For this project, write a TestCar class that contains a main method that instantiates at least two Cars.
The following code is a brief and incomplete example. You can use this code as a starting point. You shall add instructions to instantiate your favorite car and invoke each of the methods with a variety of parameter values to test each option within each method.
Notes:
- To be able to test the functionality of each phase, you will add instructions to the main method in each phase.
It takes careful consideration to anticipate and test every possibility.
JUST SOFTWARE TEST CARTEST DO NOT NEED 1st PART
Step by Step Solution
There are 3 Steps involved in it
Step: 1
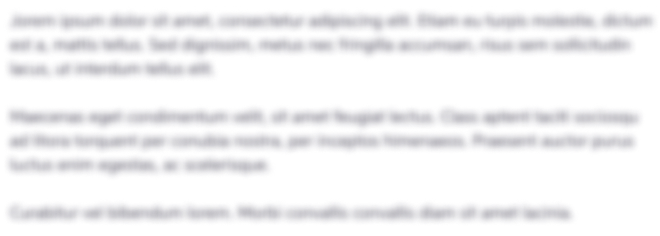
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started