Question
this is the feedback you can see my output and Expected output in every section. Please so please help me to match my output and
this is the feedback you can see my output and Expected output in every section. Please so please help me to match my output and Expected output to match out.
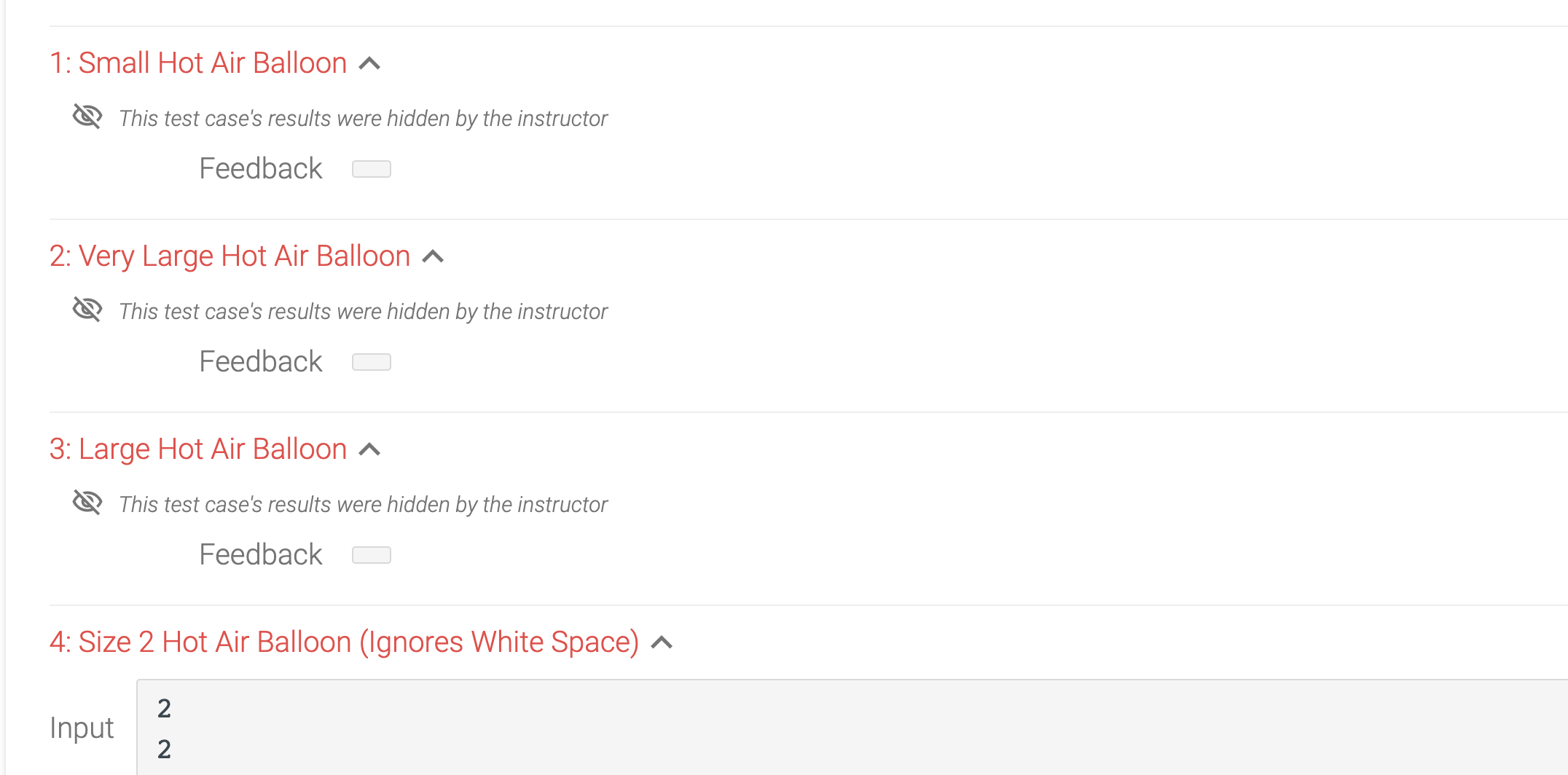
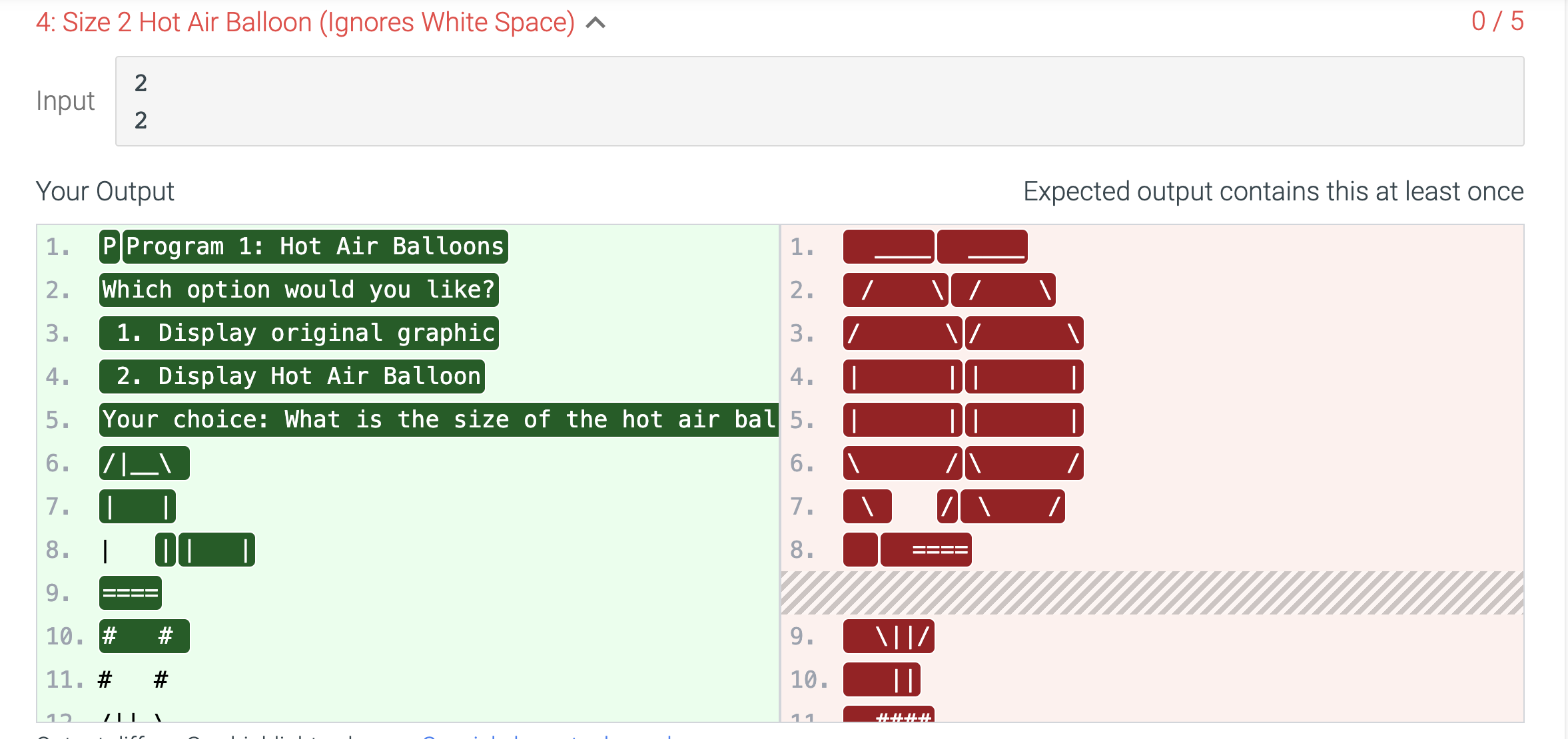
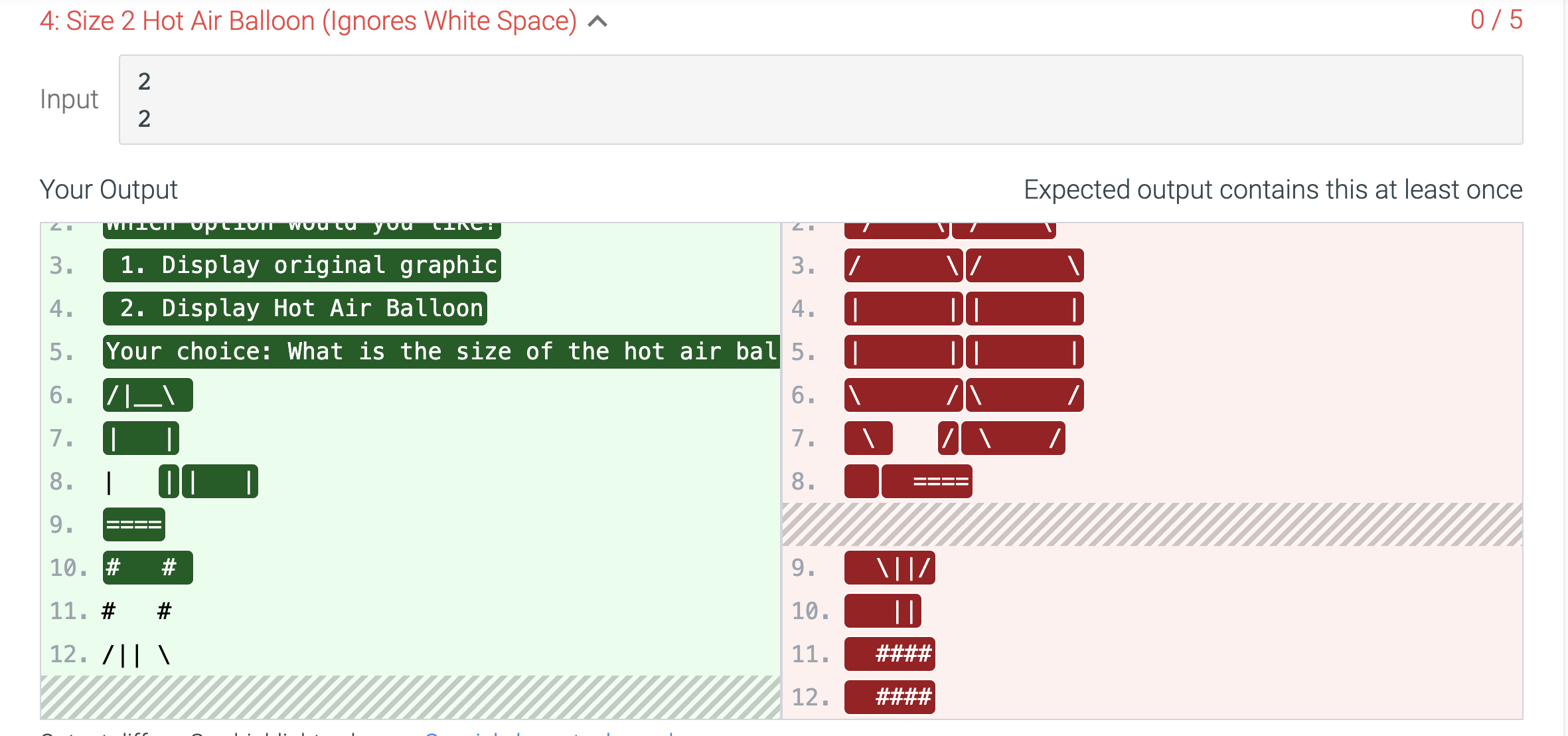
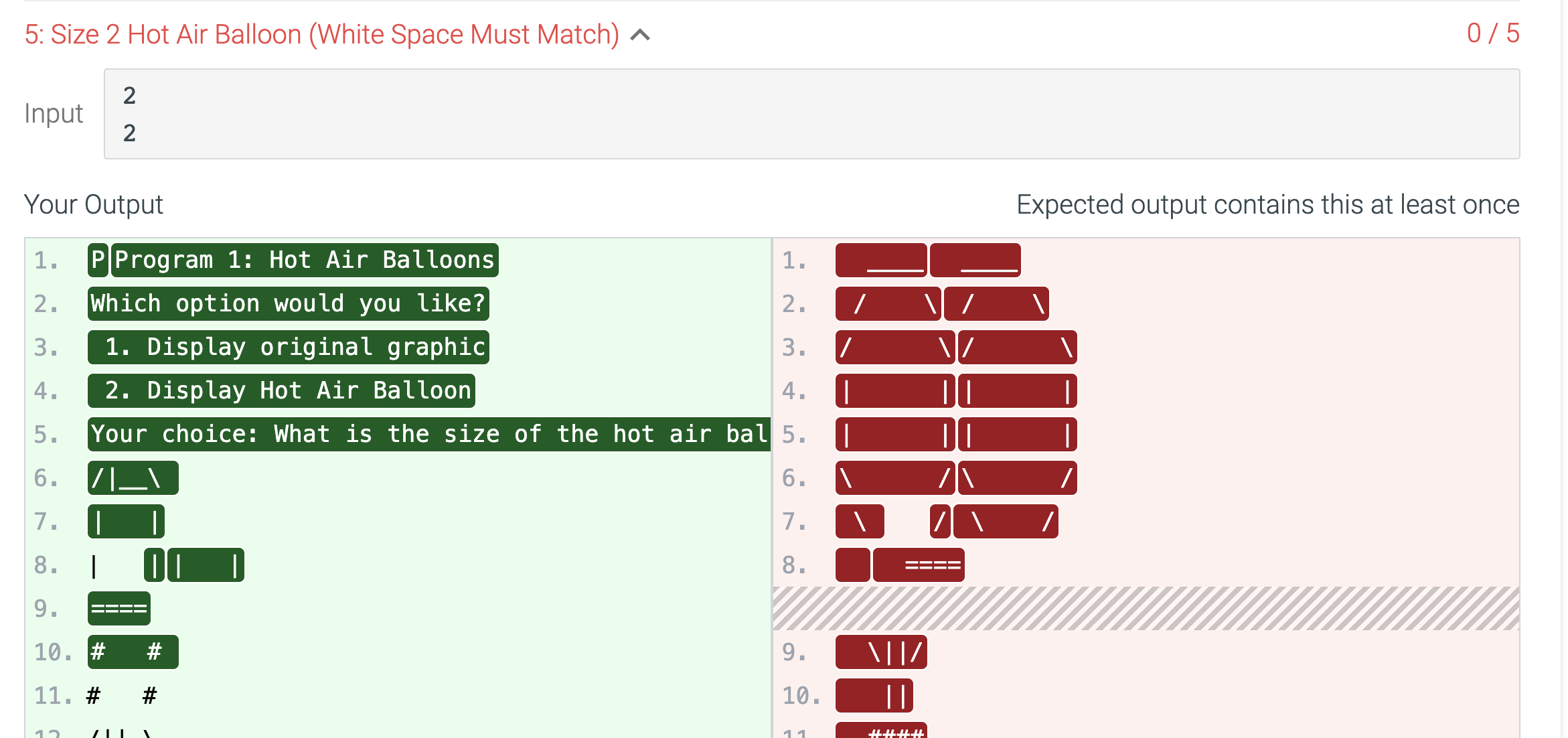
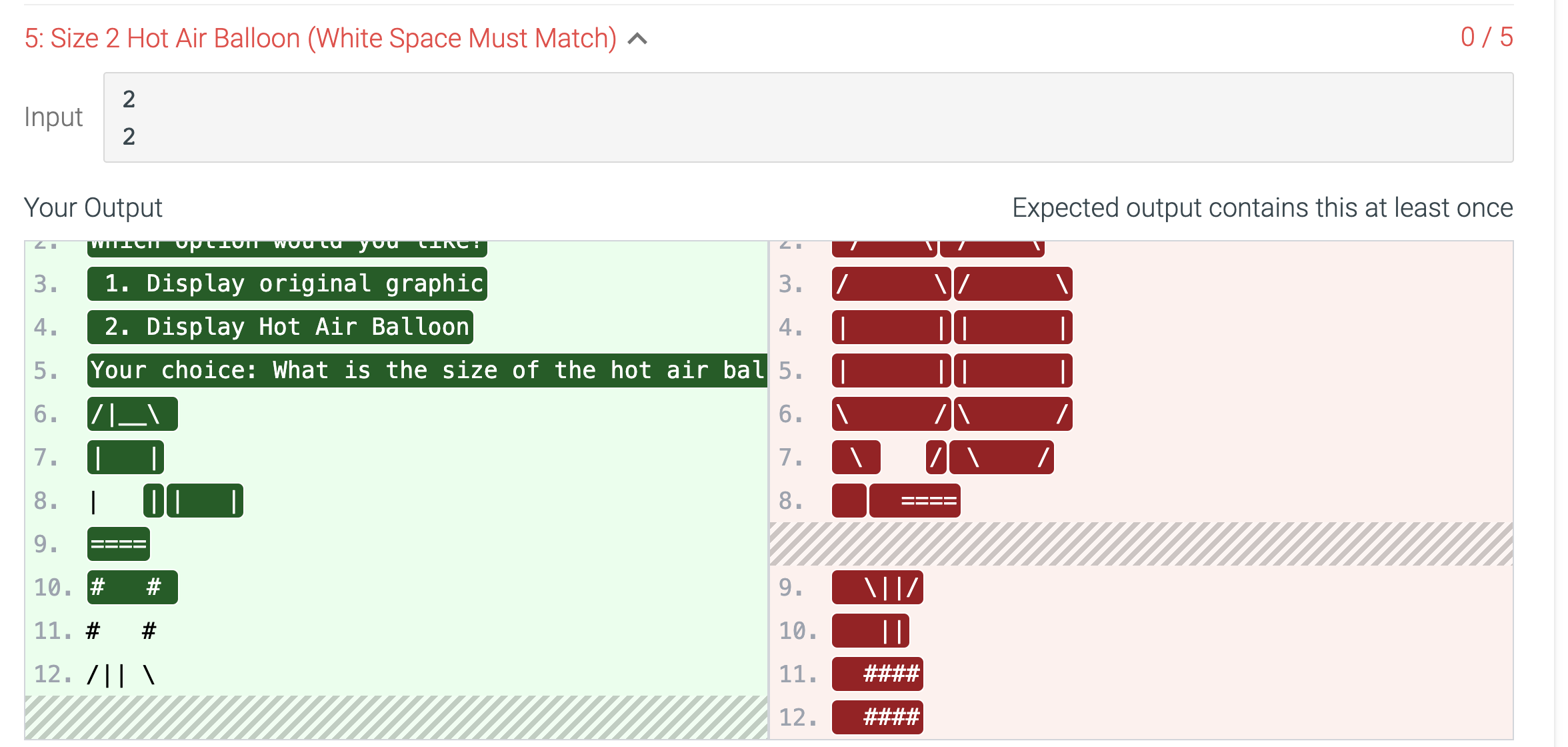
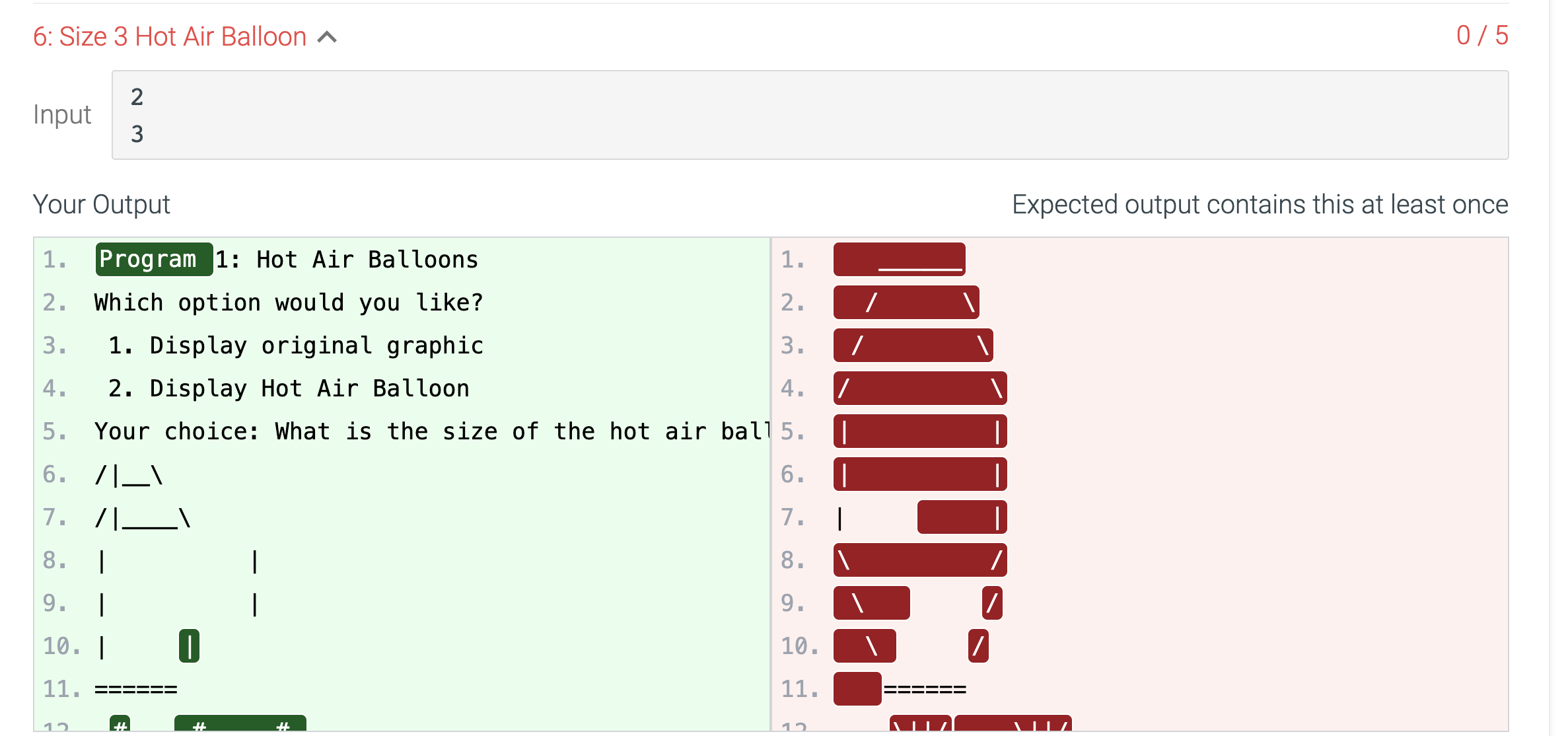
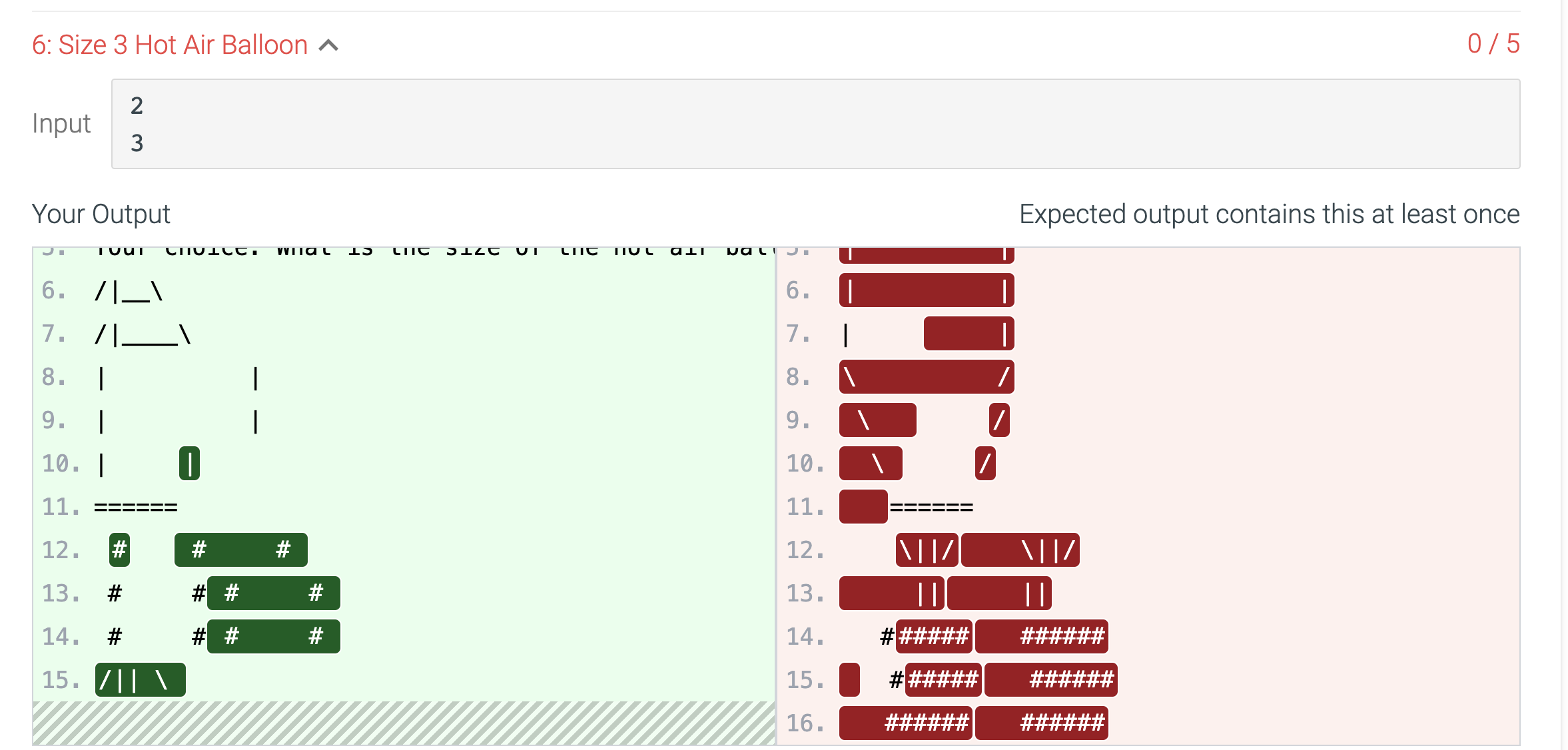
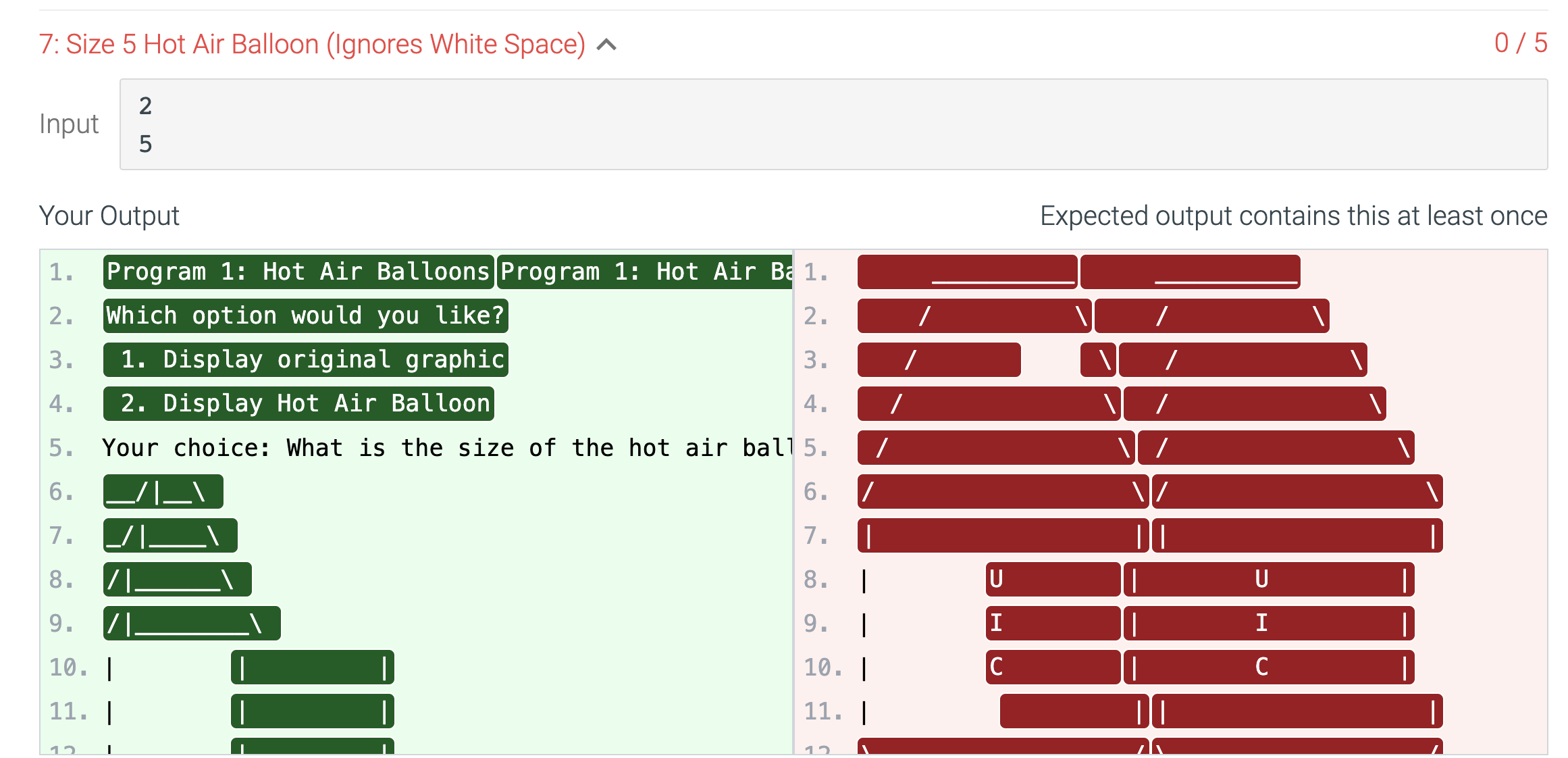
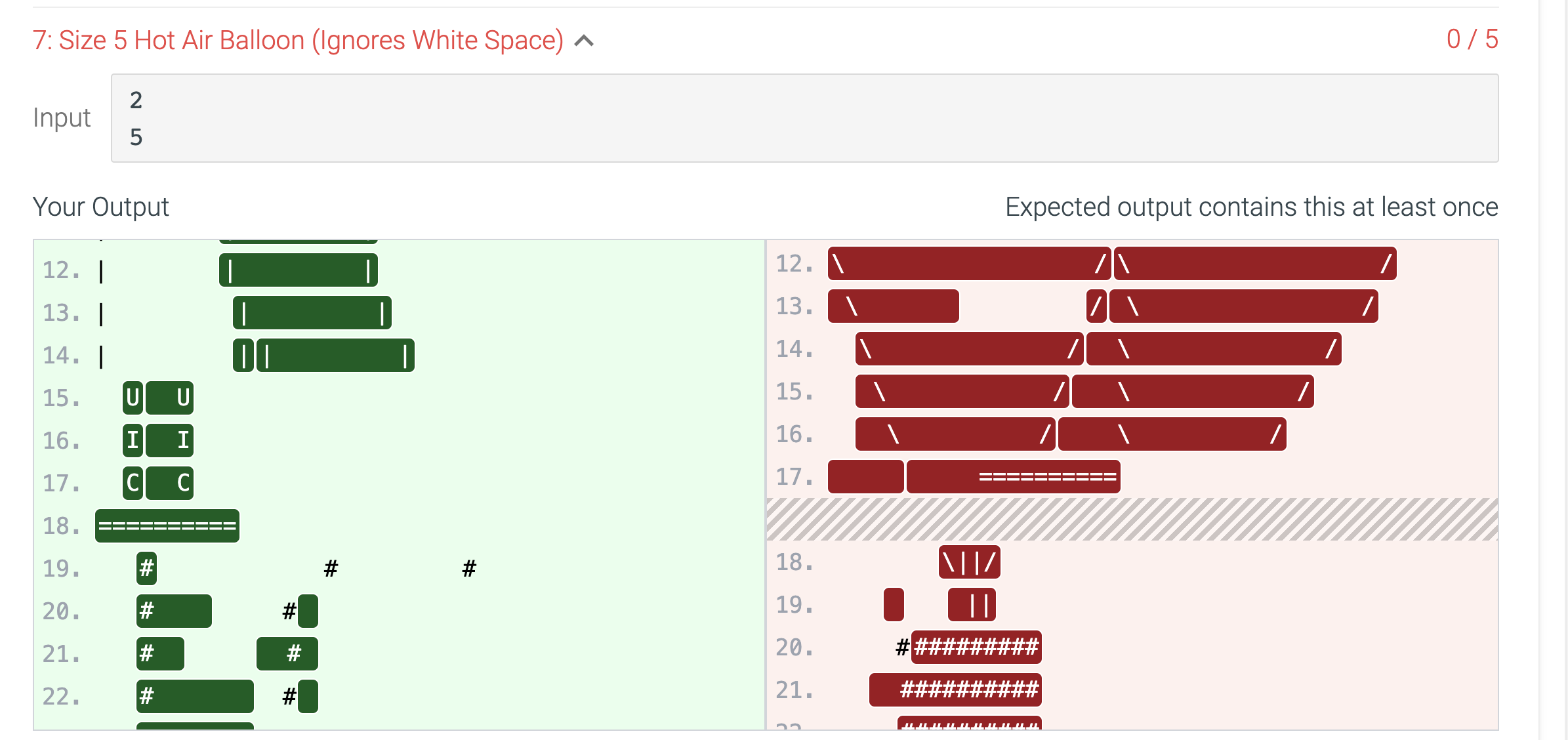
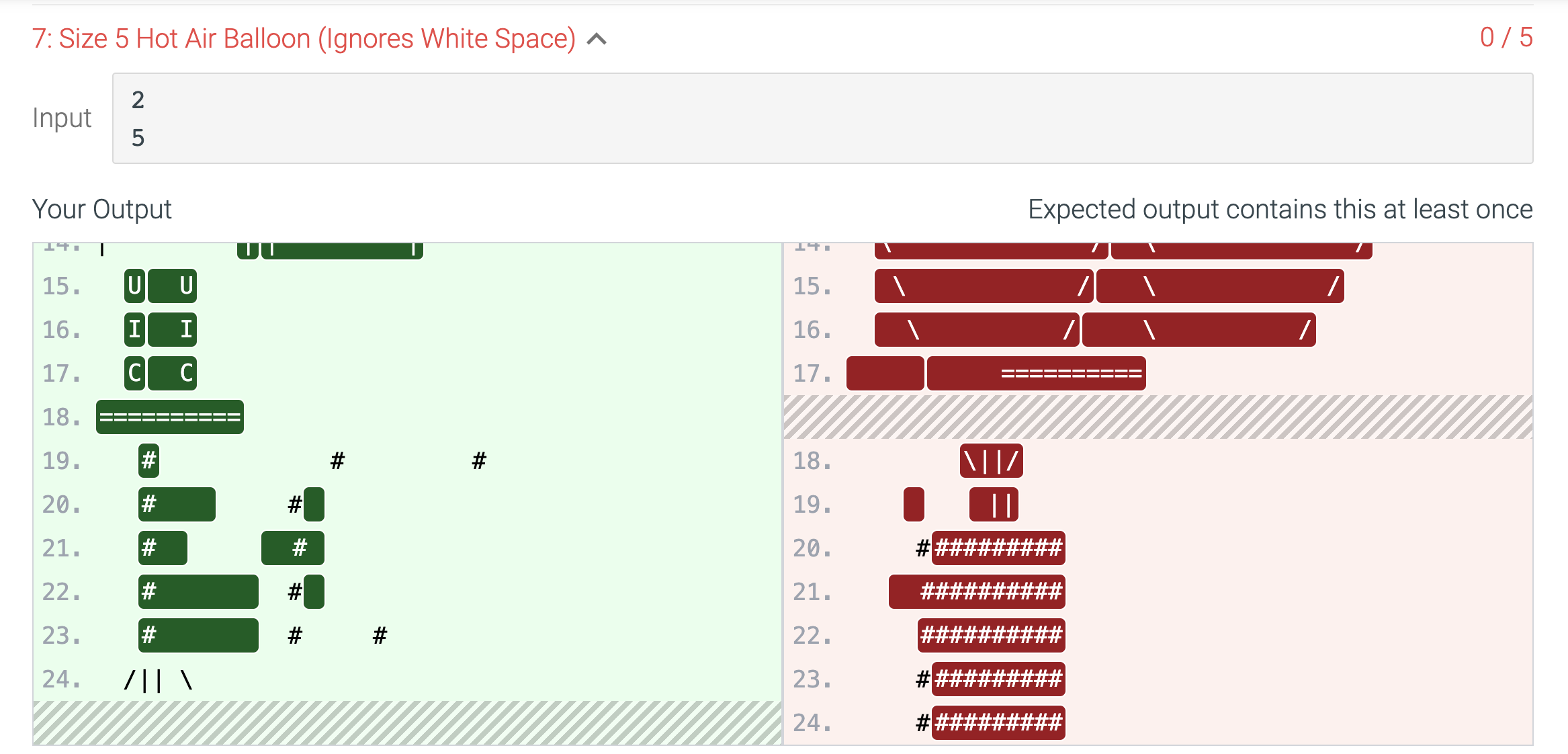
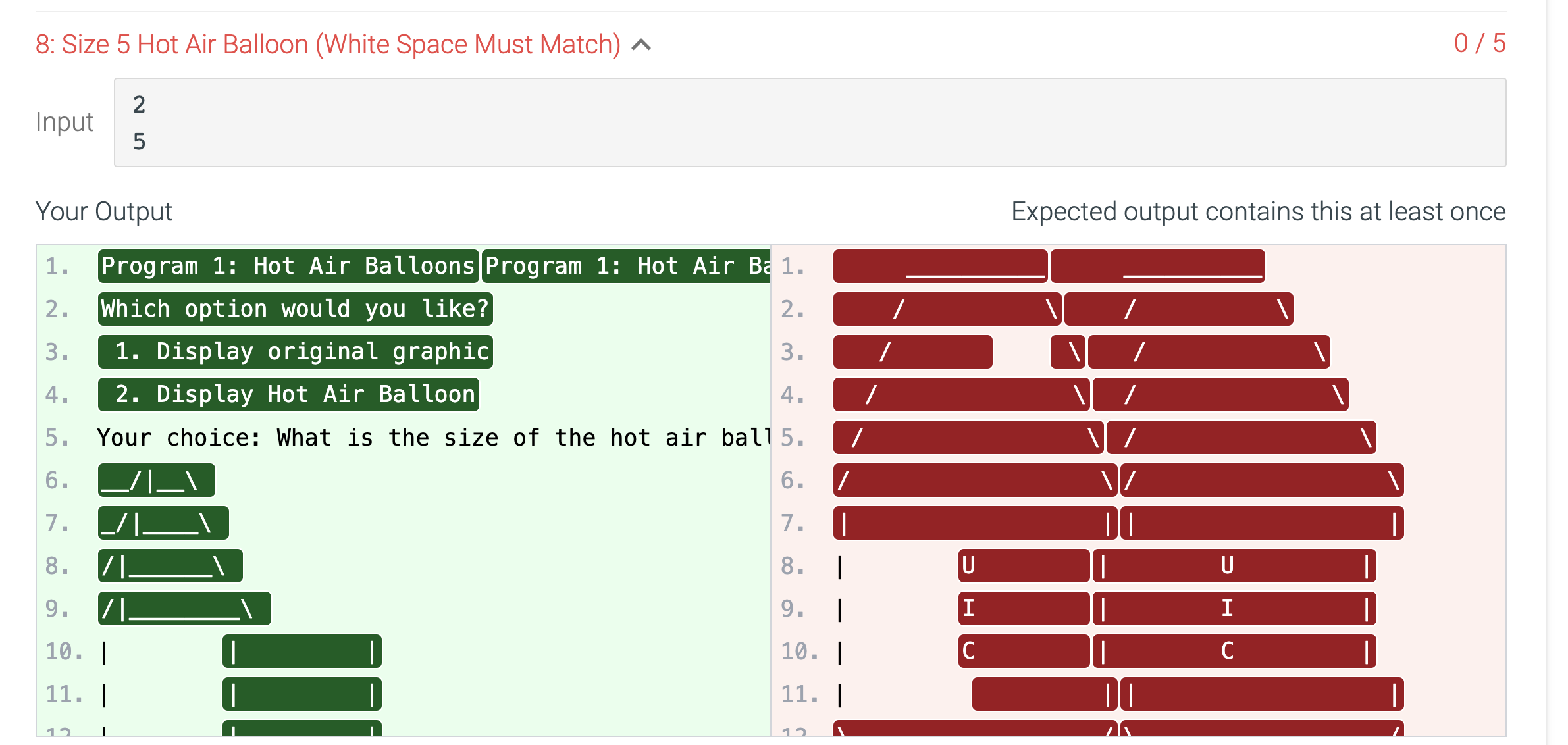
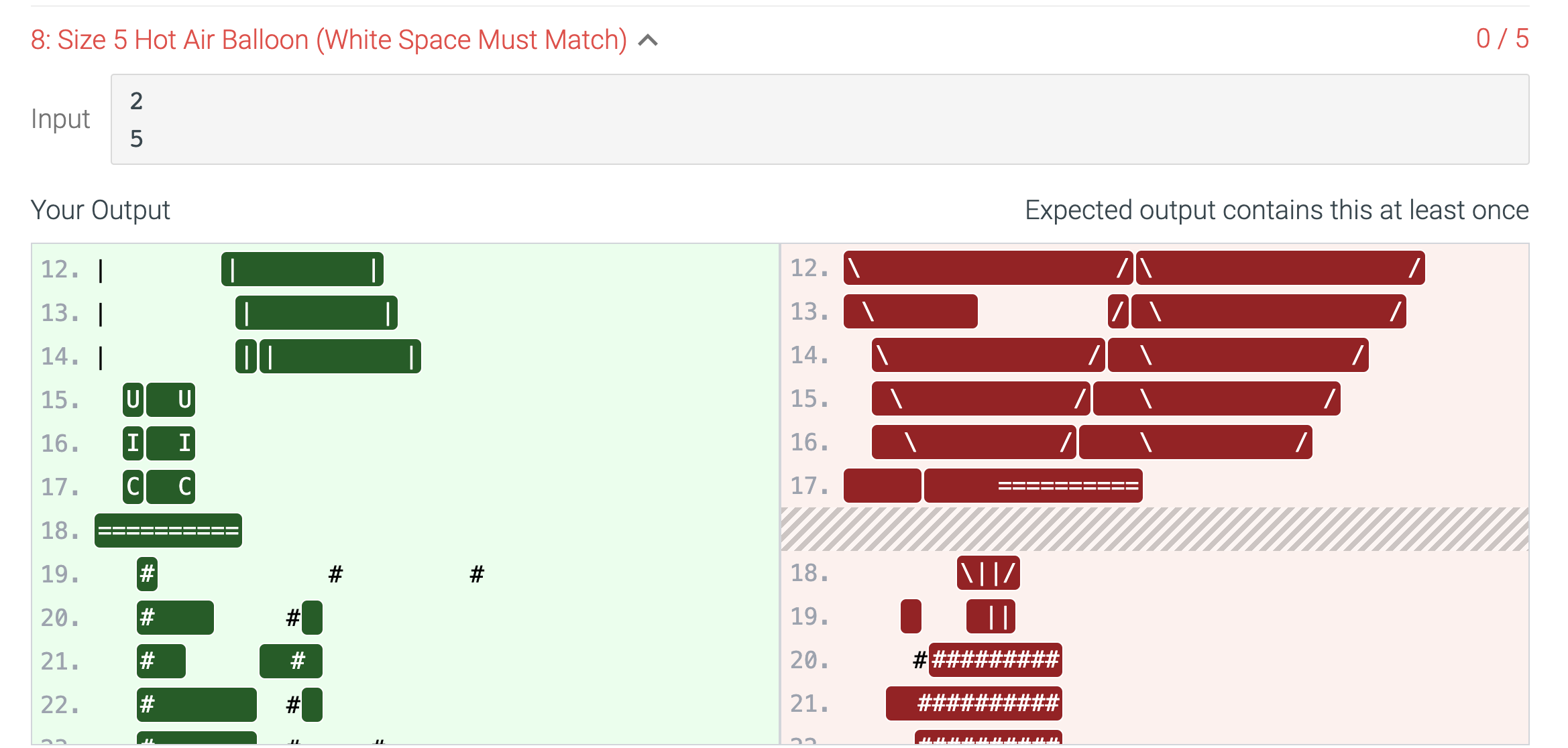
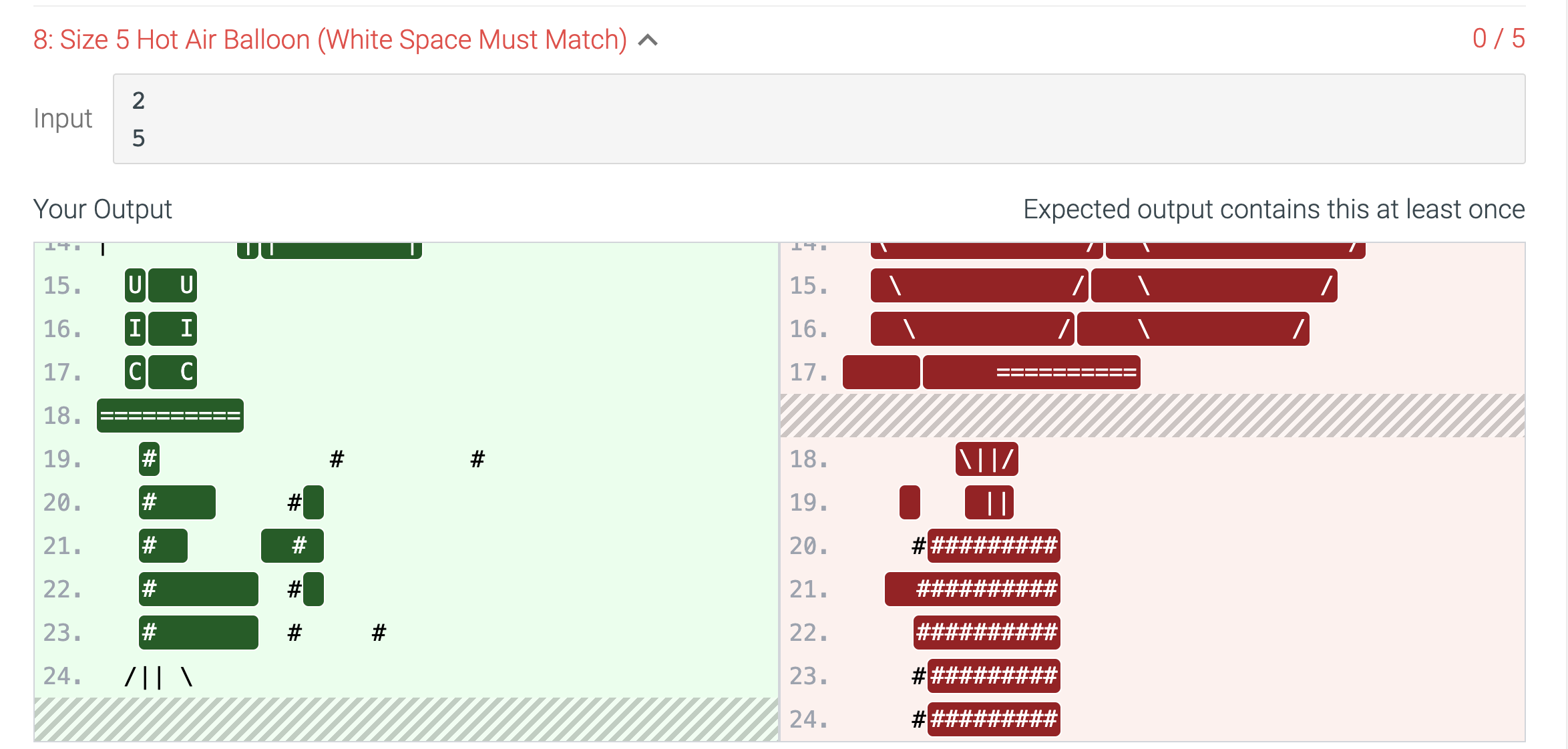
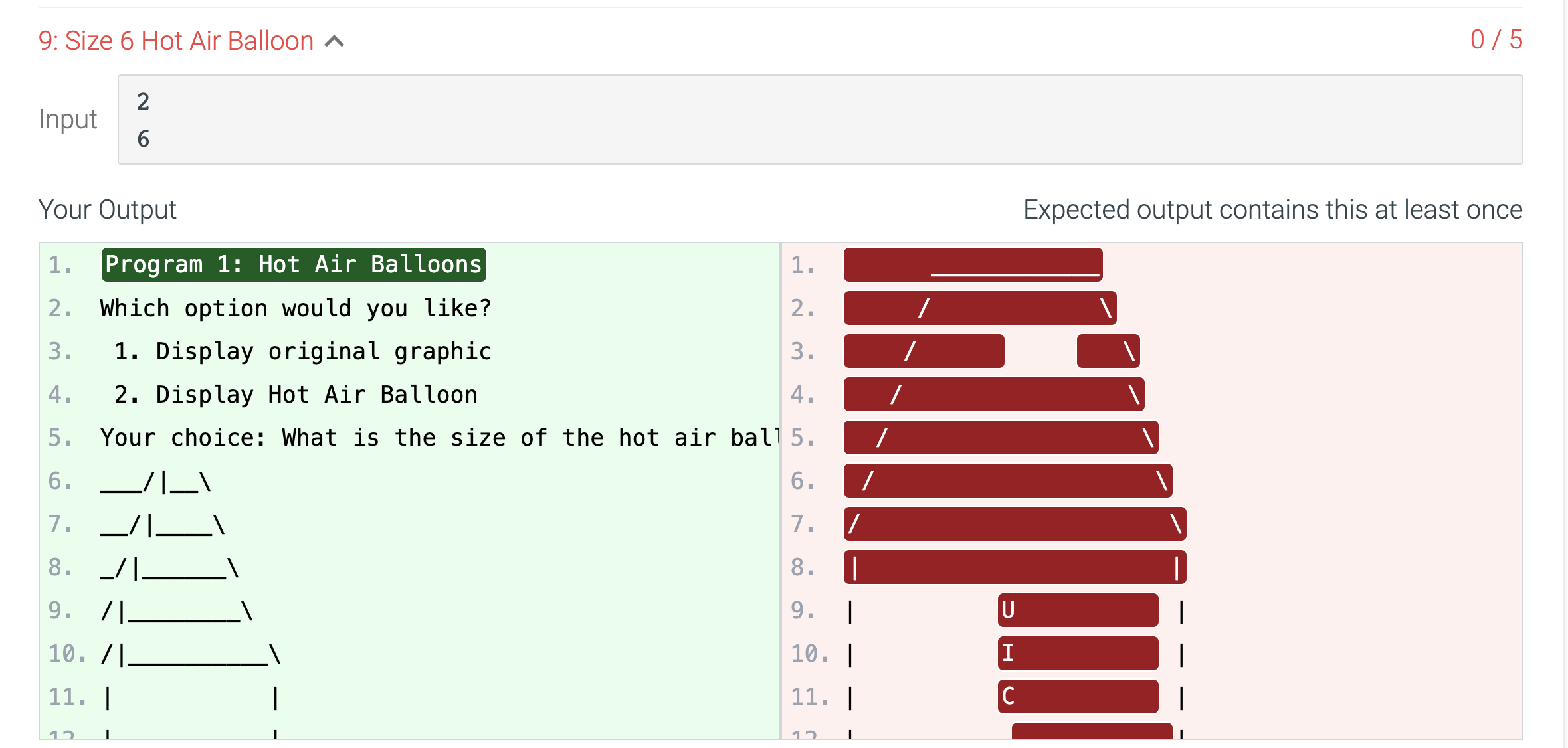
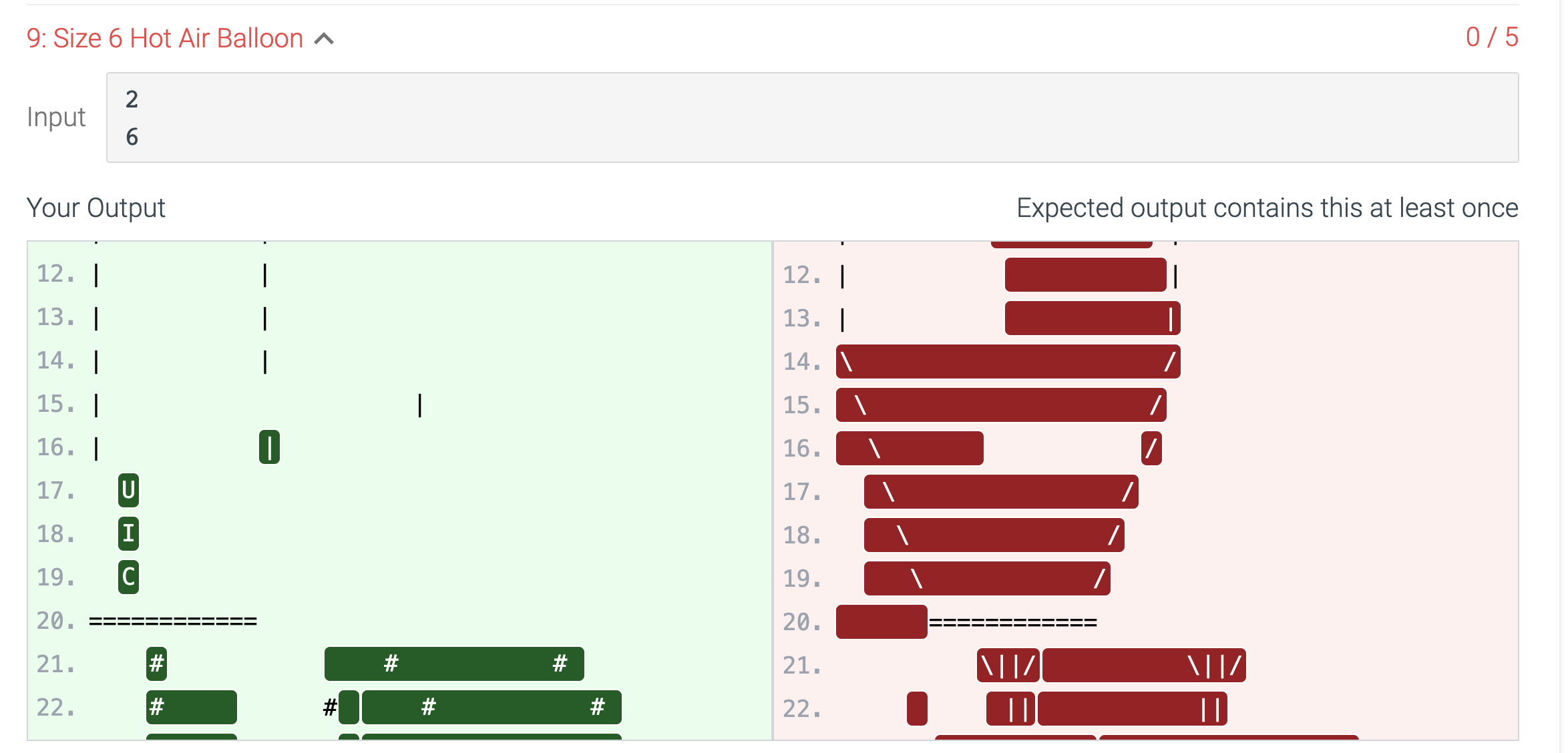
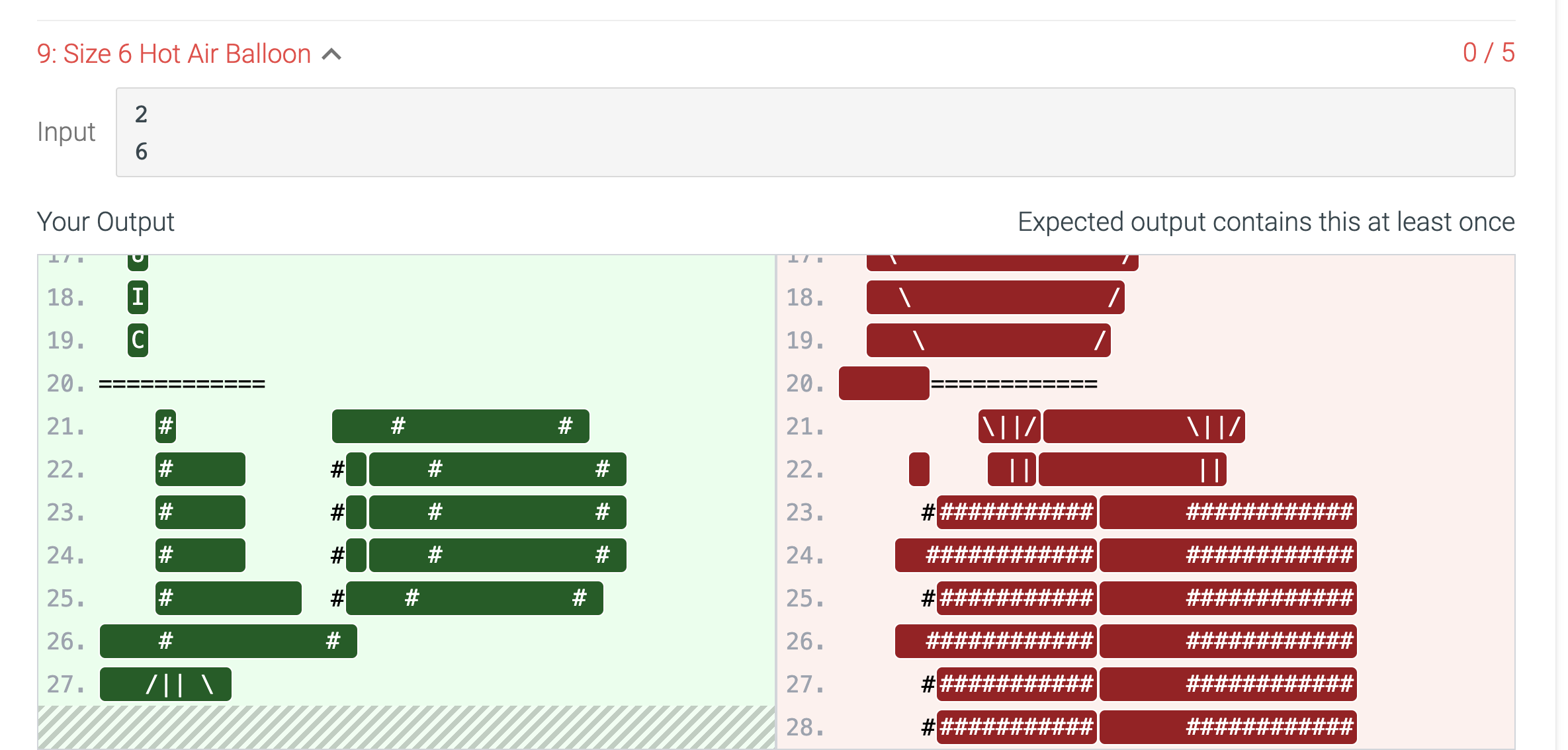
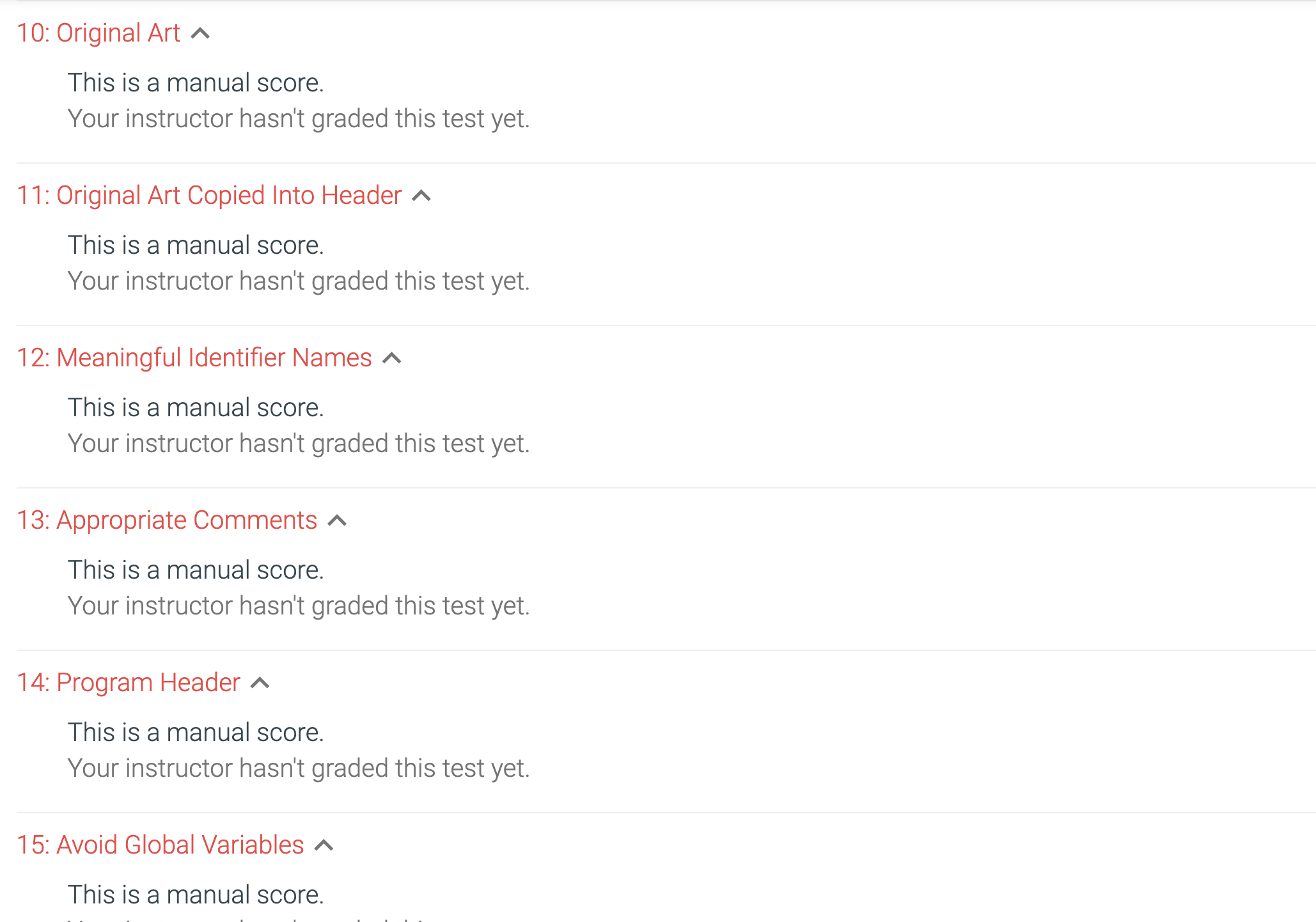
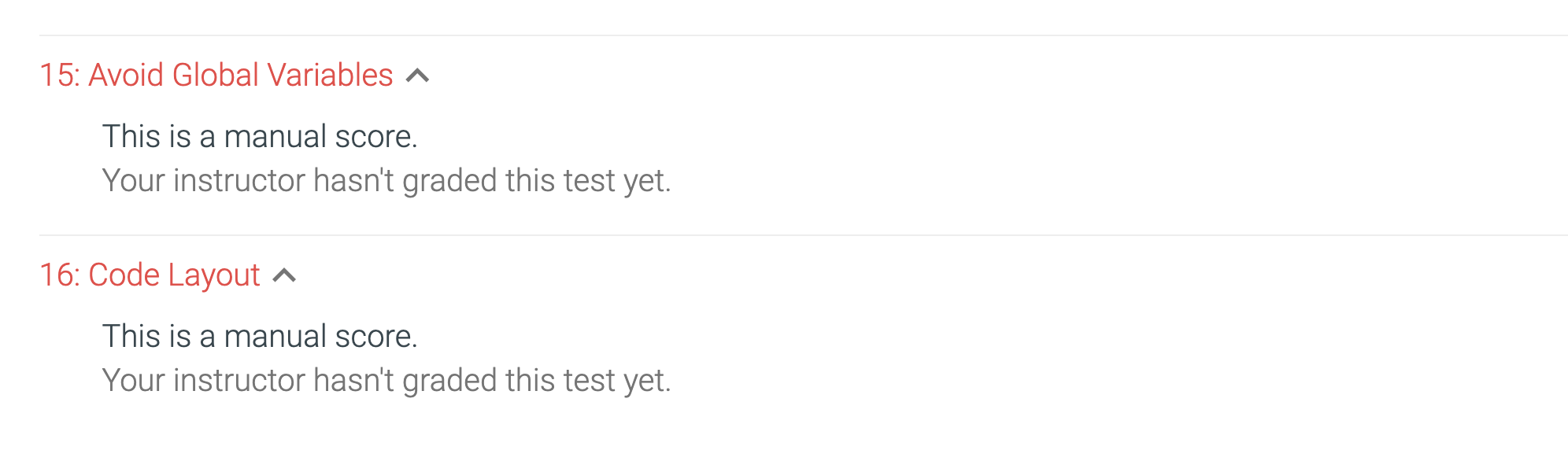
Main Menu
The main menu gives the user a choice of displaying your own art, or the hot air balloon.
If the user chooses your art, your program should display your own original ASCII art (requirements are listed below in the Original Art section).
If the user chooses the hot air balloon, your program should ask what the size of the hot air balloon should be, and then create the hot air balloon
Hot Air Balloon
The appearance of the balloon will be determined by the size the user enters. You may assume the user enters a number 2 or above (a size 1 balloon doesn't look very good).
For example, a size 2 balloon is shown below. Note that:
- The vertical sections are each 2 tall (since the size is 2). These are made using the characters: / | and \
- The top is 4 wide (2x size), and is made using the underscore character _
- The bottom of the balloon is 4 wide (2x size) and is made using the equals character =
- The basket at the bottom of the balloon is 2 tall (size) and 4 wide (2x size) and is made using the pound/hash symbol #
- the connection between the balloon and the basket is always 2 tall (even if size is bigger, this remains 2 tall) and uses the same characters as the vertical pieces: \ | and / It is centered below the balloon/above the basket.
____ / \ / \ | | | | \ / \ / ==== \||/ || #### ####
In order to pass most of the test cases, the balloon must match the above exactly, including spaces. There should be no spaces after the last character in the line.
UIC Text on Balloon
If the balloon is size 5 or more, it should have the text UIC, centered vertically and horizontally on the balloon (in the section with straight sides). If the balloon cannot be divided evenly in half, have the text go slightly up or slightly left. (So in the size 6 balloon shown below, there is one fewer line above the UIC text than below, and one fewer space on the left than on the right.)
____________ / \ / \ / \ / \ / \ / \ | | | U | | I | | C | | | | | \ / \ / \ / \ / \ / \ / ============ \||/ || ############ ############ ############ ############ ############ ############
Original Art
If the user chooses to display original art, a creation of your own design should be displayed. Your art should be very different from the pyramid in the previous step. If you want inspiration for your design, Google ASCII art. It should not require any input, so it can be evaluated more quickly when grading (if you'd like to make it depend on size, you can add input and then comment it out when you submit the program, making sure the size gets set to a value that works well).
Your original art must use at least one loop, and it must be between 5 and 80 lines of output, with a maximum width of 100 characters per line, including whitespace (spaces, tabs, etc).
To help with grading, copy and paste your finished art into the comments at the top of your program. Here is an example of my original art, although your original graphic should be different than the example given.
|| || ===++=== /=====\ || || || // \\ || || || || || || || || \\ // || \\ // \=====/ ===++=== \=====/
Formatting
Your program should be properly formatted, as described in this document. Content related to documenting functions and classes may be ignored, although you are welcome to use functions if you'd like (but they are not required).
Some Important C++ You'll Use
You can use setw and setfill to add spaces or other characters. setw creates a "width" for the text being displayed. So calling setw with 5, and then outputting "hi" will add 3 spaces before "hi" (so that there's a total of 5 being displayed).
cout << setw(5) << "hi" << endl;
If you have two pieces of text you want to use setw for, you need to call it before each output (after a piece of output, setw gets undone). For example: "hi" and "bye" will have widths of 5, but "yes" doesn't have any spaces added:
cout << setw(5) << "hi" << "yes" << setw(5) << "bye" << endl;
setfill can be used to change how setw behaves. Normally, setw will add spaces to get to the appropriate width, but you can call setfill to choose a different character. Notice that the period is in single quotes to tell C++ that it's a character (one letter/symbol) instead of a string (0 or more characters) which use double quotes.
cout << setfill('.') << setw(5) << "hi" << setw(5) << "bye" << endl;
Will display three periods instead of spaces, and then the word hi. Unlike setw, setfill persists--it will keep using periods until we tell it to use spaces again--so the word bye has two periods before it. This can be helpful for repeating a character, for example 10 dollar signs:
cout << setfill('$') << setw(10) << "$" << endl;
To display a back slash \ you'll actually need to write two in a row. That's because the backslash is a special character used to denote new lines (\n), tabs (\t), quotes (\"), etc. To specify that you actually want a backslash, you write it twice:
cout << "Output a backslash: \\" << endl;
Lastly, you'll need to use loops to complete the project. Here is an example of a while loop to display the numbers 1 to 10:
int counter = 1; while (counter <= 10) { cout << counter << endl; counter++; // Adds one to the counter variable }
Advice On Getting Started
After getting input for the size, create the top third of the balloon, without worrying about whitespace: create size lines of the top, with no spaces. For example, with size three:
/\ /\ /\
Now, add spaces so the left side is lined up the way it's supposed to be:
/\ /\ /\
Next, add spaces so the right side lines up as well. The might be a good time to add the top line also:
______ / \ / \ / \
You can do a similar process with the middle and bottom of the balloon, displaying the correct characters, and then adding spaces to line everything up. After that, add the basket and the lines that connect the balloon to the basket. I recommend starting by ignoring the UIC in the middle of larger balloons, that can be the last thing you add once the rest of your balloon is working well.
1: Small Hot Air Balloon ^ This test case's results were hidden by the instructor Feedback 2: Very Large Hot Air Balloon This test case's results were hidden by the instructor Feedback 3: Large Hot Air Balloon Input This test case's results were hidden by the instructor Feedback 4: Size 2 Hot Air Balloon (Ignores White Space) 2 2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
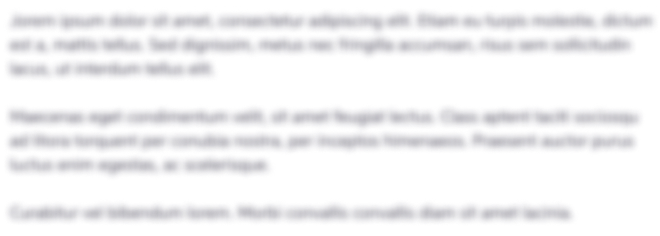
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started