Answered step by step
Verified Expert Solution
Question
1 Approved Answer
USE JAVA: Given the Interface Code and the Interface Implementation Code; Write Junit Tests to test all fuctionality. ------------- Interface code: public interface CustomList {
USE JAVA: Given the Interface Code and the Interface Implementation Code; Write Junit Tests to test all fuctionality.
-------------
Interface code:
public interface CustomList { /** * This method should add a new item into the CustomList and should * return true if it was successfully able to insert an item. * @param item the item to be added to the CustomList * @return true if item was successfully added, false if the item was not successfully added (note: it should always be able to add an item to the list) */ boolean add (T item); /** * This method should add a new item into the CustomList at the * specified index (thus shuffling the other items to the right). If the index doesn't * yet exist, then you should throw an IndexOutOfBoundsException. * @param index the spot in the zero-based array where you'd like to insert your * new item * @param item the item that will be inserted into the CustomList * @return true when the item is added * @throws IndexOutOfBoundsException */ boolean add (int index, T item) throws IndexOutOfBoundsException; /** * This method should return the size of the CustomList * based on the number of actual elements stored inside of the CustomList * @return an int representing the number of elements stored in the CustomList */ int getSize(); /** * This method will return the actual element from the CustomList based on the * index that is passed in. * @param index represents the position in the backing Object array that we want to access * @return The element that is stored inside of the CustomList at the given index * @throws IndexOutOfBoundsException */ T get(int index) throws IndexOutOfBoundsException; /** * This method should remove an item from the CustomList at the * specified index. This will NOT leave an empty null where the item * was removed, instead all other items to the right will be shuffled to the left. * @param index the index of the item to remove * @return the actual item that was removed from the list * @throws IndexOutOfBoundsException */ T remove(int index) throws IndexOutOfBoundsException;
-------------------------------------
interface implementation code:
import java.util.Arrays; // Updated this existing class to include the 7 changes stated below public class CustomArrayList implements CustomList { // 1st, created an instance variable called arraySize private int arraySize = 0; // 2nd, moved new Object[10] into the constructor as the constructor initializes // the object Object[] arrayObject; /** * add method contains the functionality of doubling the array in size when the * object array is full. EX: when adding the 11th element; object array grows * from 10 to 20 elements. EX: when adding the 21st element; object array grows * from 20 to 40 elements. */ // 3rd, Created the Constructor public CustomArrayList() { System.out.println("Constructor call creates an array object of 10 elements "); arrayObject = new Object[10]; // Default ArraySize is 10 elements } // ----------New functionality required as part of Hw7 -------------------- @Override public boolean add(int index, T item) throws IndexOutOfBoundsException { if (arraySize == arrayObject.length) { int invalidIndex = arrayObject.length + 1; System.out.println("Cannot add element at index position = " + invalidIndex); increaseSize(); return false; } else { arrayObject[arraySize++] = item; } return false; } // ----------Kept Old functionality from Hw5 -------------------- @Override public boolean add(T item) { //4th, Provided Method body for the CustomList Interface's add() method if(arraySize == arrayObject.length) { int invalidIndex=arrayObject.length+1; System.out.println("Cannot add element at index position = " + invalidIndex ); increaseSize(); return false; } else { arrayObject[arraySize++]= item; return true; } } @Override public T remove(int index) throws IndexOutOfBoundsException { // TODO Auto-generated method stub return null; } // --------------------Reusing Hw5's Code for everything listed below // ----------------- @Override public int getSize() { // 5th, Provided Method body for the CustomList Interface's getSize() method return arrayObject.length; } @SuppressWarnings("unchecked") @Override public T get(int index) { // 6th, Provided Method body for the CustomList Interface's get() method return (T) arrayObject[index]; } // 7th, Created a brand new method that exists outside of the CustomList Interface // in order to grow the array in size when it gets full private void increaseSize() { int updatedSize = (arrayObject.length) * 2; System.out.println( "Updating the Array Size to go from " + arrayObject.length + " to " + updatedSize + " elements"); System.out.println("Transferring all the elements into the bigger array "); arrayObject = Arrays.copyOf(arrayObject, updatedSize); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
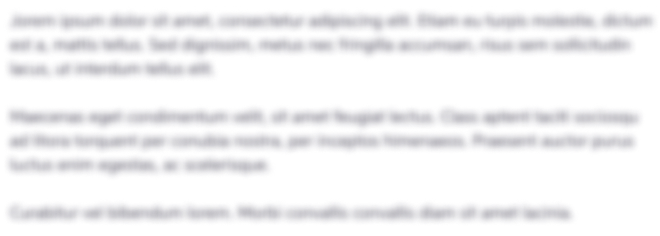
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started