Question
Perform the steps in exercise 4. Prepare a document with screenshots from your Linux terminal to showcase that the steps were successfully followed. Use 10
Perform the steps in exercise 4. Prepare a document with screenshots from your Linux terminal to showcase that the steps were successfully followed. Use 10 seconds instead of five seconds in the document.
Exercise 4:
I need Output SS of Linux Terminal of all steps that is Necessary
The scope of the fourth exercise is to understand the ROS architecture, nodes, and a communication among nodes using action. A client node will contact the server node and the server node will wait for a stipulated duration; then the client node will print the elapsed duration.
1: (First time only)
(2) Create the skeleton for ROS package
Following segments changes based on the home works and directories;
user@hostname$ cd ~/catkin_ws/src
(a project based on rospy package package dependency)
user@hostname$ catkin_create_pkg ex4 rospy
user@hostname$ ls
user@hostname$ cd ex4
user@hostname$ ls
(list and check the files within the directory)
(3) Changes to the package.xml
Edit the packages.xml in the directory ~/catkin_ws/src/ex4 as follows:
(4) Create an action file
user@hostname$ cd ~/catkin_ws/src/ex4
user@hostname$ mkdir action
Create a file Tim.action with the following contents:
duration time_to_wait
---
duration time_elapsed
uint32 updates_sent
---
duration time_elapsed
duration time_remaining
(4) Changes to CMakeLists.txt file
Edit the CMakeLists.txt in the directory ~/catkin_ws/src/ex2 as follows:
find_package(catkin REQUIRED COMPONENTS
# other packages are already listed here
actionlib_msgs
)
Uncomment and make the following change:
add_action_files(
DIRECTORY action
FILES Tim.action
)
## Generate added messages and services with any dependencies listed here
Uncomment the following:
generate_messages(
DEPENDENCIES
actionlib_msgs
std_msgs
)
Uncomment and add the following:
catkin_package(
# INCLUDE_DIRS include
# LIBRARIES ex4
CATKIN_DEPENDS
actionlib_msgs
)
(5) Create the directory for python files
(directory for creating python scripts, later we will create other directories as srv, msg)
user@hostname$ cd ~/catkin_ws/src/ex4
user@hostname$ mkdir scripts
user@hostname$ ls -al
you should see the scripts directory
(4) Create the Python file as a node representing client
Open an editor for Python (I use Geany ), and store the following file as fancy_action_client.py in the directory scripts. (make sure indentation are proper for Python programs when you copy/paste)
#! /usr/bin/env python
# BEGIN ALL
#! /usr/bin/env python
import rospy
import time
import actionlib
from ex4.msg import TimAction, TimGoal, TimResult, TimFeedback
# BEGIN PART_1
def feedback_cb(feedback):
print('[Feedback] Time elapsed: %f'%(feedback.time_elapsed.to_sec()))
print('[Feedback] Time remaining: %f'%(feedback.time_remaining.to_sec()))
# END PART_1
rospy.init_node('timer_action_client')
client = actionlib.SimpleActionClient('timer', TimAction)
client.wait_for_server()
goal = TimGoal()
goal.time_to_wait = rospy.Duration.from_sec(5.0)
# BEGIN PART_5
# Uncomment this line to test server-side abort:
#goal.time_to_wait = rospy.Duration.from_sec(500.0)
# END PART_5
# BEGIN PART_2
client.send_goal(goal, feedback_cb=feedback_cb)
# END PART_2
# BEGIN PART_4
# Uncomment these lines to test goal preemption:
#time.sleep(3.0)
#client.cancel_goal()
# END PART_4
client.wait_for_result()
# BEGIN PART_3
print('[Result] State: %d'%(client.get_state()))
print('[Result] Status: %s'%(client.get_goal_status_text()))
print('[Result] Time elapsed: %f'%(client.get_result().time_elapsed.to_sec()))
print('[Result] Updates sent: %d'%(client.get_result().updates_sent))
# END PART_3
# END ALL
The client file will be inside the folder ~/catkin_ws/src/ex4/scripts
user@hostname$ cd ~/catkin_ws/src/ex4/scripts
Make the script executable
user@hostname$ chmod +x fancy_action_client.py
(5) Create the Python file as a node representing server
Open an editor for Python, and store the following file as fancy_action_server.py in the directory scripts. (make sure indentation are proper for Python programs when you copy/paste)
#! /usr/bin/env python
# BEGIN ALL
#! /usr/bin/env python
import rospy
import time
import actionlib
# BEGIN PART_1
from ex4.msg import TimAction, TimGoal, TimResult, TimFeedback
# END PART_1
def do_timer(goal):
start_time = time.time()
# BEGIN PART_2
update_count = 0
# END PART_2
# BEGIN PART_3
if goal.time_to_wait.to_sec() > 60.0:
result = TimResult()
result.time_elapsed = rospy.Duration.from_sec(time.time() - start_time)
result.updates_sent = update_count
server.set_aborted(result, "Timer aborted due to too-long wait")
return
# END PART_3
# BEGIN PART_4
while (time.time() - start_time) < goal.time_to_wait.to_sec():
# END PART_4
# BEGIN PART_5
if server.is_preempt_requested():
result = TimResult()
result.time_elapsed = rospy.Duration.from_sec(time.time() - start_time)
result.updates_sent = update_count
server.set_preempted(result, "Timer preempted")
return
# END PART_5
# BEGIN PART_6
feedback = TimFeedback()
feedback.time_elapsed = rospy.Duration.from_sec(time.time() - start_time)
feedback.time_remaining = goal.time_to_wait - feedback.time_elapsed
server.publish_feedback(feedback)
update_count += 1
# END PART_6
# BEGIN PART_7
time.sleep(1.0)
# END PART_7
# BEGIN PART_8
result = TimResult()
result.time_elapsed = rospy.Duration.from_sec(time.time() - start_time)
result.updates_sent = update_count
server.set_succeeded(result, "Timer completed successfully")
# END PART_8
rospy.init_node('timer_action_server')
server = actionlib.SimpleActionServer('timer', TimAction, do_timer, False)
server.start()
rospy.spin()
# END ALL
(6) Compile and build the Nodes Python Files
Both fancy_action_client.py and fancy_action_server.py should be in the directory ~/catkin_ws/src/ex4/scripts
user@hostname$ cd ~/catkin_ws/src/ex4/scripts
user@hostname$ ls
user@hostname$ chmod +x fancy_action_server.py
Compile the packages so ROS can use it:
user@hostname$ cd ~/catkin_ws
user@hostname$ catkin_make
(7) Run the code
One terminal to run roscore (main ROS application): Open a terminal and run the following:
user@hostname$ roscore
Second terminal 2nd window to run the client node. If you set the setup.bash properly you can use the roscd to get to the package directory; then to the scripts ( cd into scripts) directory. Use the following command to start the client node.
user@hostname$ roscd ex4
user@hostname$ cd scripts
user@hostname$ rosrun ex4 fancy_action_client.py
Third window to run the server node.
user@hostname$ roscd ex4
user@hostname$ cd scripts
user@hostname$ rosrun ex4 fancy_action_server.py
Observe the outputs
Uncomment the following lines at fancy_action_client.py
# Uncomment these lines to test goal preemption:
#time.sleep(3.0)
#client.cancel_goal()
Uncomment the following lines at fancy_action_client.py
# Uncomment this line to test server-side abort:
#goal.time_to_wait = rospy.Duration.from_sec(500.0)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
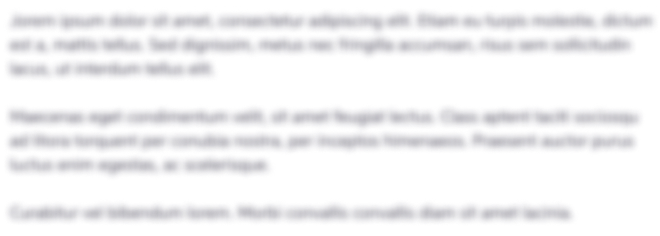
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started