USE PYTHON3; DO NOT IMPORT ANY PACKAGES
Please do both part 2 and part 3, I already finished part 1
Part 2:
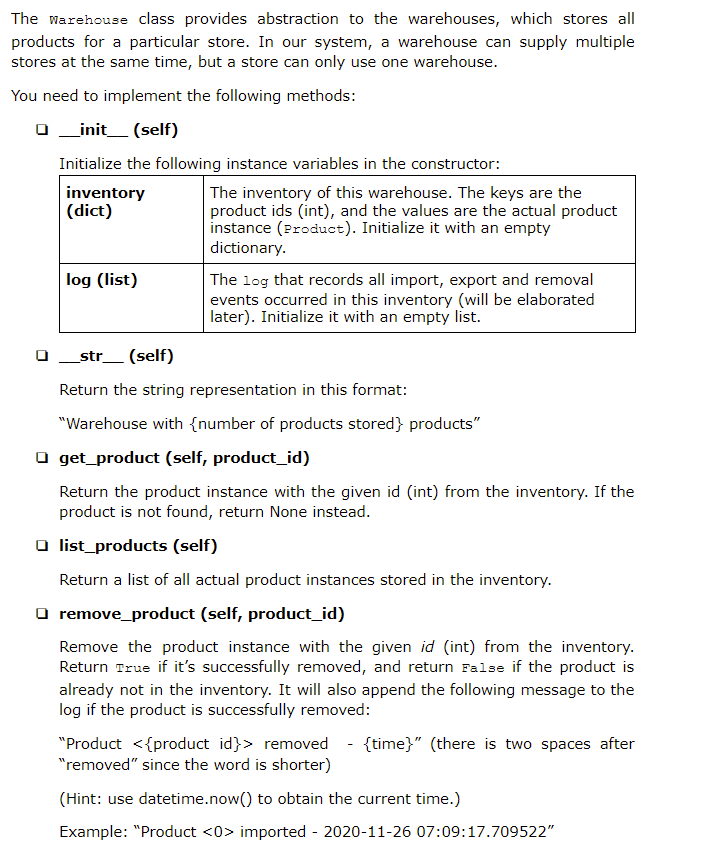
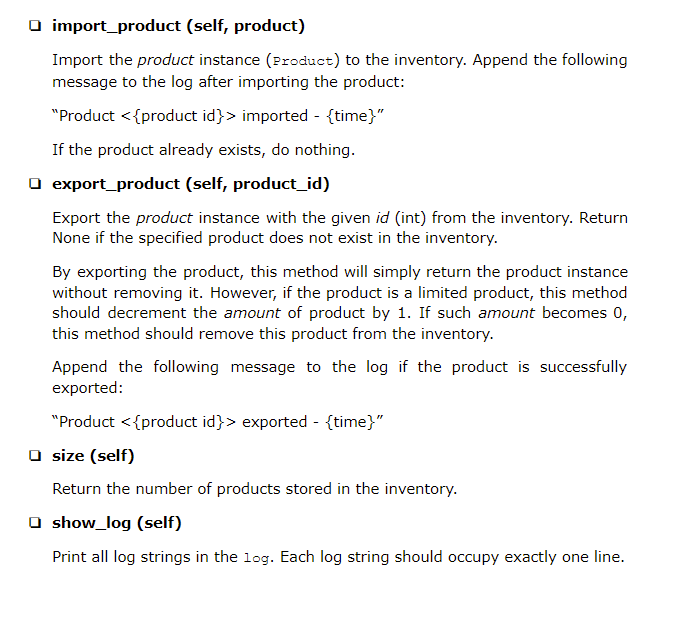
Part 3:
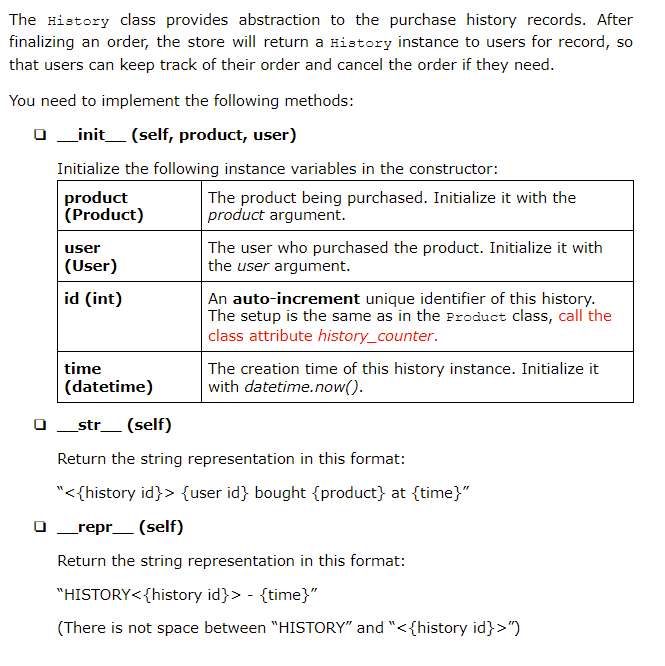
What the code looks like in the text editor:
class Warehouse: """ TODO: Complete the docstring. """
##### Part 2 ##### def __init__(self): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE # self.inventory = ... self.log = ...
def __str__(self): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
def get_product(self, product_id): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
def list_products(self): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
def remove_product(self, product_id): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
def import_product(self, product): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
def export_product(self, product_id): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
def size(self): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
def show_log(self): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
PART 3
class History: """ TODO: Complete the docstring. """
##### Part 3 ##### def __init__(self, product, user): """ TODO: Complete the docstring. """ self.product = ... self.user = ... self.id = ... self.time = datetime.now()
def __str__(self): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
def __repr__(self): """ TODO: Complete the docstring. """ # YOUR CODE GOES HERE #
Thank you so much!
Edit: Hi, I'm not sure what you mean. What's above is just the format of the code. As the problem is solved, you will be adding the code. If it helps, here's the link to part 1 of this problem:
https://www.chegg.com/homework-help/questions-and-answers/use-python3-import-packages-please-part-1-s-code-looks-like-editor-class-product-todo-comp-q69814934?trackid=VjkoWi59
Edit2: The link to part 1 is above and bolded. It has the question, the code format, and the code answer. Let me know if the link isn't working for you :)
The Warehouse class provides abstraction to the warehouses, which stores all products for a particular store. In our system, a warehouse can supply multiple stores at the same time, but a store can only use one warehouse. You need to implement the following methods: 0 __init__(self) Initialize the following instance variables in the constructor: inventory The inventory of this warehouse. The keys are the (dict) product ids (int), and the values are the actual product instance (Product). Initialize it with an empty dictionary. log (list) The log that records all import, export and removal events occurred in this inventory (will be elaborated later). Initialize it with an empty list. _str_ (self) Return the string representation in this format: "Warehouse with {number of products stored} products" O get_product (self, product_id) Return the product instance with the given id (int) from the inventory. If the product is not found, return None instead. o list_products (self) Return a list of all actual product instances stored in the inventory. O remove_product (self, product_id) Remove the product instance with the given id (int) from the inventory. Return True if it's successfully removed, and return False if the product is already not in the inventory. It will also append the following message to the log if the product is successfully removed: "Product removed {time}" (there is two spaces after "removed" since the word is shorter) (Hint: use datetime.now() to obtain the current time.) Example: "Product
imported - 2020-11-26 07:09:17.709522" O import_product (self, product) Import the product instance (Product) to the inventory. Append the following message to the log after importing the product: "Product imported - {time}" If the product already exists, do nothing. O export_product (self, product_id) Export the product instance with the given id (int) from the inventory. Return None if the specified product does not exist in the inventory. By exporting the product, this method will simply return the product instance without removing it. However, if the product is a limited product, this method should decrement the amount of product by 1. If such amount becomes 0, this method should remove this product from the inventory. Append the following message to the log if the product is successfully exported: "Product exported - {time}" size (self) Return the number of products stored in the inventory. O show_log (self) Print all log strings in the log. Each log string should occupy exactly one line. The History class provides abstraction to the purchase history records. After finalizing an order, the store will return a History instance to users for record, so that users can keep track of their order and cancel the order if they need. You need to implement the following methods: _init__(self, product, user) Initialize the following instance variables in the constructor: product The product being purchased. Initialize it with the (Product) product argument. The user who purchased the product. Initialize it with (User) the user argument. id (int) An auto-increment unique identifier of this history. The setup is the same as in the Product class, call the class attribute history_counter. time The creation time of this history instance. Initialize it (datetime) with datetime.now(). user _str_ (self) Return the string representation in this format: "{user id} bought {product} at {time}" repr_(self) Return the string representation in this format: "HISTORY - {time}" (There is not space between "HISTORY" and "") The Warehouse class provides abstraction to the warehouses, which stores all products for a particular store. In our system, a warehouse can supply multiple stores at the same time, but a store can only use one warehouse. You need to implement the following methods: 0 __init__(self) Initialize the following instance variables in the constructor: inventory The inventory of this warehouse. The keys are the (dict) product ids (int), and the values are the actual product instance (Product). Initialize it with an empty dictionary. log (list) The log that records all import, export and removal events occurred in this inventory (will be elaborated later). Initialize it with an empty list. _str_ (self) Return the string representation in this format: "Warehouse with {number of products stored} products" O get_product (self, product_id) Return the product instance with the given id (int) from the inventory. If the product is not found, return None instead. o list_products (self) Return a list of all actual product instances stored in the inventory. O remove_product (self, product_id) Remove the product instance with the given id (int) from the inventory. Return True if it's successfully removed, and return False if the product is already not in the inventory. It will also append the following message to the log if the product is successfully removed: "Product removed {time}" (there is two spaces after "removed" since the word is shorter) (Hint: use datetime.now() to obtain the current time.) Example: "Product imported - 2020-11-26 07:09:17.709522" O import_product (self, product) Import the product instance (Product) to the inventory. Append the following message to the log after importing the product: "Product imported - {time}" If the product already exists, do nothing. O export_product (self, product_id) Export the product instance with the given id (int) from the inventory. Return None if the specified product does not exist in the inventory. By exporting the product, this method will simply return the product instance without removing it. However, if the product is a limited product, this method should decrement the amount of product by 1. If such amount becomes 0, this method should remove this product from the inventory. Append the following message to the log if the product is successfully exported: "Product exported - {time}" size (self) Return the number of products stored in the inventory. O show_log (self) Print all log strings in the log. Each log string should occupy exactly one line. The History class provides abstraction to the purchase history records. After finalizing an order, the store will return a History instance to users for record, so that users can keep track of their order and cancel the order if they need. You need to implement the following methods: _init__(self, product, user) Initialize the following instance variables in the constructor: product The product being purchased. Initialize it with the (Product) product argument. The user who purchased the product. Initialize it with (User) the user argument. id (int) An auto-increment unique identifier of this history. The setup is the same as in the Product class, call the class attribute history_counter. time The creation time of this history instance. Initialize it (datetime) with datetime.now(). user _str_ (self) Return the string representation in this format: "{user id} bought {product} at {time}" repr_(self) Return the string representation in this format: "HISTORY - {time}" (There is not space between "HISTORY" and "")